1 引入依赖
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://maven.apache.org/POM/4.0.0"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.xu</groupId>
<artifactId>KotlinOpenCV</artifactId>
<version>1.0</version>
<properties>
<kotlin.version>2.0.0</kotlin.version>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<kotlin.code.style>official</kotlin.code.style>
<kotlin.compiler.jvmTarget>1.8</kotlin.compiler.jvmTarget>
</properties>
<repositories>
<repository>
<id>mavenCentral</id>
<url>https://repo1.maven.org/maven2/</url>
</repository>
</repositories>
<build>
<sourceDirectory>src/main/kotlin</sourceDirectory>
<testSourceDirectory>src/test/kotlin</testSourceDirectory>
<plugins>
<plugin>
<groupId>org.jetbrains.kotlin</groupId>
<artifactId>kotlin-maven-plugin</artifactId>
<version>2.0.0</version>
<executions>
<execution>
<id>compile</id>
<phase>compile</phase>
<goals>
<goal>compile</goal>
</goals>
</execution>
<execution>
<id>test-compile</id>
<phase>test-compile</phase>
<goals>
<goal>test-compile</goal>
</goals>
</execution>
</executions>
</plugin>
<plugin>
<artifactId>maven-surefire-plugin</artifactId>
<version>2.22.2</version>
</plugin>
<plugin>
<artifactId>maven-failsafe-plugin</artifactId>
<version>2.22.2</version>
</plugin>
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>exec-maven-plugin</artifactId>
<version>1.6.0</version>
<configuration>
<mainClass>MainKt</mainClass>
</configuration>
</plugin>
</plugins>
</build>
<dependencies>
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-all</artifactId>
<version>5.8.29</version>
</dependency>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-compress</artifactId>
<version>1.27.0</version>
</dependency>
<dependency>
<groupId>org.tukaani</groupId>
<artifactId>xz</artifactId>
<version>1.10</version>
</dependency>
<dependency>
<groupId>org.jetbrains.kotlinx</groupId>
<artifactId>kotlinx-coroutines-core</artifactId>
<version>1.9.0-RC</version>
</dependency>
<dependency>
<groupId>org.bytedeco</groupId>
<artifactId>opencv-platform</artifactId>
<version>4.9.0-1.5.10</version>
</dependency>
<dependency>
<groupId>org.bytedeco</groupId>
<artifactId>ffmpeg-platform</artifactId>
<version>6.1.1-1.5.10</version>
</dependency>
<dependency>
<groupId>org.jetbrains.kotlin</groupId>
<artifactId>kotlin-test-junit5</artifactId>
<version>2.0.0</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter</artifactId>
<version>5.10.0</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.jetbrains.kotlin</groupId>
<artifactId>kotlin-stdlib</artifactId>
<version>2.0.0</version>
</dependency>
</dependencies>
</project>
2 测试代码
package com.xu.com.xu.image
import org.bytedeco.javacpp.Pointer
import org.bytedeco.opencv.global.opencv_core
import org.bytedeco.opencv.global.opencv_highgui
import org.bytedeco.opencv.global.opencv_imgproc
import org.bytedeco.opencv.opencv_core.Mat
import org.bytedeco.opencv.opencv_core.Point
import org.bytedeco.opencv.opencv_core.Scalar
import org.bytedeco.opencv.opencv_highgui.MouseCallback
import org.opencv.core.Core
import java.io.File
import java.util.*
object Drawing {
init {
val os = System.getProperty("os.name")
val type = System.getProperty("sun.arch.data.model")
if (os.uppercase(Locale.getDefault()).contains("WINDOWS")) {
val lib = if (type.endsWith("64")) {
File("lib\\opencv-4.9\\x64\\" + System.mapLibraryName(Core.NATIVE_LIBRARY_NAME))
} else {
File("lib\\opencv-4.9\\x86\\" + System.mapLibraryName(Core.NATIVE_LIBRARY_NAME))
}
System.load(lib.absolutePath)
}
println("OpenCV: ${Core.VERSION}")
}
data class MouseState(
val image: Mat,
var second: Point = Point(),
var first: Point = Point(),
var drawing: Boolean = false
)
@JvmStatic
fun main(args: Array<String>) {
change()
}
private fun change() {
val image = Mat(700, 1000, opencv_core.CV_8UC3, Scalar(255.0, 255.0, 255.0, 0.0))
val windowName = "Kotlin Mouse Drawing"
opencv_highgui.namedWindow(windowName, opencv_highgui.WINDOW_AUTOSIZE)
val state = MouseState(image)
val mouseCallback = object : MouseCallback() {
override fun call(event: Int, x: Int, y: Int, flags: Int, params: Pointer?) {
when (event) {
opencv_highgui.EVENT_LBUTTONDOWN -> {
state.drawing = true
state.first.x(x)
state.first.y(y)
}
opencv_highgui.EVENT_MOUSEMOVE -> {
if (state.drawing) {
state.second.x(x)
state.second.y(y)
opencv_imgproc.line(
image,
state.first,
state.second,
Scalar(0.0, 0.0, 255.0, 0.0)
)
state.first.x(state.second.x())
state.first.y(state.second.y())
}
}
opencv_highgui.EVENT_LBUTTONUP -> {
state.drawing = false
}
}
}
}
opencv_highgui.setMouseCallback(windowName, mouseCallback, null)
while (true) {
opencv_highgui.imshow(windowName, image)
if (opencv_highgui.waitKey(1).toChar() == 27.toChar()) {
break
}
}
}
}
3 测试结果
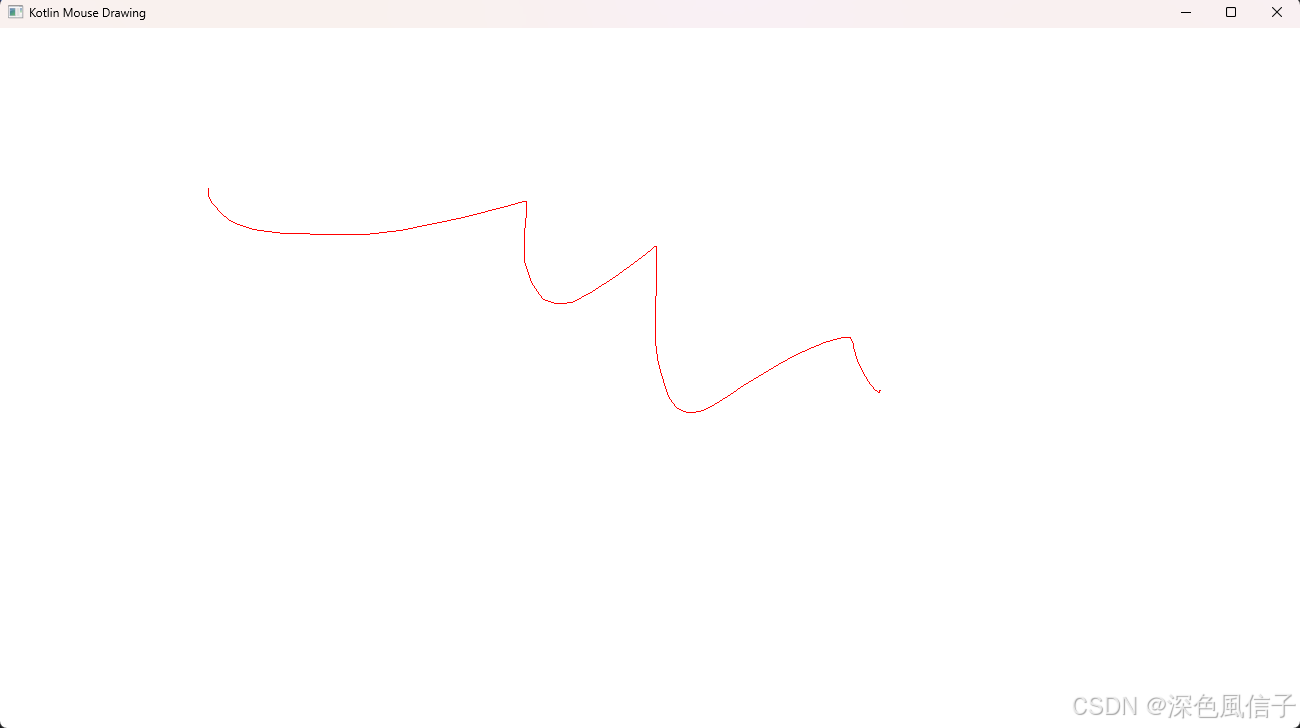