1.BST树介绍
#include "pch.h"
#include <iostream>
#include <queue>
#include <stack>
#include <vector>
using namespace std;
template<typename T>
class BSTree
{
public:
BSTree() :_root(nullptr) {}
void noninsert(const T &val)
{
if (_root == nullptr)
{
_root = new BSTNode(val);
return;
}
BSTNode *pre = nullptr;
BSTNode *cur = _root;
while (cur != nullptr)
{
pre = cur;
if (val < cur->_data)
{
cur = cur->_left;
}
else if (val > cur->_data)
{
cur = cur->_right;
}
else
{
return;
}
}
if (val < pre->_data)
{
pre->_left = new BSTNode(val);
}
else
{
pre->_right = new BSTNode(val);
}
}
void nonremove(const T &val)
{
BSTNode *pre = nullptr;
BSTNode *cur = _root;
while (cur != nullptr)
{
if (val < cur->_data)
{
pre = cur;
cur = cur->_left;
}
else if (val > cur->_data)
{
pre = cur;
cur = cur->_right;
}
else
{
break;
}
}
if (cur == nullptr)
return;
if (cur->_left != nullptr && cur->_right != nullptr)
{
BSTNode *old = cur;
pre = cur;
cur = cur->_left;
while (cur->_right != nullptr)
{
pre = cur;
cur = cur->_right;
}
old->_data = cur->_data;
}
BSTNode *child = cur->_left;
if (child == nullptr)
{
child = cur->_right;
}
if (pre == nullptr)
{
_root = child;
}
else
{
if (cur == pre->_left)
{
pre->_left = child;
}
else
{
pre->_right = child;
}
}
delete cur;
}
void mirror()
{
mirror(_root);
}
bool isBSTree()
{
BSTNode *pre = nullptr;
return isBSTree(_root, pre);
}
void findAreaData(int first, int last)
{
vector<int> vec;
findAreaData(_root, first, last, vec);
for (int v : vec)
{
cout << v << " ";
}
cout << endl;
}
int getLCA(int val1, int val2)
{
BSTNode *p = getLCA(_root, val1, val2);
if (p != nullptr)
{
return p->_data;
}
else
{
throw "no LCA node!";
}
}
int getLastKValue(int k)
{
int i = 0;
BSTNode *p = getLastKValue(_root, k, i);
if (p != nullptr)
{
return p->_data;
}
else
{
throw "no last k value, k is invalid!";
}
}
bool isChildTree(const BSTree<T> &tree)
{
if (_root == nullptr)
return false;
BSTNode *cur = _root;
while (cur != nullptr)
{
if (tree._root->_data > cur->_data)
{
cur = cur->_right;
}
else if (tree._root->_data < cur->_data)
{
cur = cur->_left;
}
else
{
break;
}
}
if (cur == nullptr)
return false;
return isChildTree(cur, tree._root);
}
void rebuildBSTree(int pre[], int len1, int in[], int len2)
{
this->_root = rebuildBSTree(pre, 0, len1-1, in, 0, len2-1);
}
int number()
{
return number(_root);
}
int level()
{
return level(_root);
}
void preOrder()
{
cout << "递归实现前序遍历:";
preOrder(_root);
cout << endl;
}
void inOrder()
{
cout << "递归实现中序遍历:";
inOrder(_root);
cout << endl;
}
void postOrder()
{
cout << "递归实现后序遍历:";
postOrder(_root);
cout << endl;
}
void levelOrder()
{
cout << "递归实现层序遍历:";
int l = level(_root);
for (int i = 0; i < l; ++i)
{
levelOrder(_root, i);
}
cout << endl;
}
void nonpreOrder()
{
if (_root == nullptr)
return;
stack<BSTNode*> s;
s.push(_root);
while (!s.empty())
{
BSTNode *top = s.top();
s.pop();
cout << top->_data << " ";
if (top->_right != nullptr)
{
s.push(top->_right);
}
if (top->_left != nullptr)
{
s.push(top->_left);
}
}
cout << endl;
}
void noninOrder()
{
if (_root == nullptr)
return;
stack<BSTNode*> s;
BSTNode *cur = _root;
while (!s.empty() || cur != nullptr)
{
if(cur != nullptr)
{
s.push(cur);
cur = cur->_left;
}
else
{
BSTNode *top = s.top();
s.pop();
cout << top->_data << " ";
cur = top->_right;
}
}
cout << endl;
}
void nonpostOrder()
{
if (_root == nullptr)
return;
stack<BSTNode*> s1;
stack<BSTNode*> s2;
s1.push(_root);
while (!s1.empty())
{
BSTNode *top = s1.top();
s1.pop();
if (top->_left != nullptr)
{
s1.push(top->_left);
}
if (top->_right != nullptr)
{
s1.push(top->_right);
}
s2.push(top);
}
while (!s2.empty())
{
cout << s2.top()->_data << " ";
s2.pop();
}
cout << endl;
}
void nonlevelOrder()
{
if (_root == nullptr)
return;
queue<BSTNode*> que;
que.push(_root);
while (!que.empty())
{
BSTNode *front = que.front();
cout << front->_data << " ";
que.pop();
if (front->_left != nullptr)
{
que.push(front->_left);
}
if (front->_right != nullptr)
{
que.push(front->_right);
}
}
}
public:
struct BSTNode
{
BSTNode(T data = T())
:_data(data)
, _left(nullptr)
, _right(nullptr)
{}
T _data;
BSTNode *_left;
BSTNode *_right;
};
void mirror(BSTNode *node)
{
if (node == nullptr)
return;
BSTNode *ptmp = node->_left;
node->_left = node->_right;
node->_right = ptmp;
mirror(node->_left);
mirror(node->_right);
}
bool isBSTree(BSTNode *node, BSTNode *&pre)
{
if (node == nullptr)
{
return true;
}
if (!isBSTree(node->_left, pre))
{
return false;
}
if (pre != nullptr)
{
if (node->_data < pre->_data)
{
return false;
}
}
pre = node;
return isBSTree(node->_right, pre);
}
void findAreaData(BSTNode *node, int first, int last, vector<int> &vec)
{
if (node == nullptr)
return;
findAreaData(node->_left, first, last, vec);
if (node->_data > last)
{
return;
}
if (first <= node->_data && last >= node->_data)
{
vec.push_back(node->_data);
}
findAreaData(node->_right, first, last, vec);
}
BSTNode* getLCA(BSTNode *node, int val1, int val2)
{
if (node == nullptr)
{
return nullptr;
}
if (val1 < node->_data && val2 < node->_data)
{
return getLCA(node->_left, val1, val2);
}
else if (val1 > node->_data && val2 > node->_data)
{
return getLCA(node->_right, val1, val2);
}
else
{
return node;
}
}
BSTNode* getLastKValue(BSTNode *node, int k, int &i)
{
if (node == nullptr)
{
return nullptr;
}
BSTNode *p1 = getLastKValue(node->_right, k, i);
if (p1 != nullptr)
{
return p1;
}
i++;
if (k == i)
{
return node;
}
return getLastKValue(node->_left, k, i);
}
bool isChildTree(BSTNode *father, BSTNode *child)
{
if (father == nullptr && child == nullptr)
{
return true;
}
if (father == nullptr)
{
return false;
}
if (child == nullptr)
{
return true;
}
if (father->_data != child->_data)
{
return false;
}
return isChildTree(father->_left, child->_left)
&& isChildTree(father->_right, child->_right);
}
BSTNode* rebuildBSTree(int pre[], int i, int j, int in[], int m, int n)
{
if (i > j || m > n)
{
return nullptr;
}
BSTNode *root = new BSTNode(pre[i]);
for (int k = m; k <= n; ++k)
{
if (pre[i] == in[k])
{
root->_left = rebuildBSTree(pre, i+1, i+(k-m), in, m, k-1);
root->_right = rebuildBSTree(pre, i+(k - m)+1, j, in, k+1, n);
break;
}
}
return root;
}
int number(BSTNode *node)
{
if (node == nullptr)
return 0;
return 1 + number(node->_left) + number(node->_right);
}
int level(BSTNode *node)
{
if (node == nullptr)
{
return 0;
}
else
{
int left = level(node->_left);
int right = level(node->_right);
return left > right ? left + 1 : right + 1;
}
}
void preOrder(BSTNode *node)
{
if (node != nullptr)
{
cout << node->_data << " ";
preOrder(node->_left);
preOrder(node->_right);
}
}
void inOrder(BSTNode *node)
{
if (node != nullptr)
{
inOrder(node->_left);
cout << node->_data << " ";
inOrder(node->_right);
}
}
void postOrder(BSTNode *node)
{
if (node != nullptr)
{
postOrder(node->_left);
postOrder(node->_right);
cout << node->_data << " ";
}
}
void levelOrder(BSTNode *node, int k)
{
if (node != nullptr)
{
if (k == 0)
{
cout << node->_data << " ";
return;
}
levelOrder(node->_left, k - 1);
levelOrder(node->_right, k - 1);
}
}
BSTNode *_root;
};
int main(int argc, char* argv[])
{
int pre[] = { 40,20,10,25,60,50,70 };
int in[] = { 10,20,25,40,50,60,70 };
BSTree<int> bst1;
bst1.rebuildBSTree(pre, sizeof(pre)/sizeof(pre[0]),
in, sizeof(in)/sizeof(in[0]));
bst1.preOrder();
bst1.inOrder();
#if 0
BSTree<int> bst;
BSTree<int>::BSTNode *n1 = new BSTree<int>::BSTNode(40);
BSTree<int>::BSTNode *n2 = new BSTree<int>::BSTNode(20);
BSTree<int>::BSTNode *n3 = new BSTree<int>::BSTNode(60);
BSTree<int>::BSTNode *n4 = new BSTree<int>::BSTNode(55);
BSTree<int>::BSTNode *n5 = new BSTree<int>::BSTNode(45);
BSTree<int>::BSTNode *n6 = new BSTree<int>::BSTNode(70);
bst._root = n1;
n1->_left = n2;
n1->_right = n3;
n3->_left = n4;
n2->_right = n6;
cout << bst.isBSTree() << endl;
#endif
#if 0
BSTree<int> bst;
bst.noninsert(40);
bst.noninsert(20);
bst.noninsert(60);
bst.noninsert(25);
bst.noninsert(45);
bst.noninsert(70);
bst.nonlevelOrder();
cout << endl;
cout << bst.level() << endl;
cout << endl;
bst.preOrder();
bst.nonpreOrder();
bst.inOrder();
bst.noninOrder();
bst.postOrder();
bst.nonpostOrder();
bst.levelOrder();
#endif
return 0;
}
2.数据结构
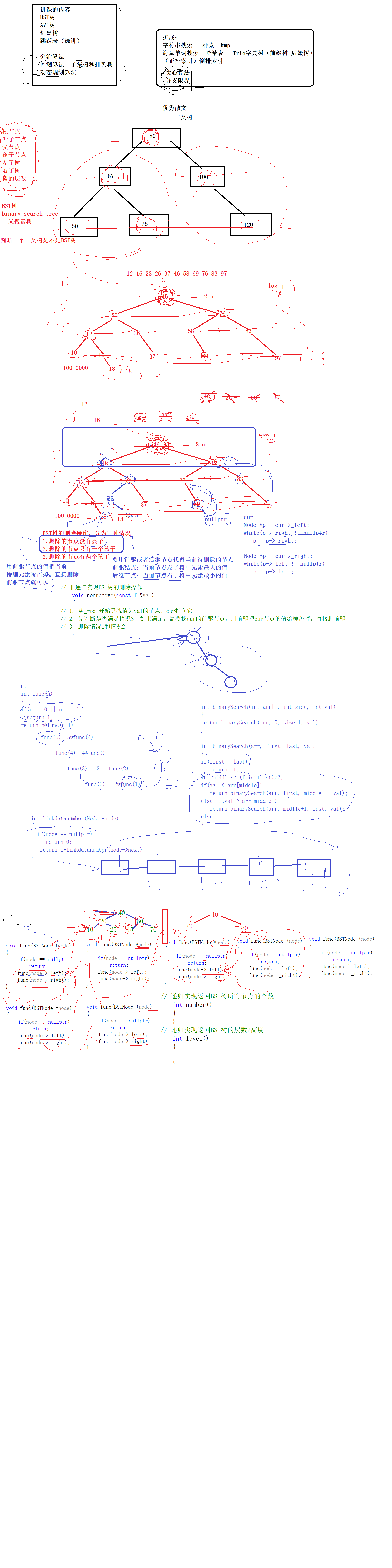
3.遍历方式
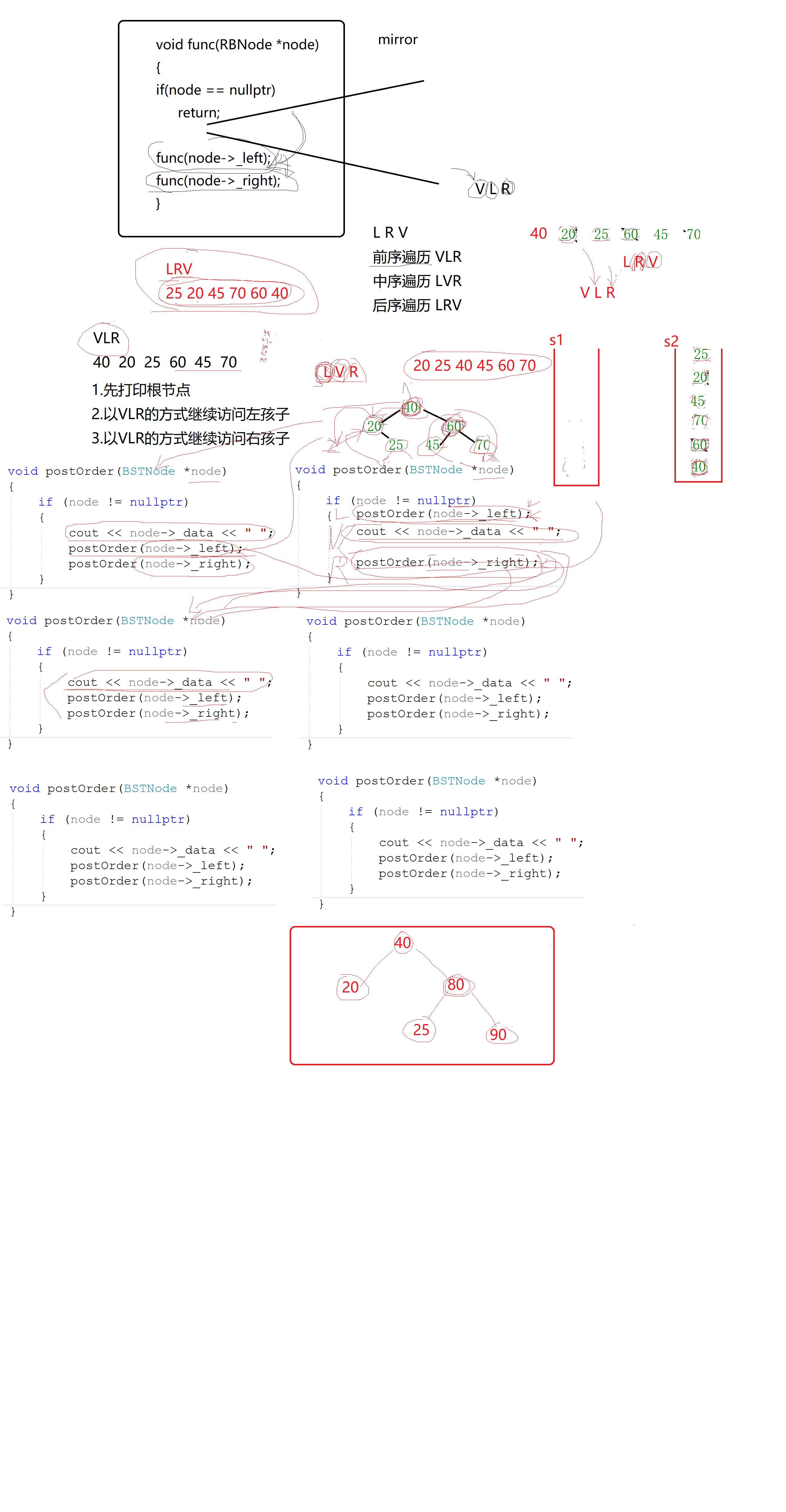