【Python】如何解决Jupyter Notebook修改外部模块后必须重启内核的问题?
“为什么我修改了Python模块的代码,Jupyter Notebook却看不到变化?”
一、问题现象:令人抓狂的开发体验
假设你正在开发一个图像处理项目,项目结构如下:
project/
├── utils/
│ └── image_processor.py
└── EDA.ipynb
当你在Notebook中运行:
from utils.image_processor import enhance_image
enhanced = enhance_image(raw_image)
然后突然发现image_processor.py
中的算法需要优化。修改保存文件后:
• ❌ 重新运行enhance_image(raw_image)
看不到任何变化
• ❌ 重新导入模块提示No module named...
• ❌ 只有重启内核才能生效
这不仅打断工作流,还会丢失当前内存中的所有变量!
二、技术原理:理解Jupyter的模块加载机制
2.1 Python的模块缓存机制
• 首次导入模块时,Python会:
- 编译
.py
为.pyc
字节码 - 将模块对象存入
sys.modules
• 后续导入直接从缓存读取
2.2 Jupyter的特殊性
• 内核进程长期存活
• 单元格执行相当于在__main__
作用域运行
• 经典的重载方式:
import importlib
importlib.reload(module) # 单一模块重载
三、终极解决方案:autoreload扩展
3.1 基础配置
在Notebook的第一个单元格添加:
%load_ext autoreload
%autoreload 2 # 全量重载模式
此时:
• ✅ 修改image_processor.py
后直接重新运行单元格即可生效
• ✅ 保持所有变量状态
• ✅ 自动处理嵌套依赖
3.2 模式对比
模式 | 作用范围 | 内存开销 | 适用场景 |
---|---|---|---|
autoreload 0 | 禁用 | 0% | 生产环境 |
autoreload 1 | 仅import 的模块 | 30% | 常规开发 |
autoreload 2 | 所有模块 | 100% | 深度调试 |
简单demo
a.py
b=8
1.ipynb
可以完成即时更新
四、高级技巧:应对复杂场景
4.1 处理C扩展模块
对于.so
/.pyd
文件,建立热重载监听:
import watchdog.observers
class CExtensionReloader:
def __init__(self, module):
self.module = module
self.observer = watchdog.observers.Observer()
self.observer.schedule(
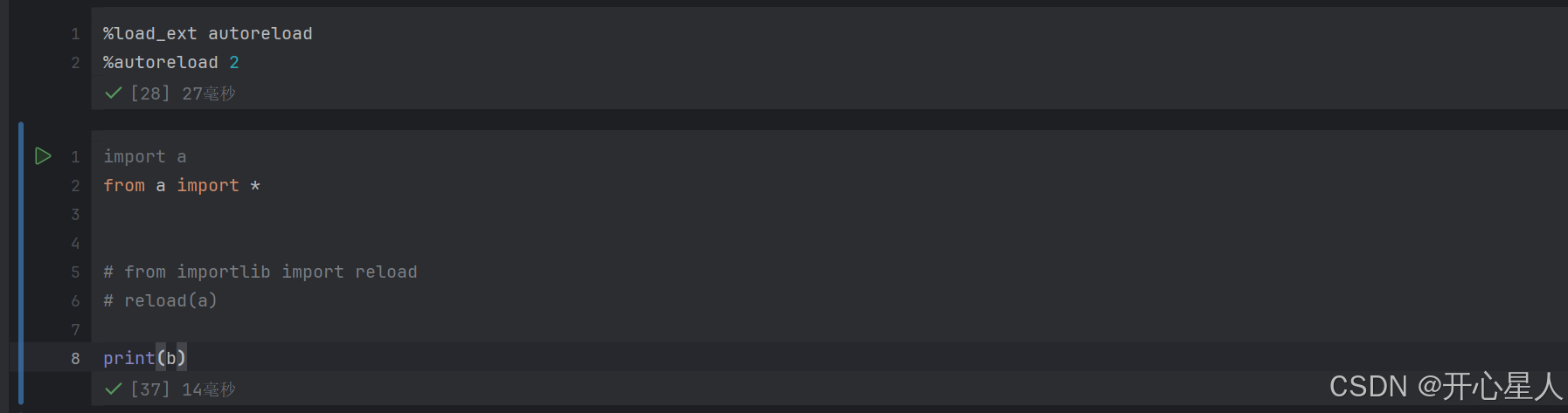
FileSystemEventHandler(
on_modified=lambda _: self._reload()),
path=os.path.dirname(module.__file__)
)
self.observer.start()
4.2 与调试器结合使用
在VSCode中配置launch.json
:
{
"configurations": [
{
"name": "Jupyter with Autoreload",
"type": "python",
"request": "launch",
"module": "notebook",
"args": [
"--NotebookApp.allow_origin='*'",
"--NotebookApp.disable_check_xsrf=True"
],
"env": {
"AUTORELOAD_MODE": "2"
}
}
]
}
4.3 性能优化方案
通过JupyterLab插件实现选择性重载:
# 安装扩展
jupyter labextension install @jupyterlab/hot-reload
# 配置热重载策略
{
"watchPatterns": [
"src/**/*.{py,js,css}",
"!node_modules/**"
],
"debounceDelay": 1000
}
五、最佳实践指南
5.1 项目级配置
在ipython_config.py
中设置:
c.InteractiveShellApp.exec_lines = [
'print("🚀 Autoreload Enabled!")',
'%autoreload 2'
]
c.InteractiveShellApp.extensions = [
'autoreload'
]
5.2 安全重载检查清单
- 检查模块是否包含
__main__
判断 - 避免在模块级初始化全局状态
- 使用版本标识符验证重载:
__version__ = "1.0.20231001"
5.3 监控重载事件
from IPython import get_ipython
def log_reload(module):
print(f"🔁 Reloaded {module.__name__}")
ip = get_ipython()
ip.events.register('post_execute', log_reload)
六、典型问题排查
6.1 修改不生效的检查步骤
- 确认文件保存路径正确
- 检查文件系统时间戳
- 查看模块缓存版本:
import utils.image_processor print(utils.image_processor.__cached__)
6.2 循环导入问题
使用importlib
的延迟导入:
def safe_import():
global expensive_module
if 'expensive_module' not in globals():
import expensive_module
6.3 副作用管理
通过上下文管理器隔离状态:
class ModuleReloadContext:
def __enter__(self):
self._modules = dict(sys.modules)
def __exit__(self, *args):
for name in set(sys.modules) - set(self._modules):
del sys.modules[name]