1、完整代码自己高德申请key,其他文章有写的
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title></title>
<script src="https://webapi.amap.com/maps?v=1.4.15&key="></script>
<style>
#map {
width: 100%;
height: 800px;
}
</style>
</head>
<body>
<h1>通过经纬度查找位置和轨迹回放</h1>
<label for="longitude">经度:</label>
<input type="text" id="longitude" placeholder="请输入经度">
<label for="latitude">纬度:</label>
<input type="text" id="latitude" placeholder="请输入纬度">
<button id="getAddress">查找位置</button>
<button id="startReplay">开始轨迹回放</button>
<button id="pauseReplay">暂停回放</button>
<button id="resumeReplay">继续回放</button>
<button id="stopReplay">停止回放</button>
<div id="output"></div>
<div id="map"></div>
<script>
const map = new AMap.Map('map', {
zoom: 13,
center: [108.999, 34.2449]
});
let currentMarker = null;
let replayMarker = null;
// 定义轨迹数据
const pathData = [
[108.999, 34.2449],
[109.000, 34.2452],
[109.002, 34.2460],
[109.004, 34.2475],
[109.005, 34.2480],
[109.006, 34.2485],
[109.008, 34.2490],
[109.010, 34.2505],
[109.012, 34.2520],
[109.014, 34.2535],
[109.016, 34.2550],
[109.018, 34.2560],
[109.020, 34.2570],
[109.022, 34.2580],
[109.024, 34.2590],
[109.026, 34.2600],
[109.028, 34.2615],
[109.030, 34.2630],
[109.032, 34.2645],
[109.034, 34.2660],
[109.036, 34.2670],
[109.038, 34.2680],
[109.040, 34.2690],
[109.042, 34.2700],
[109.044, 34.2715],
[109.046, 34.2730],
[109.048, 34.2745],
[109.050, 34.2760],
[109.052, 34.2770],
[109.054, 34.2780]
];
//绘制轨迹线
const polyline = new AMap.Polyline({
path: pathData,
strokeColor: "#FF0000",
strokeWeight: 4,
map: map
});
document.getElementById('getAddress').addEventListener('click', function() {
const longitude = document.getElementById('longitude').value;
const latitude = document.getElementById('latitude').value;
if (!longitude || !latitude) {
document.getElementById('output').innerText = '请输入有效的经纬度。';
return;
}
const key = '';
const location = `${longitude},${latitude}`;
const apiUrl = `https://restapi.amap.com/v3/geocode/regeo?key=${key}&location=${location}&extensions=all&output=JSON&radius=10`;
fetch(apiUrl)
.then(response => response.json())
.then(data => {
if (data.status === '1' && data.regeocode) {
const address = data.regeocode.formatted_address;
document.getElementById('output').innerText = `具体位置: ${address}`;
const lngLat = new AMap.LngLat(longitude, latitude);
if (currentMarker) {
map.remove(currentMarker);
}
currentMarker = new AMap.Marker({
position: lngLat,
title: address
});
currentMarker.setMap(map);
map.setCenter(lngLat);
map.setZoom(15);
} else {
document.getElementById('output').innerText = '获取具体位置失败。错误信息: ' + data.info;
}
})
.catch(error => {
console.error('错误:', error);
document.getElementById('output').innerText = '请求出错,请重试。';
});
});
// 轨迹回放控制
document.getElementById('startReplay').addEventListener('click', function() {
if (replayMarker) {
map.remove(replayMarker);
}
// 创建一个轨迹点
replayMarker = new AMap.Marker({
position: pathData[0],//初始轨迹点
// icon: "https://webapi.amap.com/images/car.png",
map: map,
autoRotation: true//标记点在路径移动过程中自动旋转方向,始终保持面向前进方向
});
replayMarker.moveAlong(pathData, 100); // 移动速度控制
});
document.getElementById('pauseReplay').addEventListener('click', function() {
if (replayMarker) {
replayMarker.pauseMove();
}
});
document.getElementById('resumeReplay').addEventListener('click', function() {
if (replayMarker) {
replayMarker.resumeMove();
}
});
document.getElementById('stopReplay').addEventListener('click', function() {
if (replayMarker) {
replayMarker.stopMove();
}
});
</script>
</body>
</html>
2、效果图 可以根据横纵坐标查询位置
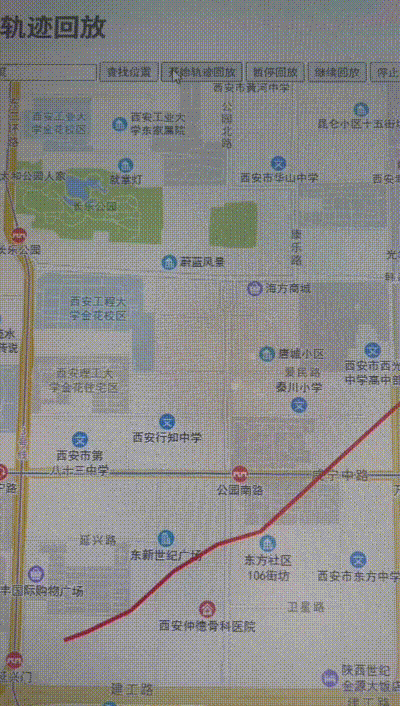