02.19 构造函数
1.思维导图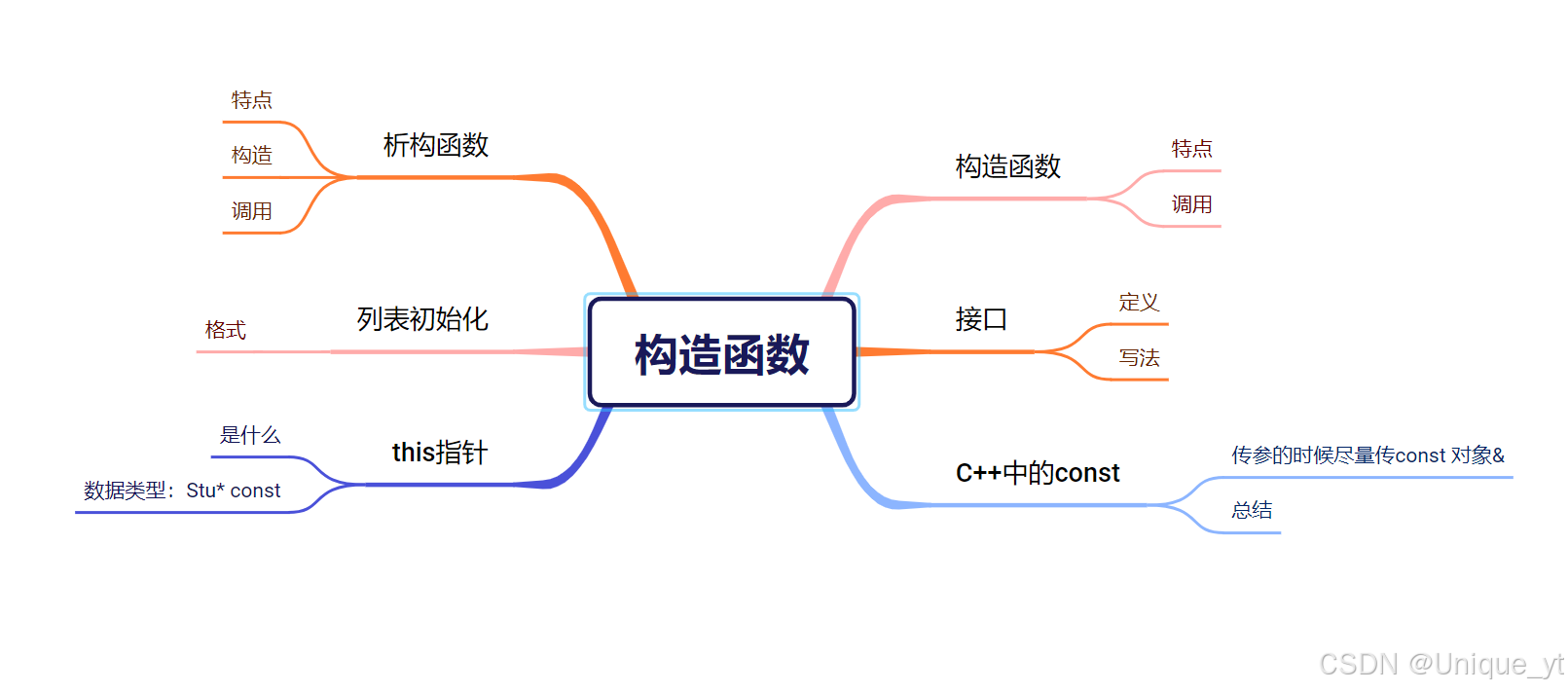
2.封装一个mystring类 ,
拥有私有成员: char* p
int len
需要让以下代码编译通过,并实现对应功能:
mystring str = "hello";
mystring ptr;
ptr.copy(str) ;
ptr.append(str);
ptr.show();输出ptr代表的字符串
ptr.compare(str) ;比较ptr和str是否一样
ptr.swap(str) ;交换ptr 和 str的内容
代码:
#include <iostream>
#include <cstring>
#include <cstdlib>
#include <unistd.h>
#include <sstream>
#include <vector>
#include <memory>
using namespace std;
class mystring{
private:
char* p;
int len;
public:
mystring();
mystring(const char* str);
~mystring();
void copy(const mystring& r);
void show();
void append(const mystring& r);
bool compare(const mystring& r);
void swap(mystring& r);
// 再写一个函数,要求实现将 mystrwing 转换成 const char*
const char* data();
};
mystring::mystring(){
p = NULL;
len = 0;
}
mystring::mystring(const char* str){
len = strlen(str);// 计算str实际长度
p = (char*)calloc(1,len+1);// 根据str的实际长度,申请对应大小的堆空间
strcpy(p,str);// 将str拷贝到堆空间里面去
}
mystring::~mystring(){
if(p != NULL){
free(p);
}
}
// 其实就是p的set接口
void mystring::copy(const mystring& r){
if(p != NULL){
free(p);
}
len = r.len;
p = (char*)calloc(1,len+1);
strcpy(p,r.p);
}
// 其实就是 p 的 get接口
const char* mystring::data(){
return p;
}
void mystring::show(){
cout << p << endl;
}
void mystring::append(const mystring& r){
len = len + r.len;
char* backup = p;
p = (char*)calloc(1,len+1);
strcpy(p,backup);
strcat(p,r.p);
free(backup);
}
bool mystring::compare(const mystring& r){
return strcmp(p,r.p) == 0;
}
void mystring::swap(mystring& r){
char* temp = p;
p = r.p;
r.p = temp;
}
int main(int argc,const char** argv){
mystring str = "hello";
printf("str = %s\n",str.data());
mystring ptr;
ptr.copy("你好");
ptr.show();
ptr.append("世界");
ptr.show();
//ptr.copy("hello");
if(ptr.compare(str)){
cout << "ptr 和 str 一样" << endl;
}else{
cout << "ptr 和 str 不一样" << endl;
}
ptr.swap(str);
ptr.show();
str.show();
}
3.封装一个 File 类,拥有私有成员 File* fp ,实现以下功能 File f = "文件名" 要求打开该文件; f.write(string str) 要求将str数据写入文件中; string str = f.read(int size) 从文件中读取最多size个字节,并将读取到的数据返回 ;析构函数
#include <iostream>
#include <cstring>
#include <cstdlib>
#include <unistd.h>
#include <sstream>
#include <vector>
#include <memory>
using namespace std;
class File {
private:
FILE* fp; // 文件指针
public:
// 构造函数,打开文件
File(const char* filename) {
fp = fopen(filename, "r+"); // 以读写模式打开文件
if (!fp) {
fp = fopen(filename, "w+"); // 如果文件不存在,创建并打开
if (!fp) {
cout << "文件打开失败" << endl;
exit(1);
}
}
}
// 析构函数,关闭文件
~File() {
if (fp) {
fclose(fp); // 关闭文件
}
}
// 写入数据到文件
void write(const char* str) {
if (fp) {
fputs(str, fp); // 将字符串写入文件
fflush(fp); // 刷新缓冲区,确保数据写入文件
} else {
cout << "要写入内容的文件未打开" << endl;
}
}
// 从文件中读取数据
string read(int size) {
string result;
if (fp) {
char* buffer = (char*)calloc(1, size + 1); // 使用 calloc 动态分配缓冲区
if (!buffer) {
cout << "内存分配失败" << endl;
return result;
}
fseek(fp, 0, SEEK_SET); // 将文件指针移动到文件开头
size_t bytesRead = fread(buffer, 1, size, fp);
buffer[bytesRead] = '\0';
result = buffer;
free(buffer); // 释放动态分配的内存
} else {
cout << "文件未打开以供读取" << endl;
}
return result;
}
};
int main() {
File f("test.txt");
// 写入数据
f.write("Hello, World!\n");
// 读取数据
string content = f.read(100);
cout << "从文件中读取到的信息为:" << content << endl;
return 0;
}
4.封装一个 Mutex 互斥锁类 要求: 构造函数:初始化互斥锁,并选择互斥锁的种类 lock 上锁互斥锁 unlock 解锁互斥锁 析构函数,销毁互斥锁 并且开启一个线程测试该互斥锁
#include <iostream>
#include <cstring>
#include <cstdlib>
#include <unistd.h>
#include <sstream>
#include <vector>
#include <memory>
#include <mutex>
#include <thread>
#include <pthread.h>
using namespace std;
class Mutex {
private:
pthread_mutex_t mutex; // 互斥锁
public:
// 构造函数,初始化互斥锁
Mutex(int type = PTHREAD_MUTEX_ERRORCHECK_NP) { //错误检查锁
pthread_mutexattr_t attr;
pthread_mutexattr_init(&attr); // 初始化互斥锁属性
pthread_mutexattr_settype(&attr, type); // 设置互斥锁类型
pthread_mutex_init(&mutex, &attr); // 初始化互斥锁
pthread_mutexattr_destroy(&attr); // 销毁互斥锁属性
}
// 析构函数,销毁互斥锁
~Mutex() {
pthread_mutex_destroy(&mutex); // 销毁互斥锁
}
// 上锁互斥锁
void lock() {
pthread_mutex_lock(&mutex); // 上锁
}
// 解锁互斥锁
void unlock() {
pthread_mutex_unlock(&mutex); // 解锁
}
};
// 全局变量,用于线程测试
Mutex test_mutex;
Mutex test_mutex1;
//test_mutex1.lock();
// 1#线程函数
void* thread1_func(void* arg) {
while(1) {
test_mutex1.lock(); // 上锁
cout << "1#线程" << endl;
sleep(1);
test_mutex.unlock(); // 解锁
}
return NULL;
}
int main() {
pthread_t thread1;
test_mutex1.lock();
// 创建一个线程
if (pthread_create(&thread1, 0, thread1_func, 0) != 0) {
cerr << "线程1创建失败" << endl;
return 1;
}
//主线程
while(1) {
test_mutex.lock(); // 上锁
cout << "主线程" << endl;
sleep(1);
test_mutex1.unlock();
}
pthread_join(thread1,NULL);
return 0;
}