数据结构与算法之二叉树: LeetCode 700. 二叉搜索树中的搜索 (Ts版)
二叉搜索树中的搜索
- https://leetcode.cn/problems/search-in-a-binary-search-tree/
描述
- 给定二叉搜索树(BST)的根节点 root 和一个整数值 val
- 你需要在 BST 中找到节点值等于 val 的节点。 返回以该节点为根的子树
- 如果节点不存在,则返回 null
示例 1
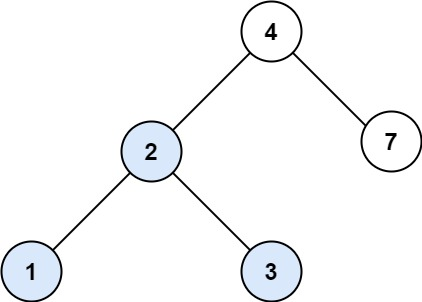
输入:root = [4,2,7,1,3], val = 2
输出:[2,1,3]
示例 2
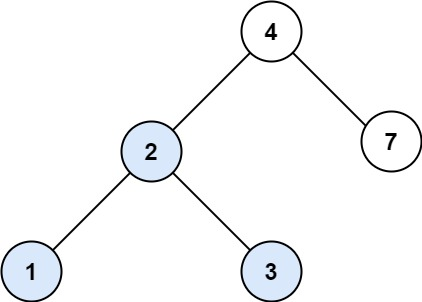
输入:root = [4,2,7,1,3], val = 5
输出:[]
提示
- 树中节点数在 [1, 5000] 范围内
- 1 <= Node.val <= 1 0 7 10^7 107
- root 是二叉搜索树
- 1 <= val <= 1 0 7 10^7 107
Typescript 版算法实现
1 ) 方案:递归
/**
* Definition for a binary tree node.
* class TreeNode {
* val: number
* left: TreeNode | null
* right: TreeNode | null
* constructor(val?: number, left?: TreeNode | null, right?: TreeNode | null) {
* this.val = (val===undefined ? 0 : val)
* this.left = (left===undefined ? null : left)
* this.right = (right===undefined ? null : right)
* }
* }
*/
function searchBST(root: TreeNode | null, val: number): TreeNode | null {
if (!root) return null;
if (val === root.val) return root;
return searchBST(val < root.val ? root.left : root.right, val);
};
2 ) 方案:迭代
/**
* Definition for a binary tree node.
* class TreeNode {
* val: number
* left: TreeNode | null
* right: TreeNode | null
* constructor(val?: number, left?: TreeNode | null, right?: TreeNode | null) {
* this.val = (val===undefined ? 0 : val)
* this.left = (left===undefined ? null : left)
* this.right = (right===undefined ? null : right)
* }
* }
*/
function searchBST(root: TreeNode | null, val: number): TreeNode | null {
while (root) {
if (val === root.val) return root;
root = val < root.val ? root.left : root.right;
}
return null;
};