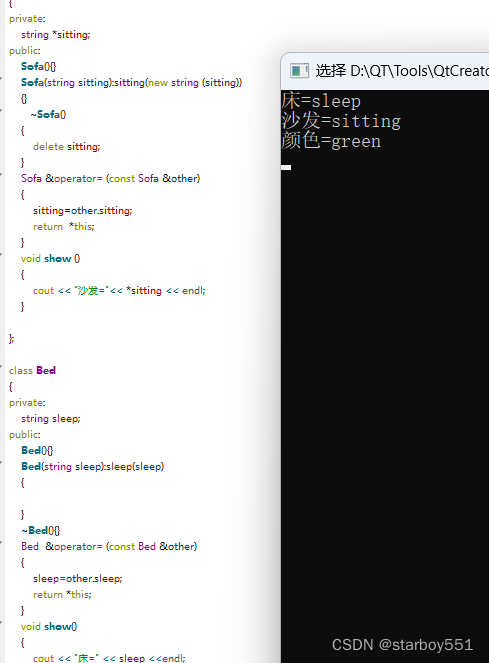
#include <iostream>
using namespace std;
//定义沙发类
class Sofa
{
private:
string *sitting;
public:
Sofa(){}//无参构造函数
Sofa(string sitting):sitting(new string (sitting))//有参构造函数
{}
~Sofa() //析构函数
{
delete sitting;
}
Sofa &operator= (const Sofa & other) //拷贝赋值函数
{
sitting=other.sitting;
return *this;
}
void show ()
{
cout << "沙发="<< *sitting << endl;
}
};
//定义床类
class Bed
{
private:
string sleep;
public:
Bed(){}
Bed(string sleep):sleep(sleep)
{
}
~Bed(){}
Bed &operator= (const Bed &other)
{
sleep=other.sleep;
return *this;
}
void show()
{
cout << "床=" << sleep <<endl;
}
};
//定义沙发床类
class Bed_Sofa:public Bed,public Sofa
{
private:
string color;
public:
Bed_Sofa(){}
Bed_Sofa(string color,string sitting ,string sleep):Bed(sleep),Sofa(sitting),color(color)
{}
Bed_Sofa &operator=(const Bed_Sofa &other)
{
if(this != &other )
{
Sofa::operator=(other);
Bed::operator=(other);
this->color=other.color;
}
return *this;
}
~Bed_Sofa()
{
}
void show()
{
Bed::show();
Sofa::show();
cout <<"颜色=" << color << endl;
}
};
int main()
{
Bed_Sofa Bs1("green" ,"sitting","sleep");
Bs1.show();
return 0;
}