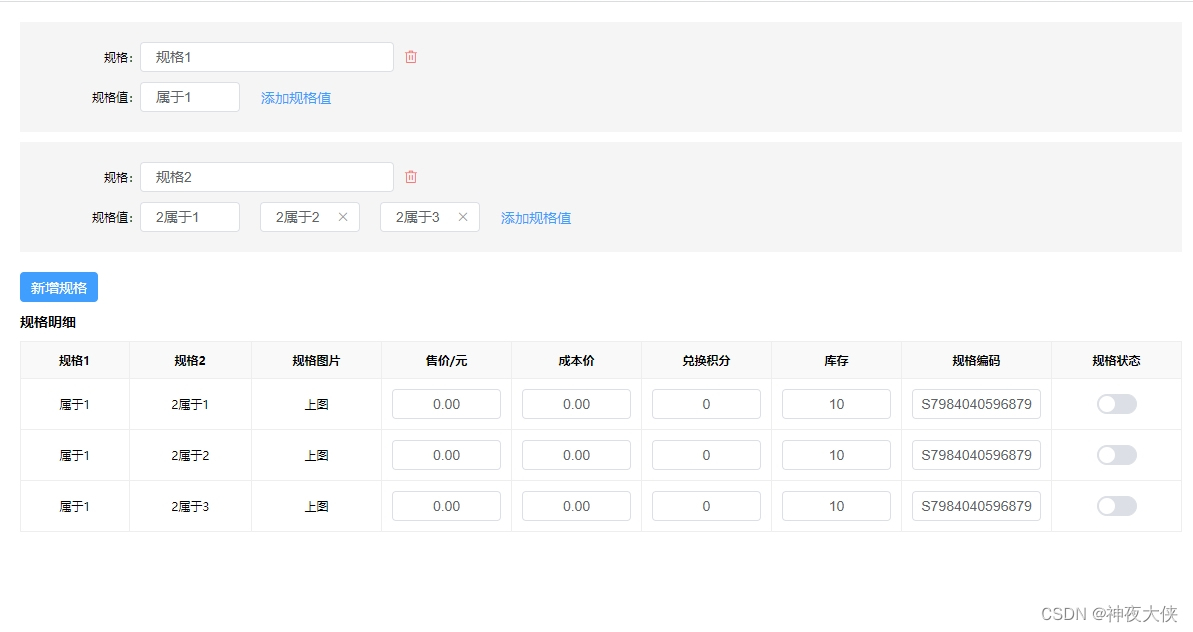
<!DOCTYPE html>
<html>
<head>
<title>商品SKU</title>
<link rel="stylesheet" href="element/css/element.css">
<style>
*{ margin:0; padding:0px; box-sizing: border-box; }
ul,li{ list-style-type: none;}
body{ font-size: 12px;}
[v-lock]{ display: none;}
.el-input__inner{ height:30px; line-height:30px;}
.el-button{height:30px; line-height:30px; padding:0 10px; }
.el-button.is-round{ height:30px; line-height:30px; background: none; border:0; color:#F56C6C; padding:0 10px;}
.flex{ display: flex; align-items: center;}
.between{ display: flex; align-items: center; justify-content: space-between;}
.center{display: flex; align-items: center; justify-content: center;}
.mt10{ margin-top:10px;}
.specbox{ padding:20px; }
.specbox .spec-top li{ background:#f5f5f5; padding:20px; margin-bottom: 10px; }
.specbox .spec-top li .tit{ width:100px; text-align: right; line-height:30px;}
.specbox .spec-top li .hm{ display: flex;}
.specbox .spec-top li .hm .tit{ margin-top:10px;}
.specbox .spec-top li .val{ flex: 1; display: flex; flex-wrap: wrap; }
.specbox .spec-top li .val .itm{ margin:10px 20px 0px 0; position: relative;}
.specbox .spec-top li .val .itm .el-input__inner{ width:100px;}
.specbox .spec-top li .val .itm .c{ position: absolute; right:0; top:0; color:#999; }
.specbox .spec-top li .val .itm .add{ padding:0;}
.specbox .spec-table{ border-collapse:collapse; width:100%; border-left:1px solid #eee; border-top:1px solid #eee;}
.specbox .spec-table tr th{ border-bottom: 1px solid #eee; background:#f9f9f9; border-right: 1px solid #eee; padding:10px;}
.specbox .spec-table tr td{ text-align: center; border-bottom: 1px solid #eee; width:130px; background:#fff; border-right: 1px solid #eee; padding:10px;}
.specbox .spec-table tr td .el-input__inner{ text-align: center; padding:0 5px;}
.specbox .spec-table tr td.td1{width:auto;}
</style>
</head>
<body>
<div id="app" v-lock>
<div class="specbox">
<ul class="spec-top">
<li v-for="(item,index) in specList" :key="index">
<div class="ht flex">
<div class="tit">规格:</div>
<div class="val">
<el-input style="width:254px;" v-model="item.label" placeholder="请输入规格名称"></el-input>
<el-button type="danger" icon="el-icon-delete" @click="delSpec(index)" round></el-button>
</div>
</div>
<div class="hm">
<div class="tit">规格值:</div>
<div class="val">
<div class="itm flex" v-for="(a,i) in item.attr" :key="i">
<el-input v-model="a" placeholder="请输入"></el-input>
<el-button class="c" v-if="i!=0" type="danger" @click="delAttr(item.attr,i)" icon="el-icon-close" round>
</div>
<div class="itm"><el-button type="text" @click="addAttr(item.attr)" class="add">添加规格值</el-button></div>
</div>
</div>
</li>
</ul>
<el-button type="primary" class="mt10" @click="addSpec">新增规格</el-button>
<h3 class="mt10">规格明细</h3>
<table class="spec-table mt10">
<tr>
<th v-for="(item,index) in specList" :key="index">{{item.label}}</th>
<th>规格图片</th>
<th>售价/元</th>
<th>成本价</th>
<th>兑换积分</th>
<th>库存</th>
<th>规格编码</th>
<th>规格状态</th>
</tr>
<tr v-for="(item,index) in specData" :key="index">
<td class="td1" v-for="(a,i) in item.spec" :key="i">{{a}}</td>
<td>上图</td>
<td><el-input v-model="item.price"></el-input></td>
<td><el-input v-model="item.costPrice"></el-input></td>
<td><el-input v-model="item.score"></el-input></td>
<td><el-input v-model="item.stock"></el-input></td>
<td style="width:150px;"><el-input v-model="item.code"></el-input></td>
<td><div class="center"><el-switch v-model="item.status"></el-switch></div></td>
</tr>
</table>
</div>
</div>
<script src="element/js/vue.min.js"></script>
<script src="element/js/element.js"></script>
<script>
new Vue({
el:'#app',
data:{
specList:[
{label:'规格1', attr:['属于1']},
{label:'规格2', attr:['2属于1','2属于2','2属于3']}
],
specData:[],
specItem: {img:'',price:'0.00',costPrice:'0.00',score:0,stock:10, code:'', status:1}
},
watch:{
specList:{
handler:function(newVal,oldVal){
this.setSpecData(newVal)
},
deep:true
}
},
mounted() {
this.specItem.code = this.getRand(14,'S');
this.setSpecData(this.specList)
},
methods:{
getRand(length, prefix) {
return (function (time, code) {
code += parseInt(time.substr(0, 1)) + parseInt(time.substr(1, 1)) + time.substr(2, 8);
while (code.length < length) code += Math.round(Math.random() * 10);
return code;
})(Date.now().toString(), prefix || '' + '')
},
// 递归函数,用于生成所有规格组合的算法
generateSpecifications(specs, index, current, result) {
if (index === specs.length) {
result.push(current);
return;
}
for (var i = 0; i < specs[index].attr.length; i++) {
var next = current.slice();
next.push(specs[index].attr[i]);
this.generateSpecifications(specs, index + 1, next, result);
}
},
//设置规格值
setSpecData(arr){
let result = [], list = [];
this.generateSpecifications(arr, 0, [], result);
result.forEach(res=>{
list.push({spec:res,...this.specItem})
})
this.specData = list
},
addSpec(){
let len = this.specList.length+1
this.specList.push({label:'规格'+len, attr:['属于1']})
this.setSpecData(this.specList)
},
addAttr(attr, i){
let len = attr.length+1
attr.push('属性'+len)
},
delSpec(index){
this.specList.splice(index,1)
},
delAttr(attr,i){
attr.splice(i,1);
},
}
})
</script>
</body>
</html>