
#include<iostream>
using namespace std;
#define MaxSize 10
typedef int ElemType;
struct Stack {
ElemType data[MaxSize];
int top;
} SqStack;
void init(Stack &s) {
s.top = -1;
}
bool push(Stack &s, ElemType x)
{
if (s.top == MaxSize - 1) {
return false;
}
s.top = s.top + 1;
s.data[s.top] = x;
return true;
}
bool pop(Stack &s, ElemType &x) {
if(s.top == -1) {
return false;
}
x = s.data[s.top];
s.top = s.top - 1;
return true;
}
void traverse(Stack s) {
for (int i = 0; i <= s.top; i++) {
cout << s.data[i] << " ";
}
cout << endl;
}
int length(Stack s) {
return s.top + 1;
}
int main() {
Stack s;
init(s);
ElemType x;
push(s, 1);
push(s, 2);
push(s, 3);
push(s, 4);
push(s, 5);
traverse(s);
pop(s, x);
cout << "pop: " << x << endl;
traverse(s);
cout << "length: " << length(s) << endl;
return 0;
}
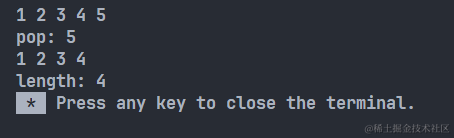