<template>
<view class="flex a-c j-c event-down" :style="{minWidth:`${minWidth}`,textAlign:`${textAlign}`}">
<view class="down-item br-8 flex a-c j-c bold col-fff f-24 lin-24" v-if="Number(day) > 0">
{{day}}
</view>
<view v-if="Number(day) > 0" class="col-fff f-24 lin-24 bold flex a-c j-c down-icon">:</view>
<view class="down-item br-8 flex a-c j-c bold col-fff f-24 lin-24">
{{hou}}
</view>
<view class="col-fff f-24 lin-24 bold flex a-c j-c down-icon">:</view>
<view class="down-item br-8 flex a-c j-c bold col-fff f-24 lin-24">
{{min}}
</view>
<view class="col-fff f-24 lin-24 bold flex a-c j-c down-icon">:</view>
<view class="down-item br-8 flex a-c j-c bold col-fff f-24 lin-24">
{{sec}}
</view>
</view>
</template>
<script>
export default {
name: "count-down",
props: {
currentTime: {
type: [String, Number],
default: 0
},
endTime: {
type: [String, Number],
default: 0
},
minWidth:{
type:String,
default:'100rpx'
},
textAlign:{
type:String,
default:'left'
}
},
data() {
return {
day: '00',
hou: '00',
min: '00',
sec: '00',
}
},
created() {
this.countDown(this.currentTime, this.endTime)
},
beforeDestroy(){
clearInterval(this.interval);
},
methods: {
timeFormat(param) {
return param < 10 ? '0' + param : param;
},
countDown(newTime, endTime) {
clearInterval(this.interval);
this.interval = null;
this.interval = setInterval(() => {
let obj = null;
newTime = newTime + 1000;
if (endTime - newTime > 0) {
let time = (endTime - newTime) / 1000;
let day = parseInt(time / (60 * 60 * 24));
let hou = parseInt(time % (60 * 60 * 24) / 3600);
let min = parseInt(time % (60 * 60 * 24) % 3600 / 60);
let sec = parseInt(time % (60 * 60 * 24) % 3600 % 60);
obj = {
day: this.timeFormat(day),
hou: this.timeFormat(hou),
min: this.timeFormat(min),
sec: this.timeFormat(sec)
};
} else {
obj = {
day: '00',
hou: '00',
min: '00',
sec: '00'
};
clearInterval(this.interval);
}
this.day = obj.day;
this.hou = obj.hou;
this.min = obj.min;
this.sec = obj.sec;
}, 1000);
},
}
}
</script>
<style scoped lang="scss">
.date {
white-space: nowrap;
}
.event-down{
.down-item{
width: 40rpx;
height: 40rpx;
background: #141414;
box-sizing: border-box;
}
.down-icon{
width: 28rpx;
height: 40rpx;
}
}
</style>
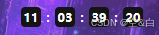