C++: String容器的使用和实现
前言:
1、首先要了解一下STL,什么是STL?如果把数据结构的章节比喻成造车,那么STL则是开车去各种地方,站在前人的肩膀上去看世界,进行更高效的工作!
2、首先我们先来介绍第一种容器,string,在以后学习新知识中,我们都需要具备一定阅读文档的能力,比如说cplusplus这个网站。接下来我们按照这个网站中对于string类介绍的内容逐渐认识string类。
一、STL
1、什么是STL
STL(standard template libaray-标准模板库):是C++标准库的重要组成部分,不仅是一个可复用的组件库,而且是一个包罗数据结构与算法的软件框架。
2、STL的六大组件
3、如何去学习STL
网上有句话说:“不懂STL,不要说你会C++”。STL是C++中的优秀作品,有了它的陪伴,许多底层的数据结构以及算法都不需要自己重新造轮子,直接站在前人的肩膀上,健步如飞的快速开发。
简单总结一下:学习STL的三个境界:能用,明理,能扩展。
二、string的使用
1、C语言中的字符串
C语言中,字符串是以'\0'结尾的一些字符的集合,为了操作方便,C标准库中提供了一些str系列的库函数,但是这些库函数与字符串是分离开的,不太符合OOP的思想,而且底层空间需要用户自己管理,稍不留神可能还会越界访问。
2、标准库中的string类
https://cplusplus.com/reference/string/string/?kw=string 大家可以点击cplusplus网站自行查阅string类的全部接口,我们接下来讲解它的常用接口。
3、string类常用接口
3.1.string类的构造
#include<iostream>
#include<string>
using namespace std;
int main()
{
string s1;//std::
string s2("hello");
string s3(10, 'a');
string s4(s2);//拷贝构造
string s5 = "hello";//构造+拷贝构造+优化
string s6 = s2;//拷贝构造
s1 = s2;
cout << s1 << endl;//operator
cout << s2 << endl;
cout << s3 << endl;
cout << s4 << endl;
}
代码中的s1到s4都是按照上面表格中的函数名称顺序来进行编写。
s1并非是一个空类,上文讲解到字符串是以'\0'结尾的,它至少会包含一个‘\0',比如一个字符串中存了10个字符,但是它的容量为11,最后一位为'\0'。
如下是标准库里给的标准参考:
// string constructor
#include <iostream>
#include <string>
int main ()
{
std::string s0 ("Initial string");
// constructors used in the same order as described above:
std::string s1;
std::string s2 (s0);
std::string s3 (s0, 8, 3);
std::string s4 ("A character sequence");
std::string s5 ("Another character sequence", 12);
std::string s6a (10, 'x');
std::string s6b (10, 42); // 42 is the ASCII code for '*'
std::string s7 (s0.begin(), s0.begin()+7);
std::cout << "s1: " << s1 << "\ns2: " << s2 << "\ns3: " << s3;
std::cout << "\ns4: " << s4 << "\ns5: " << s5 << "\ns6a: " << s6a;
std::cout << "\ns6b: " << s6b << "\ns7: " << s7 << '\n';
return 0;
}
3.2.string类对象修改操作(增删查改)
void test_string1()
{
//string s;
//s.push_back('x');
//s.append("111111111");
//s += 'x';
//s += "xxxxxxxxx";//可读性更强
//cout << s << endl;
string s;
s += '1';//operator+=
s += "3456";
cout << s << endl;
s.insert(s.begin(), '0');
cout << s << endl;
s.insert(2, "2");
cout << s << endl;
s.erase(2, 10);
cout << s << endl;
}
3.3.string类的迭代器
迭代器,可以用它来遍历这个字符串,或者修改它。有正向迭代器,和反向迭代器,顾名思义,就是正着遍历和反着遍历。但是如果是const迭代器,是不能修改的。
// 遍历
void test_string2()
{
string s1("hello");
s1 += ' ';
s1 += "world";
cout << s1 << endl;
//[下标] //常用
for (size_t i = 0; i < s1.size(); ++i)
{
//写
s1[i] += 1;
}
for (size_t i = 0; i < s1.size(); ++i)
{
//读
cout << s1[i] << " ";
}
cout << endl;
//迭代器
//写
string::iterator it = s1.begin();
//auto it = s1.begin();
while (it != s1.end())
{
*it -= 1;
++it;
}
//读
it = s1.begin();
while (it != s1.end())
{
cout << *it << " ";
++it;
}
cout << endl;
//范围for//原理:被替换成迭代器
for (auto ch : s1)
{
cout << ch << " ";
}
}
int string2int(const string& str)//传参,拷贝+引用
{
int val = 0;
//const 迭代器 只能读不能写
string::const_iterator it = str.begin();//const_,,,与传参那里的const相对应
while (it != str.end())
{
//*it = 'a';
val *= 10;
val += (*it - '0');//将12345转成 一万两千三百四十五
++it;
}
val = 0;
string::const_reverse_iterator rit = str.rbegin();
//auto rit = str.rbegin();
while (rit != str.rend())
{
//*it = 'a';
val *= 10;
val += (*rit - '0');
++rit;
}
return val;
}
//3.其他迭代器
void test_string3()
{
//倒着遍历
string s1("hello world");
string::reverse_iterator rit = s1.rbegin();
while (rit != s1.rend())
{
cout << *rit << " ";//倒着打印hello world
++rit;
}
cout << endl;
string nums("12345");
cout << string2int(nums) << endl;
}
//方向:正向迭代器,反向迭代器
//属性:普通和const
3.4.string类对象的容量操作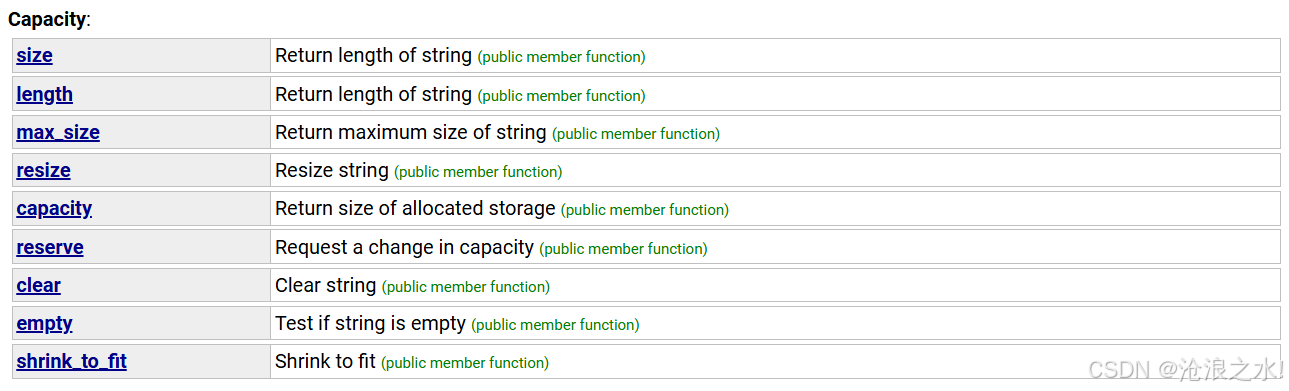
void test_string4()
{
string s1("hello world");
string s2("hello");
cout << "s1.size() :"<<s1.size() << endl;
cout << "s2.size() :"<<s2.size() << endl;
cout << "s1.length():"<<s1.length() << endl;
cout << "s2.length():"<<s2.length() << endl;//size == length
cout << "s1.capacity():"<<s1.capacity() << endl;
cout << "s2.capacity():"<<s2.capacity() << endl;
s1 += "1111111";
cout << "+=之后的s1:" << endl;
cout << "s1.capacity():"<<s1.capacity() << endl;//按倍数增容
s1.clear();
cout << "clear()之后的s1:" << endl;
cout << s1 << endl;
cout << s1.capacity() << endl;//空间还在
}
首先来看一下它们各自的长度与空间大小:
void test_string5()
{
//string s;
s.reserve(100);//不增容了,给了100空间
//s.resize(100,'x');//size也到100,字符的个数,
//size_t sz = s.capacity();
//cout << "making s grow:" << endl;
//for (int i = 0; i < 100; ++i)
//{
// s.push_back('c');
// if (sz != s.capacity())
// {
// sz = s.capacity();//将近1.5倍增容,
// cout << "capacity changed:" << sz << endl;
// }
//}
string s("hello world");
s.resize(5);
s.resize(20, 'x');//空间不够,会增容。helloxxxxxxx......
}
自此,string的常见使用都已经展示完了,接下来我们来实现一下它底层是怎么操作的。
三、string的实现
我们首先先提一下拷贝:之前章节上有讲,浅拷贝和深拷贝,浅拷贝就是只是拷贝了它的值,但是空间还是共用同一块空间,当出现析构,或者是清理情况下,就会出现错误。深拷贝就是创建了一块自己的空间,并且把值也copy过来了,有了自己的空间和相同的值。
**浅拷贝**:只是简单地复制对象的指针或引用,而不复制实际的数据。这样,两个对象将共享同一块内存数据。如果其中一个对象修改了数据,另一个对象也会看到这些更改。
**深拷贝**:不仅复制对象的指针或引用,还复制它所指向的数据。这样,每个对象都有自己独立的数据副本,互不影响。
在C++中,`std::string` 类的拷贝(无论是通过复制构造函数还是赋值操作符)都是执行深拷贝的。这意味着当你创建一个 `std::string` 对象的副本时,新的对象会拥有自己独立的数据副本,而不是仅仅指向原始对象的数据。
为了不和头文件中的string类起冲突,自己创建一个namepace,接下来就是实现的所有代码:
#pragma once
#include<iostream>
#include<string.h>
#include<assert.h>
using namespace std;
namespace zzx
{
class string
{
public:
string()
{
_str = new char[1];
_str[0] = '\0';
_size = _capacity = 0;
}
string(const char* str = "")//构造初始化
{
_size = strlen(str);
_capacity = _size;
_str = new char[_capacity + 1];
}
//s2(s1)//传统写法
string(const string& s)//拷贝构造,深拷贝,传统写法
{
_str = new char[s._capacity + 1];
_capacity = s._capacity;
_size = s._size;
strcpy(_str, s._str);
}
//s1 = s2
string& operator=(const string& s)
{
if (this != &s)
{
char* tmp = new char[s._capacity + 1];
strcpy(_str, s._str);
delete[] _str;
_str = tmp;
_size = s._size;
_capacity = s._capacity;
}
return *this;
}
void swap(string& s)
{
std::swap(_str, s._str);
std::swap(_size, s._size);
std::swap(_capacity, s._capacity);
}
//深拷贝现代写法
string(const string& s)
:_str(nullptr)
, _size(0)
, _capacity(0)
{
string tmp(s._str);//构造
swap(tmp);
}
string& operator=(const string& s)
{
if (this != &s)
{
string tmp(s);
swap(tmp);
}
return *this;
}
string& operator=(string s)
{
swap(s);
return *this;
}
~string()
{
delete[] _str;
_str = nullptr;
_size = _capacity = 0;
}
typedef char* iterator;
iterator begin()
{
return _str;
}
iterator end()
{
return _str + _size;
}
const char* c_str() const
{
return _str;
}
size_t size()const
{
return _size;
}
size_t capacity()const
{
return _capacity;
}
char& operator[](size_t pos) //普通对象可读可写
{
assert(pos < _size);
return _str[pos];
}
const char& operator[](size_t pos) const//只读
{
assert(pos < _size);
return _str[pos];
}
void reserve(size_t n)
{
if (n > _capacity)
{
char* tmp = new char[n + 1];
strcpy(tmp, _str);
delete[] _str;
_str = tmp;
_capacity = n;
}
}
void resize(size_t n, char ch = '\0')
{
if (n > _size)
{
reserve(n);
for (size_t i = _size; _size < n; ++i)
{
_str[i] = ch;
}
_size = n;
_str[_size] = '\0';
}
else
{
_size = n;
_str[_size] = '\0';
}
}
void push_back(char ch)
{
if (_size == _capacity)
{
size_t newCapacity = _capacity = 0 ? 4 : _capacity * 2;
reserve(newCapacity);
}
_str[_size] = ch;
++_size;
_str[_size] = '\0';
}
void append(const char* str)
{
size_t len = strlen(str);
if (_size + len > _capacity)
{
reserve(_size + len);
}
strcpy(_str + _size, str);
_size += len;
}
string& operator+=(char ch)
{
push_back(ch);
return *this;
}
string& operator+=(const char* str)
{
append(str);
return *this;
}
string& insert(size_t pos, char ch)
{
assert(pos <= _size);
if (_size == _capacity)
{
size_t newCapacity = _capacity = 0 ? 4 : _capacity * 2;
reserve(newCapacity);
}
size_t end = _size + 1;
while (end > pos)
{
_str[end] = _str[end - 1];
--end;
}
_str[pos] = ch;
++_size;
return *this;
}
string& insert(size_t pos, const char* str)
{
size_t len = strlen(str);
if (_size + len > _capacity)
{
reserve(_size + len);
}
size_t end = _size + len;
while (end > pos + len - 1)
{
_str[end] = _str[end - len];
--end;
}
strncpy(_str + pos, str, len);
_size += len;
return *this;
}
string& erase(size_t pos, size_t len = npos)
{
assert(pos < _size);
if (len == npos || pos + len > _size)
{
_str[pos] = '\0';
_size -= len;
}
else
{
strcpy(_str + pos, _str + pos + len);
_size -= len;
}
return *this;
}
size_t find(char ch, size_t pos = 0)const
{
assert(pos < _size);
while (pos < _size)
{
if (_str[pos] == ch)
{
return pos;
}
++pos;
}
return npos;
}
size_t find(const char* str, size_t pos = 0) const
{
assert(pos < _size);
const char* ptr = strstr(_str + pos, str);
if (ptr == nullptr)
{
return npos;
}
else
{
return ptr - _str;
}
}
void clear()
{
_size = 0;
_str[0] = '\0';
}
private:
char* _str;
size_t _size;
size_t _capacity;
const static size_t npos = -1;
};
}
ostream& operator<<(ostream& out, const string& s)
{
for (size_t i = 0; i < s.size(); ++i)
{
out << s[i];
}
return out;
}
istream& operator>>(istream& in, string& s)
{
s.clear();
char buff[127] = { '\0' };
size_t i = 0;
char ch = in.get();
while (ch != ' ' && ch != '\0')
{
if (i == 127)
{
s += buff;
i = 0;
}
buff[i++] = ch;
ch = in.get();
}
if (i > 0)
{
buff[i] = '\0';
s += buff;
}
return in;
}
如果不正之处,或者大家对于哪块知识还有疑惑,欢迎大家评论或者私信,我将会与大家一起学习共同探讨!!!