1.菜单栏(可以有多个)
void MenuAndToolsAndStatus::Init_Menu()
{
QMenuBar* bar = menuBar();
this->setMenuBar(bar); // 将菜单栏放入主窗口
QMenu* fileMenu = bar->addMenu("文件"); // 创建父节点
// 添加子菜单
QAction* newAction = fileMenu->addAction("新建文件"); // 设置名字
newAction->setShortcut(Qt::CTRL | Qt::Key_A); // 设置快捷键ctrl+a
fileMenu->addSeparator(); // 添加分割线
QAction* openAction = fileMenu->addAction("打开文件"); // 设置名字
openAction->setShortcut(Qt::CTRL | Qt::Key_C); // 设置快捷键ctrl+c // 创建工具栏 (可屏蔽掉 屏蔽掉后底部将失去控件栏位)
//右击弹出菜单
fileMenu->setContextMenuPolicy(Qt::CustomContextMenu);
connect(fileMenu, &QMenu::customContextMenuRequested, this, [=](const QPoint pos) {
QMenu contextMenu;
QAction* action = new QAction(tr("选项1"), this);
QAction* action2 = new QAction(tr("选项2"), this);
contextMenu.addAction(action);
contextMenu.addAction(action2);
//点击
connect(action, &QAction::triggered, this, [=]() {
QMessageBox::information(nullptr, "提示", "选项1", QMessageBox::Ok);
});
contextMenu.exec(mapToGlobal(pos));
});
// 绑定槽函数
connect(newAction, &QAction::triggered, this, [=]() {
QMessageBox::information(nullptr, "提示", "触发新建文件", QMessageBox::Ok);
});
connect(openAction, &QAction::triggered, this, [=]() {
QMessageBox::information(nullptr, "提示", "触发打开文件", QMessageBox::Ok);
});
}
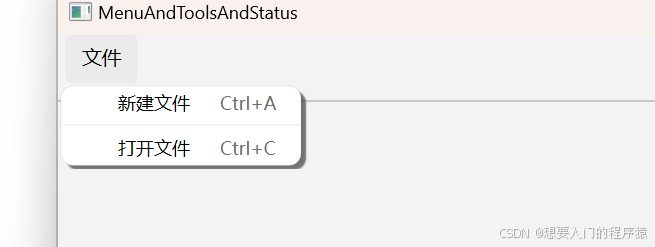
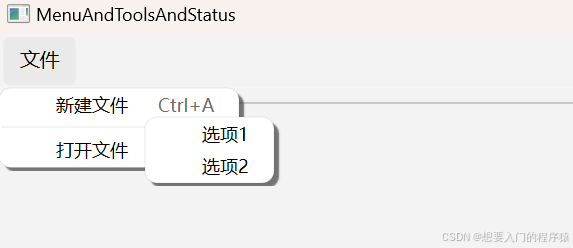
2.工具栏(最多有一个)
void MenuAndToolsAndStatus::Init_ToolBar()
{
// ----------------------------------------------------------
QToolBar* toolBar = new QToolBar(this); // 创建工具栏
addToolBar(Qt::LeftToolBarArea, toolBar); // 设置默认停靠范围
toolBar->setAllowedAreas(Qt::TopToolBarArea | Qt::BottomToolBarArea); // 允许上下拖动
toolBar->setAllowedAreas(Qt::LeftToolBarArea | Qt::RightToolBarArea); // 允许左右拖动
toolBar->setFloatable(false); // 设置是否浮动
toolBar->setMovable(false); // 设置工具栏不允许移动
// 创建快捷项等同于菜单项
QAction* newAction = new QAction("新建");
QAction* openAction = new QAction("保存");
// 工具栏添加菜单项
toolBar->addAction(newAction);
toolBar->addSeparator();
toolBar->addAction(openAction);
openAction->setToolTip("这是保存按钮");
// ----------------------------------------------------------
// 绑定槽函数
// ----------------------------------------------------------
connect(newAction, &QAction::triggered, this, [=]() {
QMessageBox::information(nullptr, "提示", "触发新建文件", QMessageBox::Ok);
});
connect(openAction, &QAction::triggered, this, [=]() {
QMessageBox::information(nullptr, "提示", "触发打开文件", QMessageBox::Ok);
});
// 设置上下文菜单策略
toolBar->setContextMenuPolicy(Qt::CustomContextMenu);
connect(toolBar, &QToolBar::customContextMenuRequested, this, [&](const QPoint pos) {
QMenu contextMenu(tr("上下文菜单"), this);
QAction* action1 = new QAction(tr("选项1"), this);
QAction* action2 = new QAction(tr("选项2"), this);
contextMenu.addAction(action1);
contextMenu.addAction(action2);
connect(action1, &QAction::triggered, this, [=]() {
QMessageBox::information(nullptr, "提示", "选项1", QMessageBox::Ok);
});
contextMenu.exec(mapToGlobal(pos));
});
}
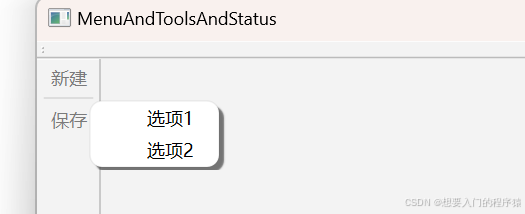
3.二级菜单
void MenuAndToolsAndStatus::Init_QSecondary_Menu()
{
// ----------------------------------------------------------
// 多层菜单导航栏
// ----------------------------------------------------------
QMenuBar* MainMenu = new QMenuBar(this);
this->setMenuBar(MainMenu);
// 1.定义父级菜单
QMenu* EditMenu = MainMenu->addMenu("文件编辑");
// 1.1 定义 EditMemu 下面的子菜单
QAction* text = new QAction(EditMenu);
text->setText("编辑文件"); // 设置文本内容
text->setShortcut(Qt::CTRL | Qt::Key_A); // 设置快捷键ctrl+a
EditMenu->addAction(text);
// 在配置模式与编辑文件之间增加虚线
EditMenu->addSeparator();
QAction* option = new QAction(EditMenu);
option->setText("配置模式");
EditMenu->addAction(option);
// 1.1.2 定义Option配置模式下的子菜单
QMenu* childMenu = new QMenu();
QAction* set_file = new QAction(childMenu);
set_file->setText("设置文件内容");
set_file->setShortcut(Qt::CTRL | Qt::Key_B);
childMenu->addAction(set_file);
QAction* read_file = new QAction(childMenu);
read_file->setText("读取文件内容");
read_file->setShortcut(Qt::CTRL | Qt::Key_C);
childMenu->addAction(read_file);
// ----------------------------------------------------------
// 注册菜单到窗体中
// ----------------------------------------------------------
// 首先将childMenu注册到option中
option->setMenu(childMenu);
// 然后再将childMenu加入到EditMenu中
// EditMenu->addMenu(childMenu);
// ----------------------------------------------------------
// 绑定信号和槽
// ----------------------------------------------------------
connect(text, &QAction::triggered, this, [=]() {
QMessageBox::information(nullptr, "提示", "触发编辑文件", QMessageBox::Ok);
});
connect(set_file, &QAction::triggered, this, [=]() {
QMessageBox::information(nullptr, "提示", "触发设置文件", QMessageBox::Ok);
});
connect(read_file, &QAction::triggered, this, [=]() {
QMessageBox::information(nullptr, "提示", "触发读取文件", QMessageBox::Ok);
});
}
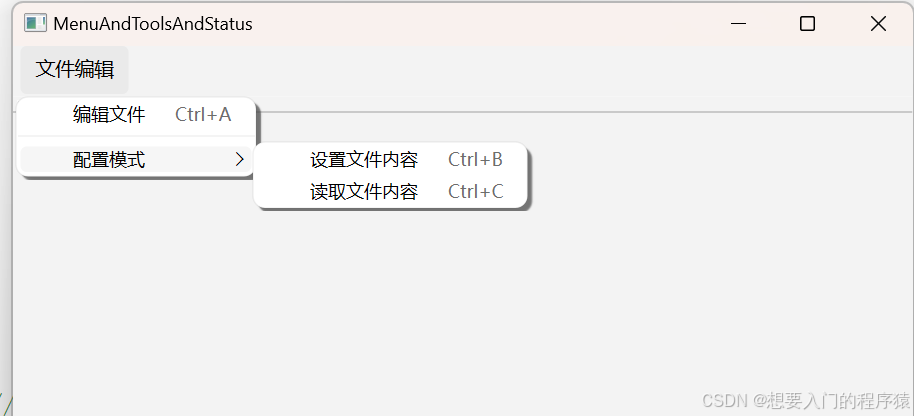
4.状态栏
void MenuAndToolsAndStatus::Init_StatusBar()
{
QStatusBar* statusbar = new QStatusBar();
setStatusBar(statusbar);
QLabel* url = new QLabel(this);
QLabel* about = new QLabel(this);
url->setFrameStyle(QFrame::Box | QFrame::Sunken);
url->setText(tr("<a href=\"https://www.baidu.com\">访问百度</a>"));
url->setOpenExternalLinks(true);
about->setFrameStyle(QFrame::Box | QFrame::Sunken);
about->setText(tr("<a href=\"https://www.taobao.com\">访问淘宝</a>"));
about->setOpenExternalLinks(true);
// 将信息增加到底部(永久添加)
statusbar->addPermanentWidget(url);
statusbar->addPermanentWidget(about);
// 隐藏状态栏下方三角形
statusbar->setSizeGripEnabled(false);
}
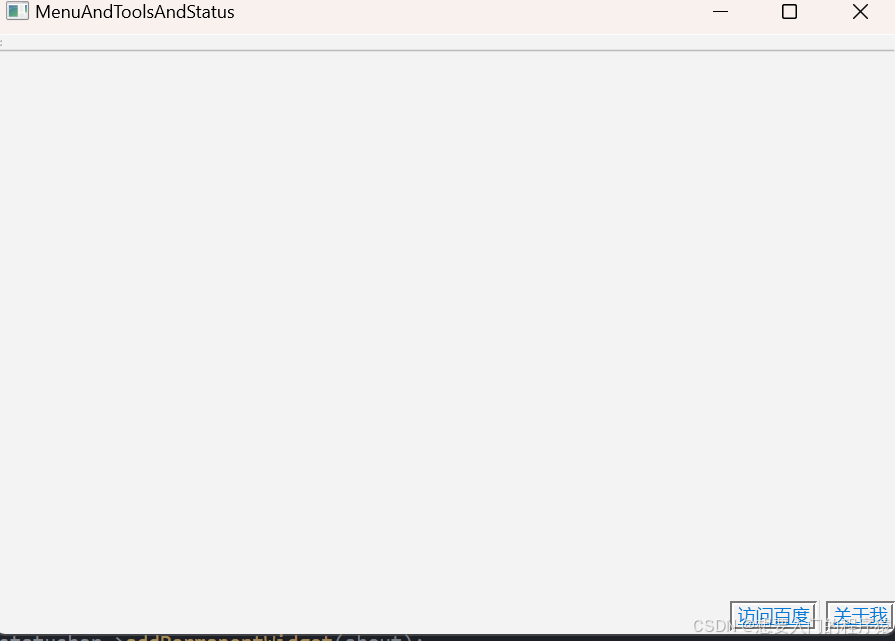