springboot系列十五:SpringBoot整合MyBatis, MyBatis-Plus
文章目录
- SpringBoot整合MyBatis
- 需求说明
- 代码实现
- 注意事项和细节
- SpringBoot整合MyBatis-Plus
- 官方文档
- 基本介绍
- 应用案例
- 注意事项和细节
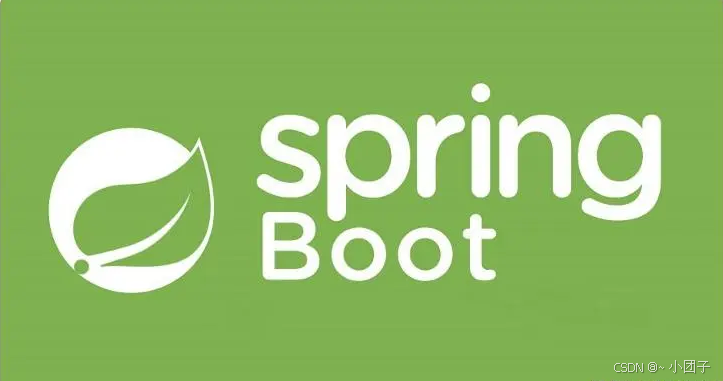
SpringBoot整合MyBatis
需求说明
1.将SpringBoot和MyBatis整合。
2.查出一条数据
代码实现
1.创建数据库和表
CREATE TABLE `monster` (
`id` INT(11) UNSIGNED NOT NULL AUTO_INCREMENT,
`age` TINYINT NOT NULL,
`email` VARCHAR(64) DEFAULT NULL,
`birthday` DATE DEFAULT NULL,
`gender` CHAR(2) DEFAULT NULL,
`name` VARCHAR(255) DEFAULT NULL,
`salary` DECIMAL(10,2) DEFAULT 0.00,
PRIMARY KEY(`id`)
)CHARSET=utf8
2.创建springboot_mybatis
项目, 这里使用灵活创建项目方式.
3.添加pom.xml
<dependencies>
<!--导入web项目场景启动器, 会自动地导入和web开发相关的所有依赖[库/jar]-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!--引入lombok, 使用版本仲裁-->
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</dependency>
<!--引入mybatis starter-->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.2</version>
</dependency>
<!--引入mysql驱动, 使用版本仲裁-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
<!--引入配置处理器 解决yaml文件不提示问题-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-configuration-processor</artifactId>
</dependency>
<!--如何在SpringBoot中开发测试类, 引入starter-test-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
</dependency>
</dependencies>
4.创建src/main/resources/application.yml
server:
port: 9090
spring:
datasource:
url: jdbc:mysql://127.0.0.1:3306/springboot_mybatis?useUnicode=true&characterEncoding=utf-8&useSSL=false
driver-class-name: com.mysql.cj.jdbc.Driver
username: root
password: zhaozhiwei521
5.先创建主程序src/main/java/com/zzw/springboot/mybatis/Application.java
@SpringBootApplication
public class Application {
public static void main(String[] args) {
ApplicationContext ioc = SpringApplication.run(Application.class, args);
}
}
6.再创建springboot测试类src/test/java/com/zzw/springboot/mybatis/ApplicationTest.java
@SpringBootTest
public class ApplicationTest {
@Autowired
public JdbcTemplate jdbcTemplate;
@Test
public void contextLoads() {
//查看底层使用的是什么数据源类型[HikariDataSource]
System.out.println("底层数据源类型=" + jdbcTemplate.getDataSource().getClass());
//底层数据源类型=class com.zaxxer.hikari.HikariDataSource
}
}
7.切换数据源为 druid, 修改pom.xml
参考整合Druid到SpringBoot
8.创建src/main/java/com/zzw/springboot/mybatis/bean/Monster.java
/**
* @author 赵志伟
* @version 1.0
* 在Mapper接口中使用 @Mapper注解, 就会扫描并将该接口注入到ioc容器
*/
@Mapper
public interface MonsterMapper {
//方法 根据id返回Monster对象
public Monster getMonsterById(Integer id);
}
9.配置src/main/resources/mapper/AdminMapper.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.zzw.springboot.mybatis.mapper.MonsterMapper">
<!--配置 getMonsterById()-->
<select id="getMonsterById" resultType="com.zzw.springboot.mybatis.bean.Monster">
select * from monster where id = #{id}
</select>
</mapper>
10.配置yaml
mybatis: # 配置要扫描的XxxMapper.xml
mapper-locations: classpath:mapper/*.xml
11.写测试类
@SpringBootTest
public class ApplicationTest {
@Autowired
public JdbcTemplate jdbcTemplate;
@Autowired
private MonsterMapper monsterMapper;
//测试MonsterMapper接口是否可用
@Test
public void getMonsterById() {
Monster monster = monsterMapper.getMonsterById(1);
System.out.println(monster);
}
}
12.springboot整合mybatis配置
mybatis: # 配置要扫描的XxxMapper.xml
mapper-locations: classpath:mapper/*.xml
#通过config-location 可以指定mybatis-config.xml, 可以以传统的方式来配置mybatis
#config-location:
#也可以直接在application.yml中进行配置
#举例说明1: 比如配置原来的 typeAliases
#举例说明2: 比如配置mybatis的日志输出
type-aliases-package: com.zzw.springboot.mybatis.bean
configuration:
log-impl: org.apache.ibatis.logging.stdout.StdOutImpl
13.使用传统方式配置mybatis
mybatis: # 配置要扫描的XxxMapper.xml
mapper-locations: classpath:mapper/*.xml
#通过config-location 可以指定mybatis-config.xml, 可以以传统的方式来配置mybatis
#config-location:
config-location: classpath:mybatis-config.xml
#也可以直接在application.yml中进行配置
#举例说明1: 比如配置原来的 typeAliases
#举例说明2: 比如配置mybatis的日志输出
# type-aliases-package: com.zzw.springboot.mybatis.bean
# configuration:
# log-impl: org.apache.ibatis.logging.stdout.StdOutImpl
#说明: 配置mybatis的两种方式选择: 如果配置比较简单, 就直接在application.yml中进行配置
#如果配置内容比较复杂, 可以考虑单独地做一个mybatis-config.xml
src/main/resources/mybatis-config.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"https://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<!--配置MyBatis自带的日志输出-查看原生的sql
DOCTYPE规定 <settings/>节点/元素 必须在前面, 放在后面会报错
-->
<settings>
<setting name="logImpl" value="STDOUT_LOGGING"/>
</settings>
<!--配置别名-->
<typeAliases>
<!--
如果一个包下有很多的类, 我们可以直接引入包
, 这样该包下面的所有类名, 可以直接使用
-->
<package name="com.zzw.springboot.mybatis.bean"/>
</typeAliases>
</configuration>
13.新建service层 src/main/java/com/zzw/springboot/mybatis/service/MonsterService.java
public interface MonsterService {
//根据id返回Monster对象
Monster getMonsterById(Integer id);
}
src/main/java/com/zzw/springboot/mybatis/service/impl/MonsterServiceImpl.java
@Service
public class MonsterServiceImpl implements MonsterService {
//装配MonsterMapper
@Resource
private MonsterMapper monsterMapper;
@Override
public Monster getMonsterById(Integer id) {
return monsterMapper.getMonsterById(id);
}
}
14.测试
@SpringBootTest
public class ApplicationTest {
//配置MonsterService
@Resource
private MonsterService monsterService;
//测试MonsterService
@Test
public void getMonsterById() {
Monster monster = monsterService.getMonsterById(1);
System.out.println("monster--" + monster);
}
}
15.配置controller层 src/main/java/com/zzw/springboot/mybatis/controller/MonsterController.java
@Controller
public class MonsterController {
@Autowired
private MonsterService monsterService;
@RequestMapping("/getMonsterById")
@ResponseBody
public Monster getMonsterById(@RequestParam(value = "id") Integer id) {
return monsterService.getMonsterById(id);
}
}
16.测试
注意事项和细节
@Data
public class Monster {
private Integer id;
private Integer age;
//这里通过注解来解决时区问题
//GMT 就是格林尼治标准时间
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss", timezone = "GMT+8")
private Date birthday;
private String email;
private String name;
private String gender;
private Double salary;
}
SpringBoot整合MyBatis-Plus
官方文档
MyBatis-Plus官网: https://baomidou.com/introduce/
基本介绍
1.Mybatis-Plus (简称 MP) 是一个 MyBatis 的增强工具,在MyBatis的基础上只做增强不做改变,为简化开发,提高效率而生。
2.强大的CRUD操作: 内置通用 Mapper,通过Service,通过少量配置即可实现表单大部分CRUD操作,更有强大的条件构造器,满足各类使用需求。
应用案例
需求
1.将Springboot 和 mybatis-plus结合
代码实现
1.创建数据库和表
CREATE DATABASE springboot_mybatisplus;
USE springboot_mybatisplus;
CREATE TABLE monster (
`id` INT (11) UNSIGNED PRIMARY KEY AUTO_INCREMENT,
`age` TINYINT (4) DEFAULT NULL,
`email` VARCHAR (192) DEFAULT NULL,
`birthday` DATE DEFAULT NULL,
`gender` CHAR (6) DEFAULT NULL,
`name` VARCHAR (765) DEFAULT NULL,
`salary` DECIMAL (10,2) DEFAULT 0.00
)ENGINE=INNODB DEFAULT CHARSET=utf8mb4;
INSERT INTO monster (`age`, `email`, `birthday`, `gender`, `name`, `salary`) VALUES
(17, '123@qq.com', '1999-01-01', 'male', '张三', 10000.00),
(18, '123@qq.com', '1999-01-01', 'male', '李四', 10000.00)
2.创建springboot-mybatisplus
项目, 采用灵活方式 参考springboot快速入门
3.引入必要的依赖,参考 去掉mybatis-spring-boot-starter
4.引入mybatis-plus-boot-starter
, 在中央仓库
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.4.3</version>
</dependency>
5.增加druid配置类 src/main/java/com/zzw/springboot/mybatisplus/config/DruidDataSourceConfig.java
, 参考整合Druid到SpringBoot
6.创建src/main/resources/application.yml
server:
port: 9090
spring:
datasource:
url: jdbc:mysql://127.0.0.1:3306/springboot_mybatis?useUnicode=true&characterEncoding=utf-8&useSSL=false
driver-class-name: com.mysql.cj.jdbc.Driver
username: root
password: zhaozhiwei521
6.plus启动成功 (标志)
7.创建src/main/java/com/zzw/springboot/mybatisplus/bean/Monster.java
@Data
public class Monster {
private Integer id;
private Integer age;
//这里通过注解来解决时区问题
//GMT 就是格林尼治标准时间
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss", timezone = "GMT+8")
private Date birthday;
private String email;
private String name;
private String gender;
private Double salary;
}
8.新建src/main/java/com/zzw/springboot/mybatisplus/mapper/MonsterMapper.java
/**
* @author 赵志伟
* @version 1.0
* 解读
* 1.BaseMapper已经默认提供了很多的crud方法, 可以直接使用
* 2.如果BaseMapper提供的方法不能满足业务需求, 我们可以再开发新的方法, 并在MonsterMapper.xml中进行配置
*/
@Mapper
public interface MonsterMapper extends BaseMapper<Monster> {
}
9.测试src/test/java/com/zzw/springboot/mybatisplus/ApplicationTest.java
@SpringBootTest
public class ApplicationTest {
@Autowired
private MonsterMapper monsterMapper;
@Test
public void getMonsterById() {
Monster monster = monsterMapper.selectById(1);
System.out.println("monster=" + monster);
}
}
10.新建src/main/java/com/zzw/springboot/mybatisplus/service/MonsterService.java
/**
* @author 赵志伟
* @version 1.0
* 1.传统方式: 在接口中 定义方法/声明方法, 然后在实现类中进行实现
* 2.在mybatis-plus中, 我们可以继承父接口 IService
* 3.这个IService接口声明了很多方法, 比如crud
* 4.如果默认提供方法不能满足需求, 我们可以再声明需要的方法, 然后在实现类中进行实现即可.
*/
public interface MonsterService extends IService<Monster> {
//自定义方法
void t1();
}
src/main/java/com/zzw/springboot/mybatisplus/service/impl/MonsterServiceImpl.java
/**
* @author 赵志伟
* @version 1.0
* 1.传统方式: 在实现类中直接进行 implementsMonsterService
* 2.在mybatis-plus中, 我们开发Service实现类, 需要继承 ServiceImpl
* 3.我们看到 ServiceImpl类实现了IService
* 4.MonsterService接口集成了IService
* 5.MonsterServiceImpl就可以认为是实现了MonsterService接口, 这样MonsterService
* 就可以使用IService接口方法, 也可以理解成可以使用MonsterService方法.
* 6.如果MonsterService接口中, 声明了其它的方法/自定义方法, 那么我们依然需要在MonsterServiceImpl类中,
* 进行实现
*/
@Service
public class MonsterServiceImpl extends ServiceImpl<MonsterMapper, Monster> implements MonsterService {
@Override
public void t1() {
}
}
11.测试
@SpringBootTest
public class ApplicationTest {
@Autowired
private MonsterService monsterService;
@Test
public void testMonsterService() {
Monster monster = monsterService.getById(1);
System.out.println("testMonsterService monster=" + monster);
}
}
12.创建src/main/java/com/zzw/springboot/mybatisplus/controller/MonsterController.java
@Controller
public class MonsterController {
@Autowired
private MonsterService monsterService;
@ResponseBody
@RequestMapping("getMonsterById")
public Monster getMonsterById(@RequestParam(value = "id") Integer id) {
Monster monster = monsterService.getById(id);
return monster;
}
//返回所有的Monster信息
//后面讨论分页问题
@ResponseBody
@RequestMapping("getAll")
public List<Monster> getAll() {
List<Monster> monsters = monsterService.list();
return monsters;
}
}
13.测试
14.注销掉MonsterMapper的@Mapper注解,在启动类加上@MapperScan注解
/**
* @author 赵志伟
* @version 1.0
* 1.使用@MapperScan 可以指定要扫描的Mapper接口
* 2.属性basePackages/value 可以指定多个包, 这里我们指定的是com.zzw.springboot.mybatisplus.mapper包
*/
//@MapperScan(value = {"com.zzw.springboot.mybatisplus.mapper"})
@MapperScan(basePackages = {"com.zzw.springboot.mybatisplus.mapper"})
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
注意事项和细节
1.@TableName作用
/**
* @author 赵志伟
* @version 1.0
* 1.如果实体类Monster和表名monster对应, 就可以映射上, 则@TableName可省略
* 2.如果实体类Monster和表名monster_不对应, 则需要使用@TableName进行指定
*/
@Data
//@TableName(value = "monster")
public class Monster {
private Integer id;
private Integer age;
}
2.Mybatis-Plus starter 到底引入了哪些依赖?
3.为了开发方便, 可以安装 MyBatisX 插件.
官方文档: https://baomidou.com/guides/mybatis-x/
(1)新建src/main/resources/mapper/MonsterMapper.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.zzw.springboot.mybatisplus.mapper.MonsterMapper">
</mapper>
(2)演示一下自动生成 插入sql