<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JSON 格式化器</title>
<style>
body {
font-family: Arial, sans-serif;
background-color: #f4f4f9;
color: #333;
margin: 0;
padding: 0;
}
.container {
max-width: 900px;
margin: 50px auto;
padding: 20px;
background-color: #fff;
border-radius: 8px;
box-shadow: 0 2px 10px rgba(0, 0, 0, 0.1);
}
h1 {
text-align: center;
color: #007bff;
margin-bottom: 20px;
}
textarea {
width: 100%;
height: 300px;
padding: 10px;
font-size: 14px;
border: 1px solid #ddd;
border-radius: 4px;
box-sizing: border-box;
background-color: #f9f9f9;
color: #333;
resize: none;
}
button {
display: block;
width: 100%;
padding: 12px;
background-color: #007bff;
color: white;
border: none;
border-radius: 4px;
font-size: 16px;
cursor: pointer;
margin-top: 20px;
}
button:hover {
background-color: #0056b3;
}
pre {
background-color: #282c34;
color: #ffffff;
padding: 20px;
border-radius: 4px;
font-size: 14px;
overflow-x: auto;
white-space: pre-wrap;
word-wrap: break-word;
}
/* JSON 语法高亮 */
.string { color: #98c379; } /* 绿色 */
.number { color: #61afef; } /* 蓝色 */
.boolean { color: #c678dd; } /* 紫色 */
.null { color: #d19a66; } /* 橙色 */
.key { color: #e06c75; } /* 红色 */
</style>
</head>
<body>
<div class="container">
<h1>JSON 格式化器</h1>
<textarea id="jsonInput" placeholder="在此粘贴 JSON 字符串..."></textarea>
<button onclick="formatJson()">格式化 JSON</button>
<div id="error" class="error" style="color: red; margin-top: 10px;"></div>
<pre id="formattedJson"></pre>
</div>
<script>
function syntaxHighlight(json) {
if (typeof json !== 'string') {
json = JSON.stringify(json, null, 4);
}
json = json.replace(/&/g, "&").replace(/</g, "<").replace(/>/g, ">");
return json.replace(/("(\\u[\da-fA-F]{4}|\\[^u"]|[^\\"])*"(\s*:)?|\b(true|false|null)\b|-?\d+(\.\d+)?([eE][+-]?\d+)?)/g, function (match) {
let cls = "number";
if (/^"/.test(match)) {
if (/:$/.test(match)) {
cls = "key"; // 键名(红色)
} else {
cls = "string"; // 字符串(绿色)
}
} else if (/true|false/.test(match)) {
cls = "boolean"; // 布尔值(紫色)
} else if (/null/.test(match)) {
cls = "null"; // null(橙色)
}
return '<span class="' + cls + '">' + match + '</span>';
});
}
function formatJson() {
const input = document.getElementById('jsonInput').value;
const errorDiv = document.getElementById('error');
const formattedJsonDiv = document.getElementById('formattedJson');
errorDiv.textContent = ''; // 清空错误信息
formattedJsonDiv.innerHTML = ''; // 清空之前的格式化结果
try {
// 解析 JSON
const jsonObj = JSON.parse(input.trim());
// 语法高亮并渲染
formattedJsonDiv.innerHTML = syntaxHighlight(jsonObj);
} catch (error) {
errorDiv.textContent = 'JSON 解析失败,请检查格式是否正确。';
}
}
</script>
</body>
</html>
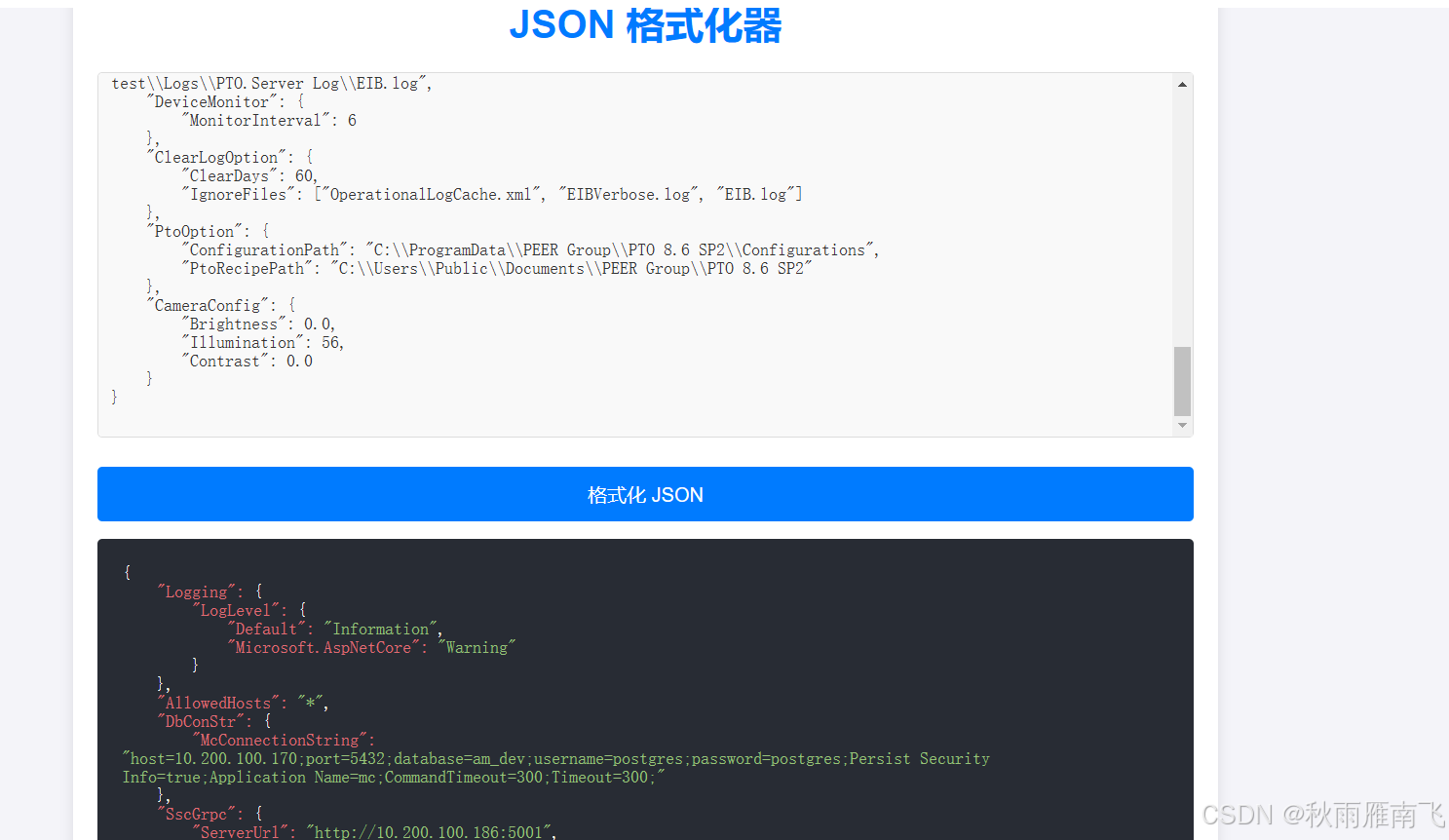