<!DOCTYPE html>
<head>
<meta charset="UTF-8">
<title>Dynamic Tabs with Table Data</title>
<style>
.tab-content {
display: none;
border: 1px solid #ccc;
padding: 1px;
margin-top: 0px;
}
.tab-content.active {
display: block;
}
button {
margin-right: 0px;
background-color: transparent;
border: 1px solid #ccc;
cursor: pointer;
padding: 5px 10px;
}
.active-tab {
background-color: rgb(77, 218, 223);
color: white;
border-color: rgb(47, 178, 183);
}
table {
width: 100%;
border-collapse: collapse;
}
th, td {
border: 1px solid #ddd;
padding: 8px;
text-align: left;
}
th {
background-color: #f2f2f2;
}
</style>
</head>
<body>
<div id="tabs">
</div>
<div id="tab-contents">
</div>
<script>
const data = {
"datasets": [
{
"name": "Dataset 1",
"data": [
{ "num1": 1234, "num2": 5678, "status1": "on", "status2": "active" },
]
},
{
"name": "Dataset 2",
"data": [
{ "num1": 3456, "num2": 7890, "status1": "off", "status2": "inactive" },
]
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Dynamic Tabs with Table Data</title>
<style>
.tab-content {
display: none;
border: 1px solid #ccc;
padding: 1px;
margin-top: 0px;
}
.tab-content.active {
display: block;
}
button {
margin-right: 0px;
background-color: transparent;
border: 1px solid #ccc;
cursor: pointer;
padding: 5px 10px;
}
.active-tab {
background-color: rgb(77, 218, 223);
color: white;
border-color: rgb(47, 178, 183);
}
table {
width: 100%;
border-collapse: collapse;
}
th, td {
border: 1px solid #ddd;
padding: 8px;
text-align: left;
}
th {
background-color: #f2f2f2;
}
</style>
</head>
<body>
<div id="tabs"></div>
<div id="tab-contents"></div>
<script>
const data = {
"datasets": [
{
"name": "Dataset 1",
"data": [
{ "num1": 1234, "num2": 5678, "status1": "on", "status2": "active" },
]
},
{
"name": "Dataset 2",
"data": [
{ "num1": 3456, "num2": 7890, "status1": "off", "status2": "inactive" },
]
}
]
};
function toggleTab(button, index) {
const allTabs = document.querySelectorAll('#tabs button');
allTabs.forEach(tab => tab.classList.remove('active-tab'));
button.classList.add('active-tab');
showTabContent(index);
}
function showTabContent(index) {
const tabContents = document.querySelectorAll('.tab-content');
tabContents.forEach(tabContent => tabContent.classList.remove('active'));
tabContents[index].classList.add('active');
}
function createTable(data) {
const table = document.createElement('table');
const thead = table.createTHead();
const headerRow = thead.insertRow();
['Num1', 'Num2', 'Status1', 'Status2'].forEach(text => {
const th = document.createElement('th');
th.textContent = text;
headerRow.appendChild(th);
});
const tbody = table.createTBody();
data.forEach(item => {
const row = tbody.insertRow();
['num1', 'num2', 'status1', 'status2'].forEach(key => {
const cell = row.insertCell();
cell.textContent = item[key];
});
});
return table;
}
const tabsDiv = document.getElementById('tabs');
const tabContentsDiv = document.getElementById('tab-contents');
data.datasets.forEach((dataset, index) => {
const tabButton = document.createElement('button');
tabButton.textContent = `Tab ${index + 1} (${dataset.name})`;
tabButton.onclick = () => toggleTab(tabButton, index);
tabsDiv.appendChild(tabButton);
const tabContent = document.createElement('div');
tabContent.className = 'tab-content';
const table = createTable(dataset.data);
tabContent.appendChild(table);
tabContentsDiv.appendChild(tabContent);
});
if (data.datasets.length > 0) {
const firstTabButton = tabsDiv.querySelector('button');
firstTabButton.classList.add('active-tab');
const firstTabContent = tabContentsDiv.querySelector('.tab-content');
firstTabContent.classList.add('active');
}
</script>
</body>
</html>
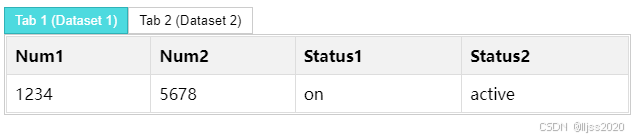