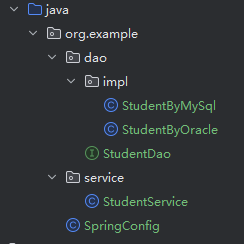
1. 依赖
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>6.1.14</version>
</dependency>
<dependency>
<groupId>jakarta.annotation</groupId>
<artifactId>jakarta.annotation-api</artifactId>
<version>3.0.0</version>
</dependency>
2. 用注解类代替xml配置文件
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
@Configuration
@ComponentScan({"org.example.service", "org.example.dao"})
@ImportResource(locations = {"classpath:spring.xml"})
public class SpringConfig {
}
3. @Resource:非简单类型注入,官方推荐
@Service
public class StudentService {
@Resource(name = "studentByMySql")
private StudentDao studentDao;
public void save() {
studentDao.save();
}
}
4. 测试
@Test
public void testApp() {
ApplicationContext applicationContext = new AnnotationConfigApplicationContext(SpringConfig.class);
StudentService studentService = applicationContext.getBean("studentService", StudentService.class);
System.out.println(studentService);
studentService.save();
}
5. 注解
- 类:
@Component
@Controller
@Service
@Repository
- 属性
@Value
@Resource