My_string.cpp
#include <iostream>
#include "my_string.h"
#include <string.h>
using namespace std;
My_string::My_string():size(15)
{
this->ptr = new char[size] ;
this->ptr[0]='\0';//串为空串
this->len = 0;
};
My_string::My_string(const My_string&other)
{
this->len = other.len;
this->size = other.size;
this->ptr = new char[this->size];
strcpy(this->ptr,other.ptr);
cout<<"调用拷贝构造函数 "<<endl;
}
My_string::My_string(const char *src):size(15)
{
this->ptr = new char[size] ;
this->len = 0;
char *temp =this->ptr;
while(*src!='\0')
{
*temp = *src;
temp++;
src++;
this->len++;
}
*temp = '\0';
//cout<<"调用构造函数 "<<endl;
}
My_string & My_string:: operator=(const My_string&other)
{
if(this!=&other)
{
this->len = other.len;
this->size = other.size;
this->ptr = new char[this->size];
strcpy(this->ptr,other.ptr);
}
cout<<"调用拷贝赋值函数 "<<endl;
return *this;
}
My_string::My_string(int num ,char value):size(15)
{
if(num<0)
{
num= 0;
}else if(num>this->size-1){
num = this->size-1;
}
this->len = 0;
int i = 0;
this->ptr = new char[size] ;
char *temp =this->ptr;
while(i<num)
{
*(temp+i) = value;
i++;
this->len++;
}
}
My_string::~My_string()
{
delete []ptr;
}
void My_string::push_pop()
{
if(empty())
{
return ;
}
this->ptr[this->len-1]= '\0';
this->len--;
}
void My_string::clear()
{
while(*ptr!='\0')
{
push_pop();
}
}
char* My_string::data(){
return this->ptr;
}
bool My_string::empty()
{
return this->ptr==NULL;
}
void My_string::push_back(char value)
{
this->ptr[this->len] = value;
this->len++;
}
char &My_string::at(int index)
{
if(index<0||index>this->size-1||empty())
{
}
return this->ptr[index-1];
}
int My_string::get_length()
{
return this->len;
}
int My_string::get_size()
{
return this->size;
}
void My_string::junzi()
{
char *temp= new char[this->size*2] ;
if(this->size-1<=this->len)
{
strcpy(temp,this->ptr);
delete [] this->ptr;//释放原来的指针
this->ptr = temp;
this->size *=2;
}
}
My_string.h
#ifndef MY_STRING_H
#define MY_STRING_H
class My_string
{
private:
char *ptr;
int size;
int len;
public:
//无参构造
My_string();
//有参构造
My_string(const char *src);
//5,A
My_string(int num ,char value);
//拷贝构造
My_string(const My_string&other);
//拷贝赋值
My_string & operator=(const My_string&other);
//析构函数
~My_string();
//判空
bool empty();
//尾插
void push_back(char value);
//尾删
void push_pop();
//at函数实现
char &at(int index);
//清空函数
void clear();
//返回c风格字符串
char *data();
//返回实际长度
int get_length();
//返回当前最大容量
int get_size();
//君子函数:容器满时,二倍扩容
void junzi();
};
#endif // MY_STRING_H
main.cpp
#include <iostream>
#include "my_string.h"
using namespace std;
int main()
{
//string s4("AAAAAc") ;
//s4.length();
//cout<<"s1.length"<<s4.length()<<endl;
My_string s0;
My_string s1("hello");
s1.clear();
cout<<"s1. ptr "<<s1.data()<<endl;
cout<<"******************"<<endl;
My_string s2(5,'A');
cout<<"s2. ptr "<<s2.data()<<endl;
s2.push_back('c');
s2.push_back('h');
s2.push_back('i');
s2.push_pop();
s2.push_pop();
cout<<"s2. ptr "<<s2.data()<<endl;
char c =s2.at(6);
int len = s2.get_length();
cout<<"char is "<<c<<endl;
cout<<"len is "<<len<<endl;
int n = s2.get_size();
cout<<"n is "<<n<<endl;
cout<<"******************"<<endl;
My_string s5("world");
My_string s6=s5;
My_string s7;
s7.operator=(s5);
cout<<"s6.ptr is "<<s6.data()<<endl;
cout<<"s7.ptr is "<<s7.data()<<endl;
cout<<"******************"<<endl;
My_string s8 ="12321321321321";
s8.junzi();
cout<<"s8.size = "<<s8.get_size()<<endl;
return 0;
}
思维导图
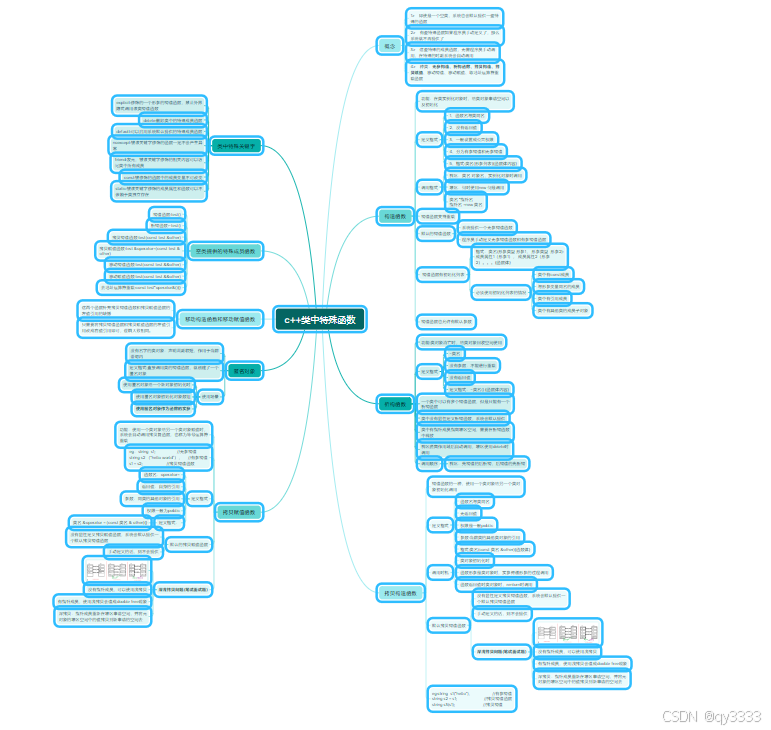