1.思维导图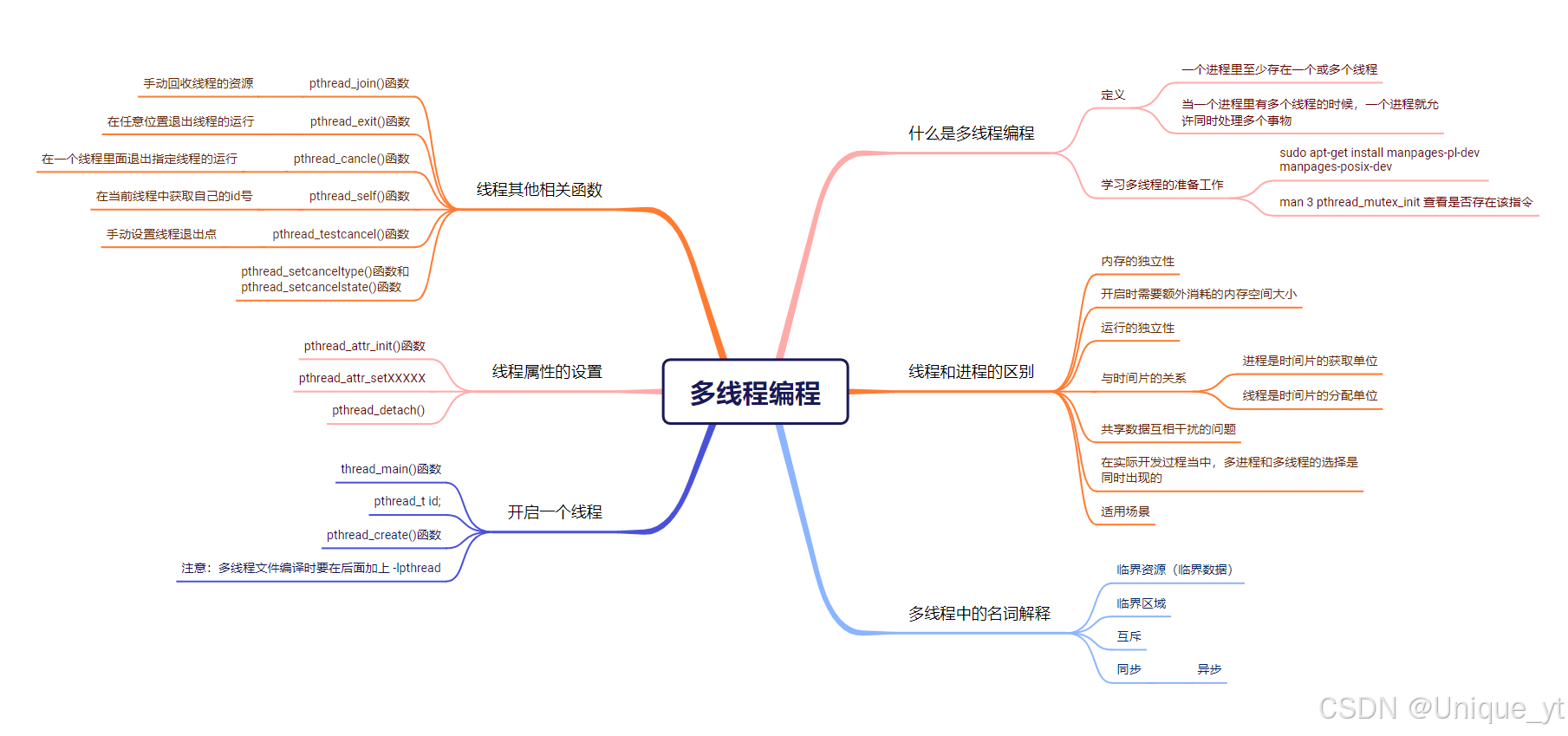
2.创建两个子进程,父进程负责:向文件中写入数据;两个子进程负责:从文件中读取数据。
要求:一定保证1号子进程先读取,2号子进程后读取,使用文件IO去实现。
1>程序代码
#include <stdio.h>
#include <string.h>
#include <unistd.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <pthread.h>
#include <semaphore.h>
#include <wait.h>
#include <signal.h>
#include <sys/socket.h>
#include <arpa/inet.h>
#include <sys/socket.h>
#include <sys/ipc.h>
#include <sys/sem.h>
#include <semaphore.h>
#include <sys/msg.h>
#include <sys/shm.h>
#include <sys/un.h>
typedef struct sockaddr_in addr_in_t;
typedef struct sockaddr addr_t;
typedef struct sockaddr_un addr_un_t;
// 打开文件,写入数据
void task1()
{
int fd = open("t1.txt", O_CREAT | O_RDWR | O_TRUNC, 0666);
if (fd == -1)
{
perror("open");
return;
}
char buf[60] = "Hello world!";
write(fd, buf, sizeof(buf));
close(fd);
}
// 读取数据
void task2()
{
int fd = open("t1.txt", O_RDONLY);
lseek(fd, 0, SEEK_SET);
char buf[60] = {0};
int res;
while ((res = read(fd, buf, 1)) > 0)
{
// 打印读取的字节
putchar(buf[0]);
}
close(fd);
}
int main(int argc, const char *argv[])
{
// 创建第一个子进程
pid_t pid1 = fork();
if (pid1 == 0)
{
sleep(1);
task2();
printf("\n1号子进程读取数据成功!\n");
}
else if (pid1 > 0)
{
// 创建第二个子进程
pid_t pid2 = fork();
if (pid2 == 0)
{
sleep(2);
task2();
printf("\n2号子进程读取数据成功!\n");
}
else if (pid2 > 0)
{
// 父进程的操作
task1();
wait(0);
wait(0);
}
}
return 0;
}
2>运行结果
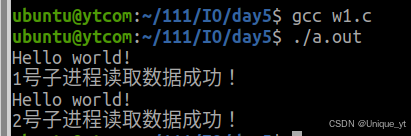
3.创建一个线程(结果为1个主线程和1个分支线程),主线程负责:输入三角形的三条边长;分支线程负责:计算三角形的面积(海伦公式)。
注意:使用sqrt函数编译的时候需要在编译的最后加上 -lm。
1>程序代码
#include <stdio.h>
#include <string.h>
#include <unistd.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <pthread.h>
#include <semaphore.h>
#include <wait.h>
#include <signal.h>
#include <sys/socket.h>
#include <arpa/inet.h>
#include <sys/socket.h>
#include <sys/ipc.h>
#include <sys/sem.h>
#include <semaphore.h>
#include <sys/msg.h>
#include <sys/shm.h>
#include <sys/un.h>
#include <math.h>
typedef struct sockaddr_in addr_in_t;
typedef struct sockaddr addr_t;
typedef struct sockaddr_un addr_un_t;
typedef struct Triangle
{
double a;
double b;
double c;
}Tri,*TriPtr;
void* thread_main(void* arg)
{
TriPtr tri = (TriPtr)arg;
double s = (tri->a + tri->b + tri->c)/2;
double area = sqrt(s*(s-(tri->a))*(s-(tri->b))*(s-(tri->c)));
printf("这个三角形的面积为:%.2lf\n",area);
free(tri);
}
int main(int argc, const char *argv[])
{
TriPtr tri = (TriPtr)malloc(sizeof(Tri));
printf("请输入三角形的三条边:");
scanf("%lf%lf%lf",&(tri->a),&(tri->b),&(tri->c));
pthread_t id;
pthread_create(&id,0,thread_main,(void*) tri);
pthread_join(id,NULL);
free(tri);
return 0;
}
2>运行结果
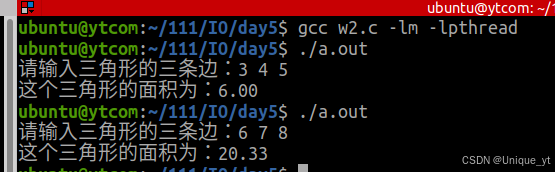