【Vue3 项目中父子组件之间如何互相传值、传递方法】
在 Vue3 的项目开发中,组件化是非常重要的特性。父子组件之间的通信是组件化开发中常见的需求,本文将详细介绍 Vue3 中父子组件之间如何互相传值以及传递方法。
一、父组件向子组件传值
1. 使用 props
props 是 Vue 中父组件向子组件传值最常用的方式。
- 子组件定义 props:在子组件中,通过props选项来声明接收的数据。例如,我们有一个ChildComponent.vue组件,想要接收父组件传递的message数据:
<template>
<div>
{{ message }}
</div>
</template>
<script setup>
const props = defineProps({
message: String
})
</script>
- 父组件传递数据:在父组件中使用子组件时,通过属性绑定的方式传递数据。假设父组件是ParentComponent.vue:
<template>
<div>
<ChildComponent :message="parentMessage" />
</div>
</template>
<script setup>
import ChildComponent from './ChildComponent.vue'
const parentMessage = 'Hello from parent'
</script>
这样,父组件的parentMessage就传递给了子组件的message。
2. 传递对象和数组
props 不仅可以传递基本类型的数据,也可以传递对象和数组。例如,父组件传递一个对象:
<template>
<div>
<ChildComponent :user="userInfo" />
</div>
</template>
<script setup>
import ChildComponent from './ChildComponent.vue'
const userInfo = { name: 'John', age: 30 }
</script>
子组件接收:
<template>
<div>
<p>Name: {{ user.name }}</p>
<p>Age: {{ user.age }}</p>
</div>
</template>
<script setup>
const props = defineProps({
user: Object
})
</script>
二、父组件向子组件传递方法
1. 通过 props 传递方法
父组件可以将方法作为 props 传递给子组件。
- 父组件定义方法并传递:在父组件ParentComponent.vue中定义一个方法,并将其传递给子组件:
<template>
<div>
<ChildComponent :handleClick="handleParentClick" />
</div>
</template>
<script setup>
import ChildComponent from './ChildComponent.vue'
const handleParentClick = () => {
console.log('Parent method called from child')
}
</script>
- 子组件调用方法:在子组件ChildComponent.vue中通过props调用传递过来的方法:
<template>
<button @click="props.handleClick">Click me</button>
</template>
<script setup>
const props = defineProps({
handleClick: Function
})
</script>
三、子组件向父组件传值
1. 使用自定义事件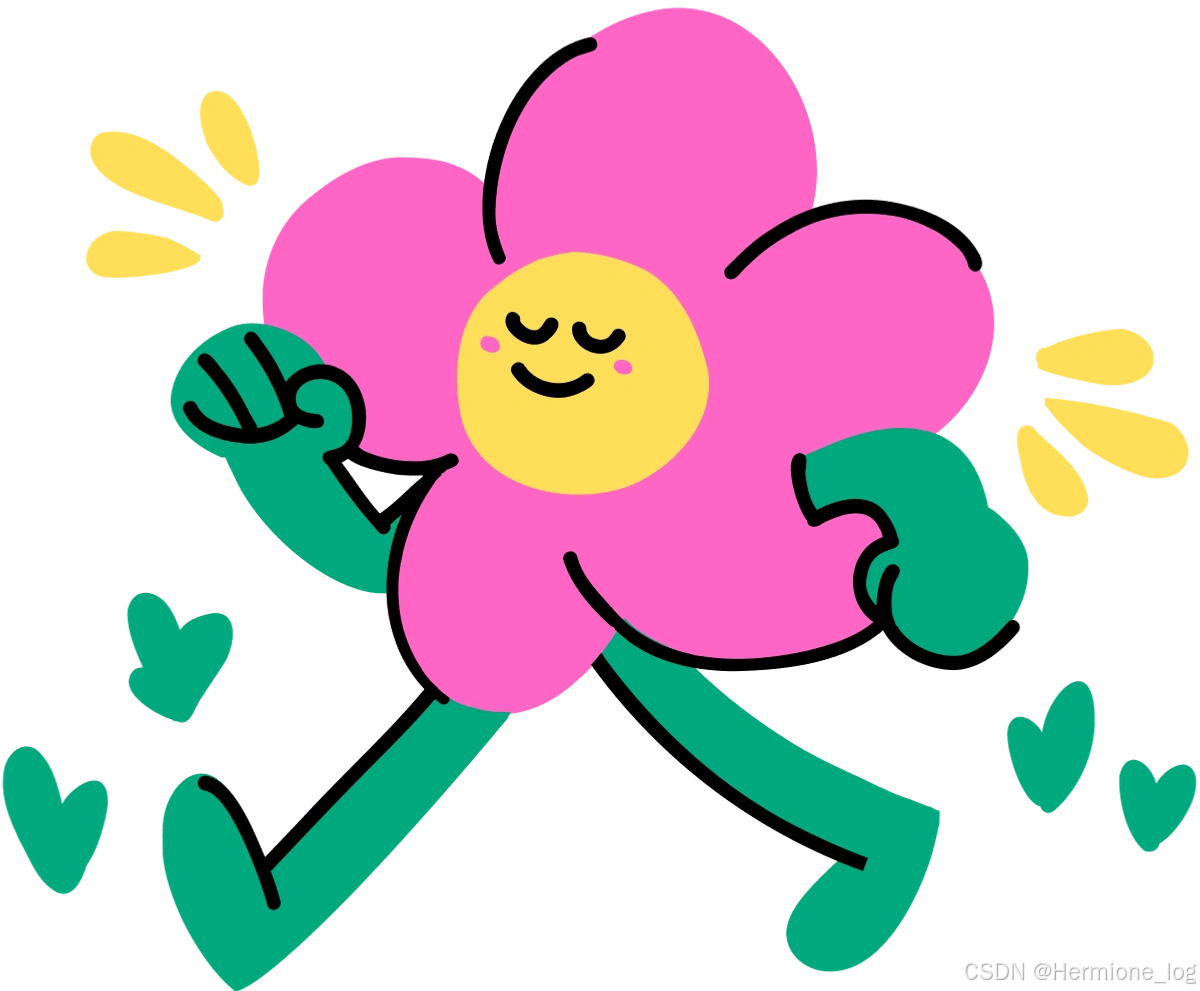
通过$emit触发自定义事件是子组件向父组件传值的常用方式。
- 子组件触发事件并传值:在子组件ChildComponent.vue中,使用defineEmits定义事件并触发,同时传递数据:
<template>
<button @click="sendDataToParent">Send data</button>
</template>
<script setup>
const emit = defineEmits(['send-data'])
const sendDataToParent = () => {
const data = 'Hello from child'
emit('send-data', data)
}
</script>
- 父组件监听事件并接收数据:在父组件ParentComponent.vue中监听子组件触发的事件,并接收数据:
<template>
<div>
<ChildComponent @send-data="handleChildData" />
</div>
</template>
<script setup>
import ChildComponent from './ChildComponent.vue'
const handleChildData = (data) => {
console.log('Received data from child:', data)
}
</script>
四、子组件向父组件传递方法(间接实现)
虽然子组件不能直接向父组件传递方法,但可以通过自定义事件来间接实现类似的效果。
- 子组件触发事件并传递方法相关数据:子组件ChildComponent.vue触发事件并传递一些数据,这些数据可以用于父组件执行相应的逻辑:
<template>
<button @click="sendMethodData">Send method data</button>
</template>
<script setup>
const emit = defineEmits(['send-method-data'])
const sendMethodData = () => {
const methodData = { param: 'example' }
emit('send-method-data', methodData)
}
</script>
- 父组件根据接收到的数据执行方法:父组件ParentComponent.vue根据接收到的数据执行相应的方法:
<template>
<div>
<ChildComponent @send-method-data="handleMethodData" />
</div>
</template>
<script setup>
import ChildComponent from './ChildComponent.vue'
const handleMethodData = (data) => {
// 根据data执行相应的方法逻辑
console.log('Received method data:', data)
}
</script>
五、总结
Vue3 中父子组件之间的传值和传递方法是开发中非常基础且重要的技能。通过 props 可以实现父组件向子组件传值和传递方法,通过自定义事件可以实现子组件向父组件传值以及间接实现传递方法相关的逻辑。熟练掌握这些通信方式,能够帮助我们更好地构建复杂的 Vue3 应用程序。