运行效果如下,也可以自己自定义要祝福的人,虽然做的不太好但是也可以先用用
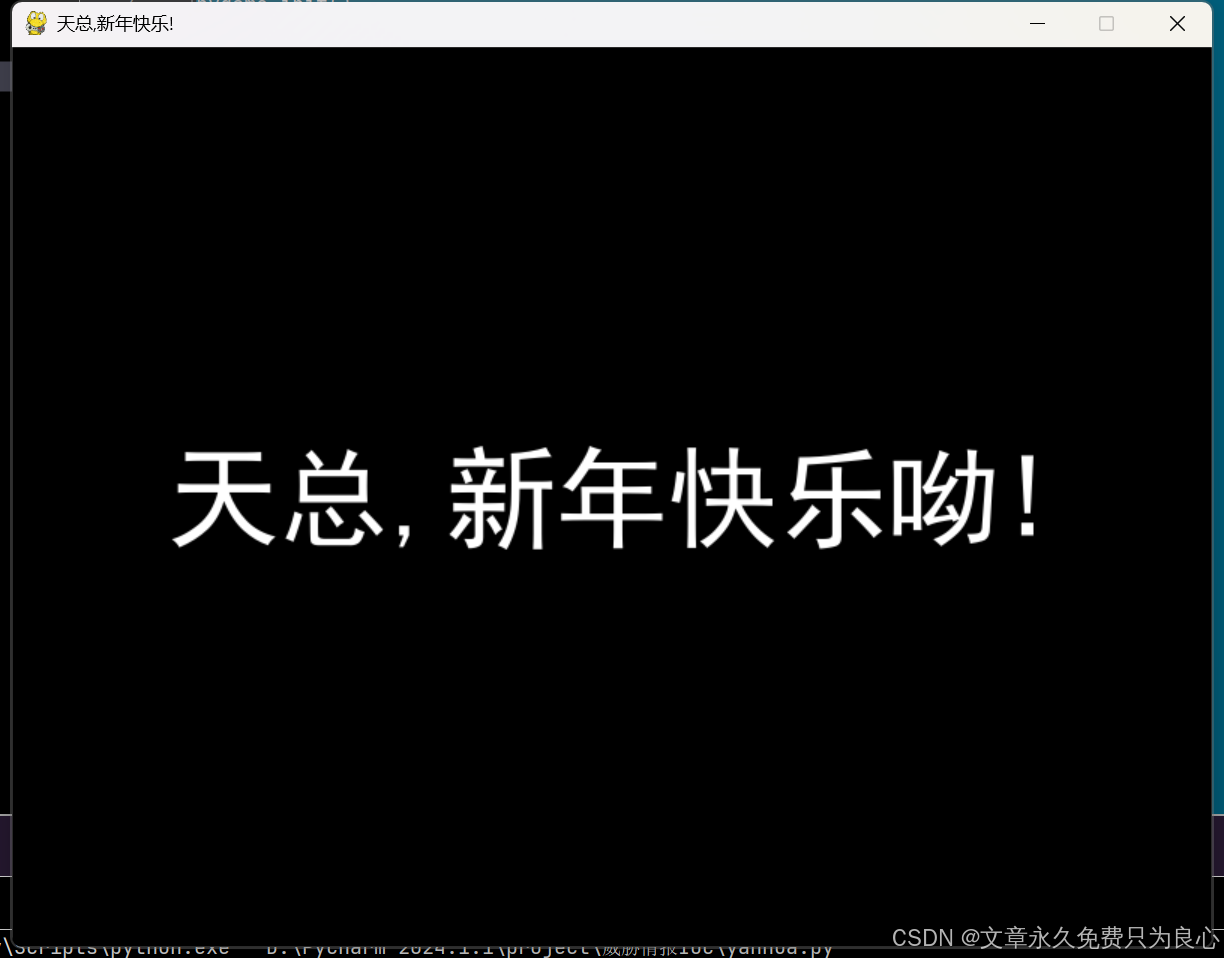
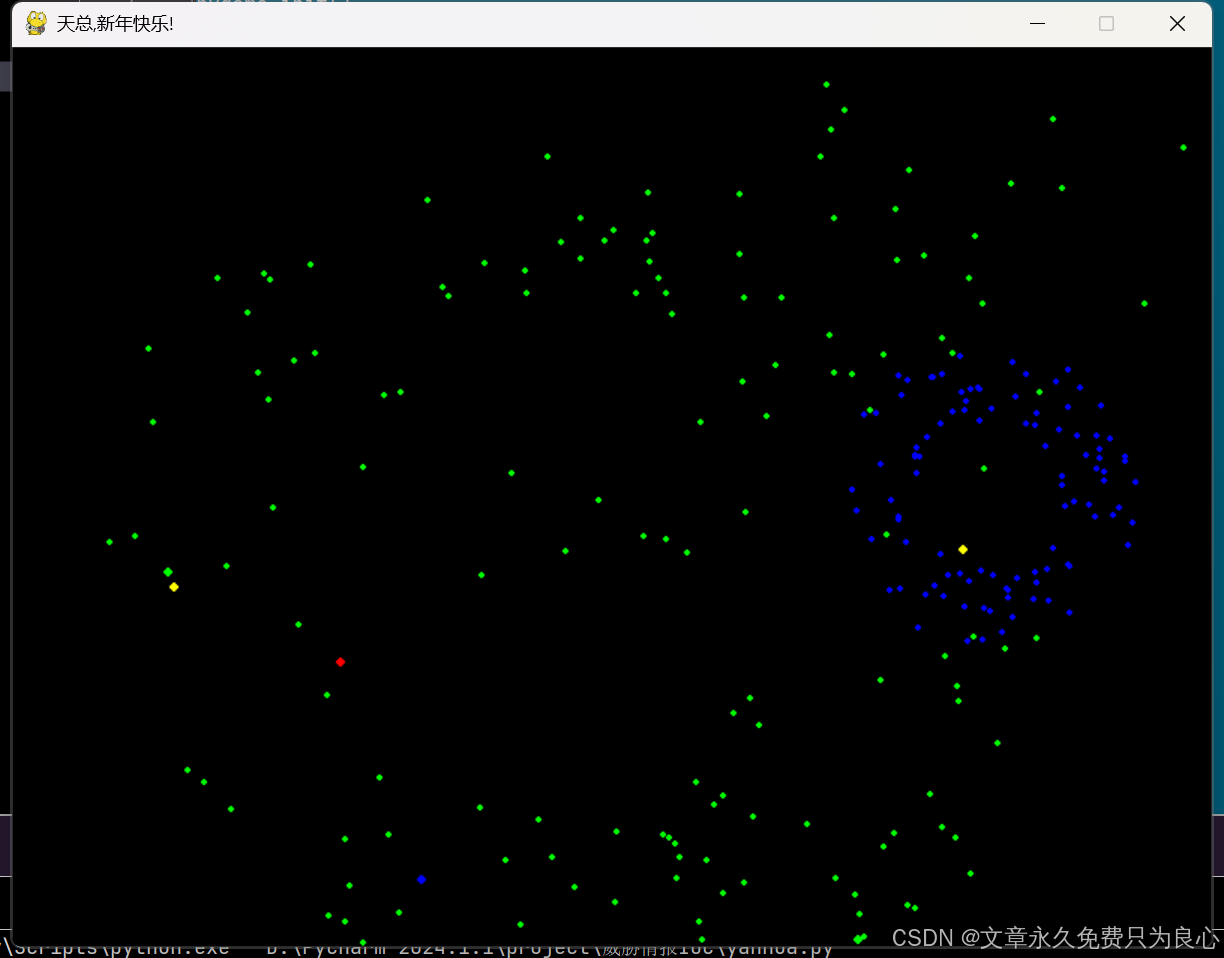
import pygame
import random
import math
# 初始化Pygame
pygame.init()
# 设置窗口大小
width, height = 800, 600
screen = pygame.display.set_mode((width, height))
pygame.display.set_caption("天总,新年快乐!")
# 颜色定义
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
YELLOW = (255, 255, 0)
# 文字设置
font_path = "C:/Windows/Fonts/simhei.ttf" # 替换为你的中文字体路径
font = pygame.font.Font(font_path, 74)
text = font.render("天总,新年快乐呦!", True, WHITE)
text_rect = text.get_rect(center=(width // 2, height // 2))
# 烟花粒子类
class Firework:
def __init__(self, x, y, color):
self.x = x
self.y = y
self.color = color
self.particles = []
self.exploded = False
def add_particle(self):
angle = random.uniform(0, 2 * math.pi)
speed = random.uniform(5, 10)
particle = {
'x': self.x,
'y': self.y,
'vx': speed * math.cos(angle),
'vy': -speed * math.sin(angle),
'life': 50
}
self.particles.append(particle)
def update(self):
if not self.exploded:
self.y -= 5
if self.y < height // 2:
self.exploded = True
for _ in range(100):
self.add_particle()
else:
for particle in self.particles:
particle['x'] += particle['vx']
particle['y'] += particle['vy']
particle['vy'] += 0.1 # 加速度
particle['life'] -= 1
self.particles = [p for p in self.particles if p['life'] > 0]
def draw(self, screen):
if not self.exploded:
pygame.draw.circle(screen, self.color, (int(self.x), int(self.y)), 3)
else:
for particle in self.particles:
pygame.draw.circle(screen, self.color, (int(particle['x']), int(particle['y'])), 2)
# 创建烟花列表
fireworks = []
# 主循环
running = True
show_text = True
text_duration = 3000 # 显示文字的时间(毫秒)
start_time = pygame.time.get_ticks()
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
current_time = pygame.time.get_ticks()
elapsed_time = current_time - start_time
if show_text and elapsed_time >= text_duration:
show_text = False
screen.fill(BLACK)
if show_text:
screen.blit(text, text_rect)
else:
if random.randint(1, 20) == 1:
fireworks.append(Firework(random.randint(100, width - 100), height, random.choice([RED, GREEN, BLUE, YELLOW])))
for firework in fireworks:
firework.update()
firework.draw(screen)
fireworks = [fw for fw in fireworks if not (fw.exploded and len(fw.particles) == 0)]
pygame.display.flip()
pygame.time.delay(30)
pygame.quit()