Qt5.12实战之控件设计
QPushButton使用
静态创建,通过设计窗口拖放控件:
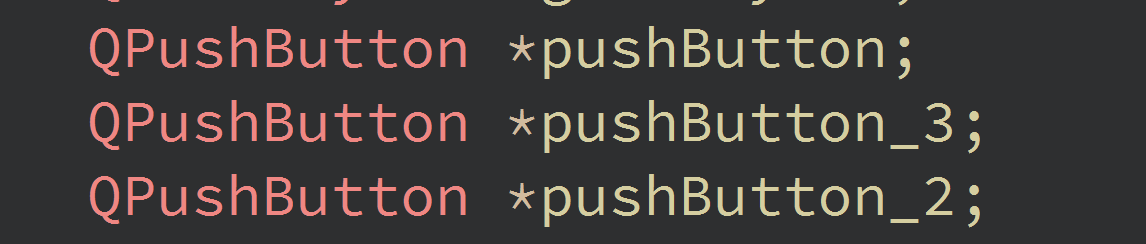
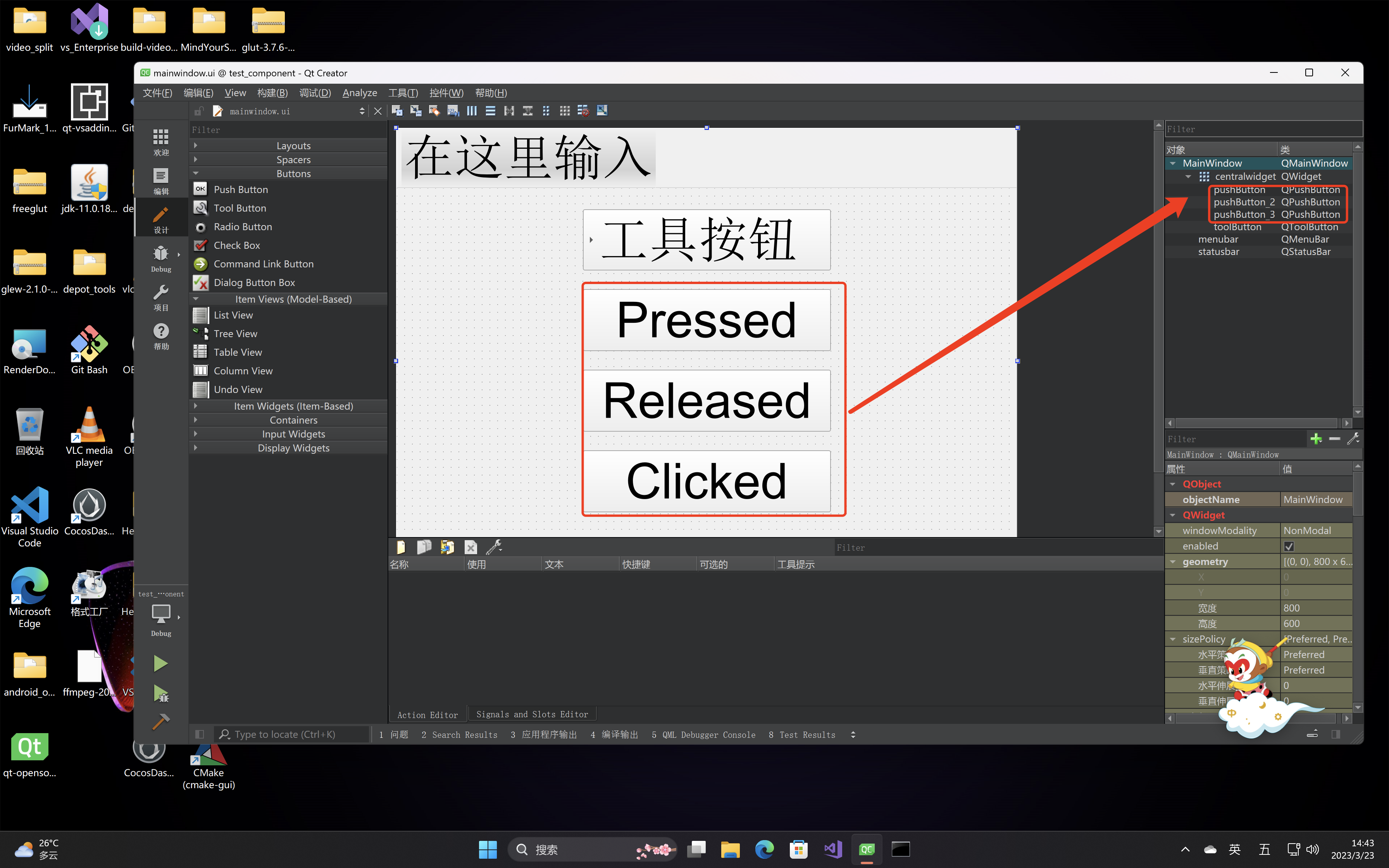
添加信号处理:
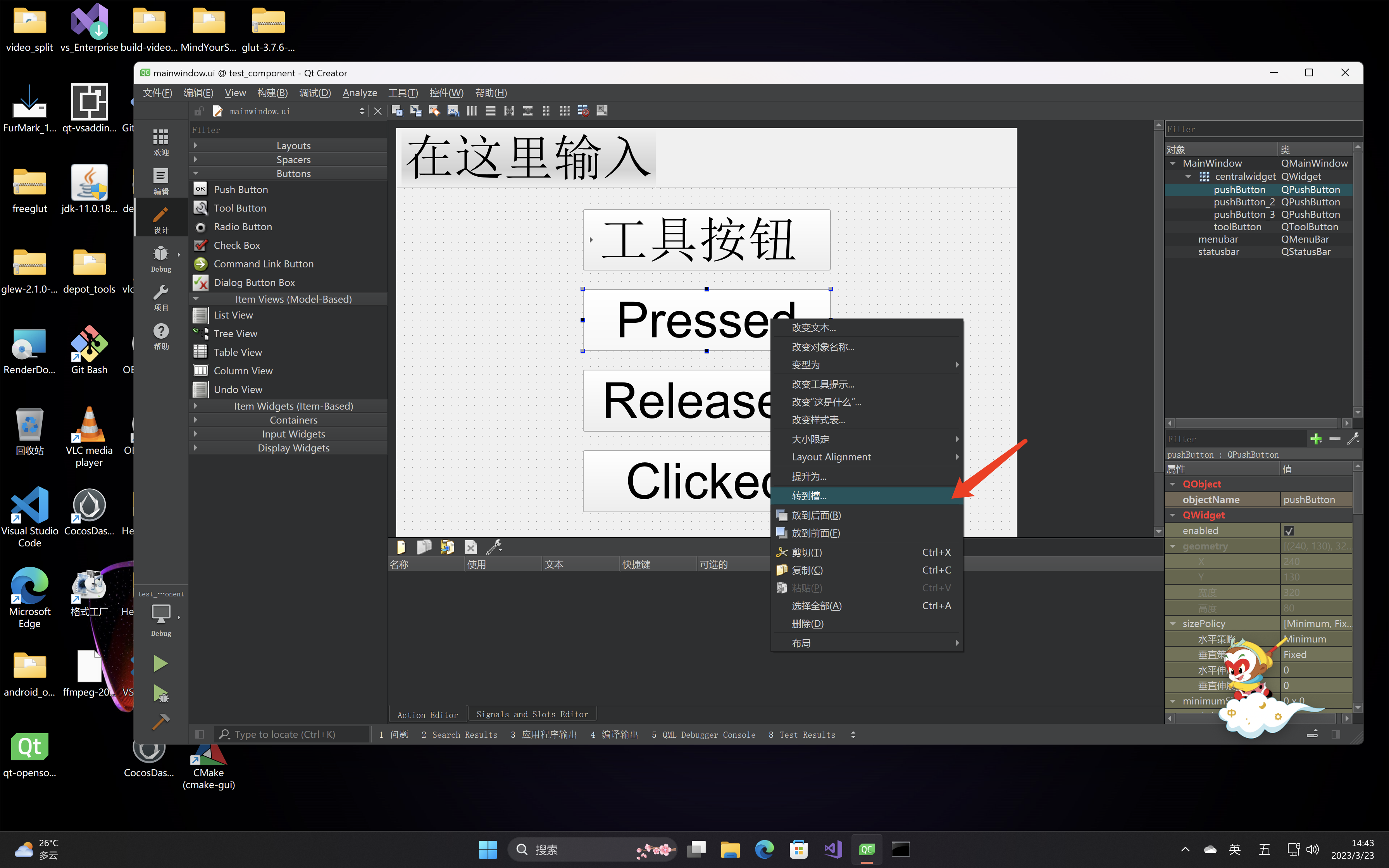
为Pressed,Released,Clicked三个按钮添加信号
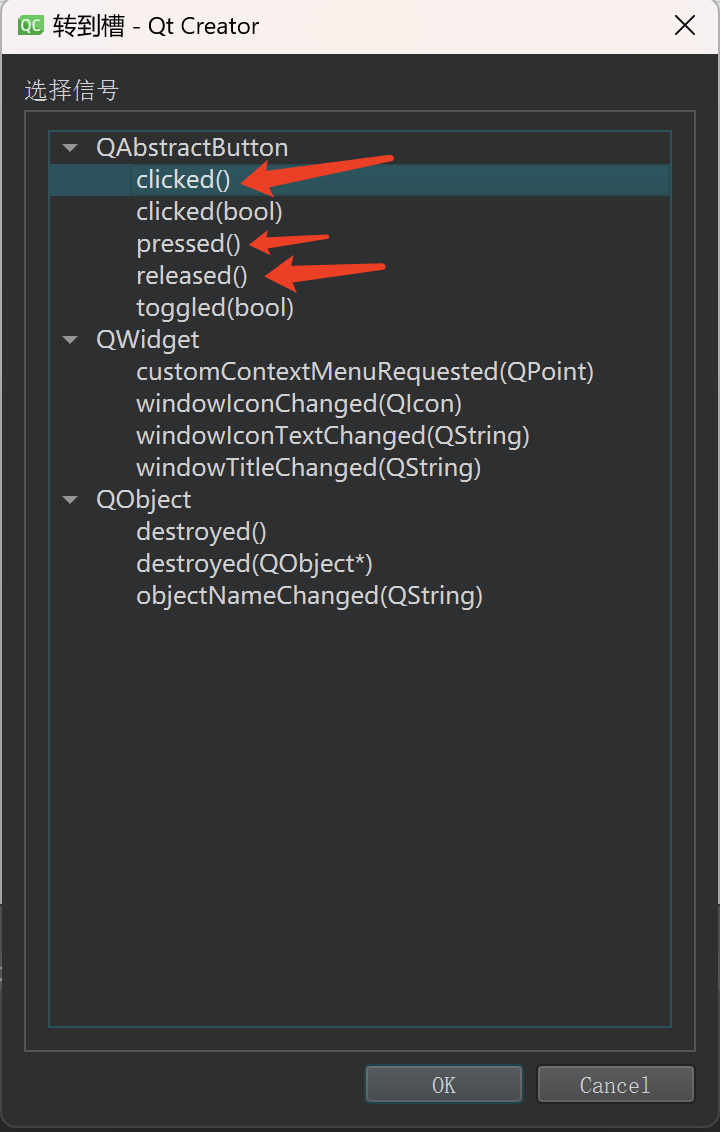
自动添加的信号槽:
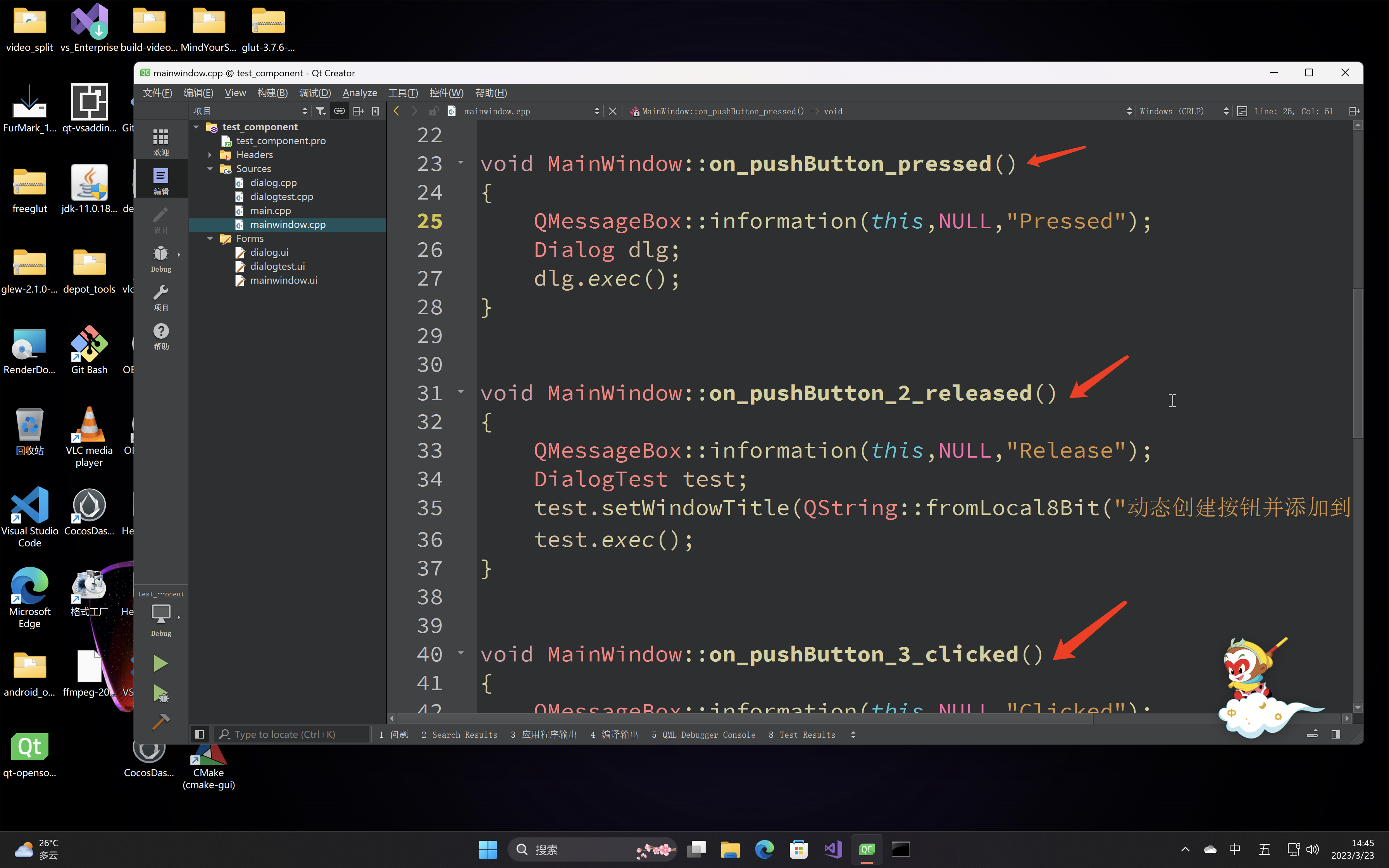
动态生成的槽定义:
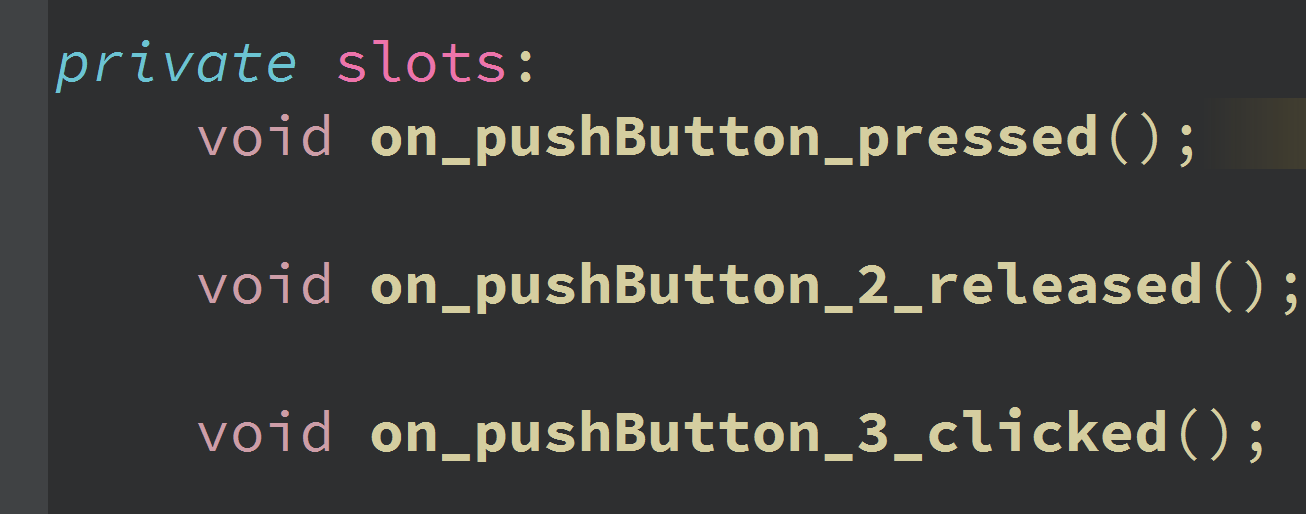
动态创建创建QPushButton
QPushButton *btn = new QPushButton(QString::fromLocal8Bit("动态添加的按钮"),this);
connect(btn,SIGNAL(clicked()),this,SLOT(on_cust_dynbtn()));
ui->gridLayout->addWidget(btn, 4, 0, 1, 1);
动态创建QToolButton
QToolButton *tool = new QToolButton(this);
tool->setArrowType(Qt::LeftArrow);
tool->setText(QString::fromLocal8Bit("自动创建的工具按钮"));
tool->setToolButtonStyle(Qt::ToolButtonTextUnderIcon);
connect(tool,SIGNAL(clicked()),this,SLOT(on_cust_tool_clicked()));
ui->gridLayout->addWidget(tool,5,0,1,1);
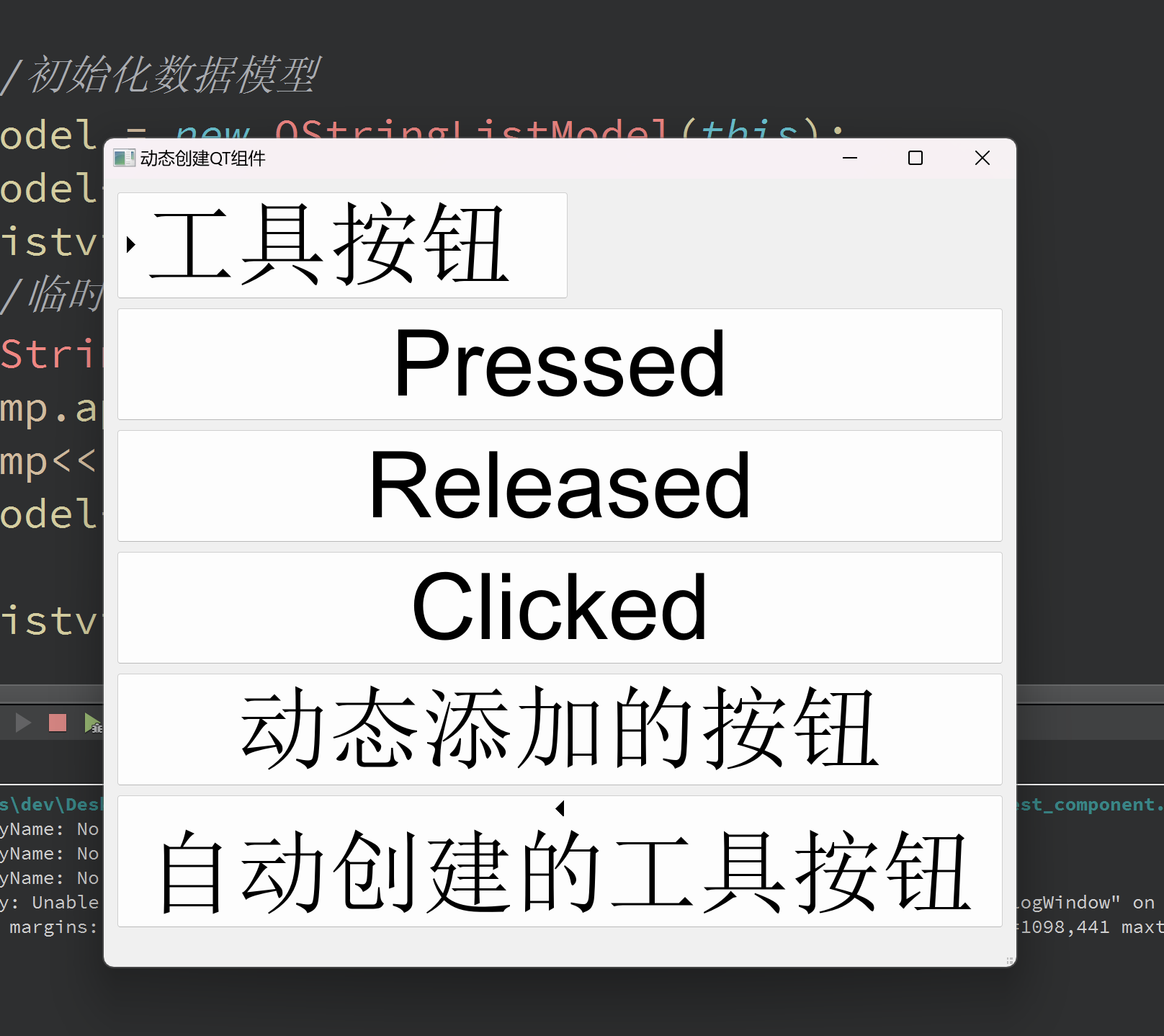
QRadioButton使用:
先在设计窗口调整好控件布局
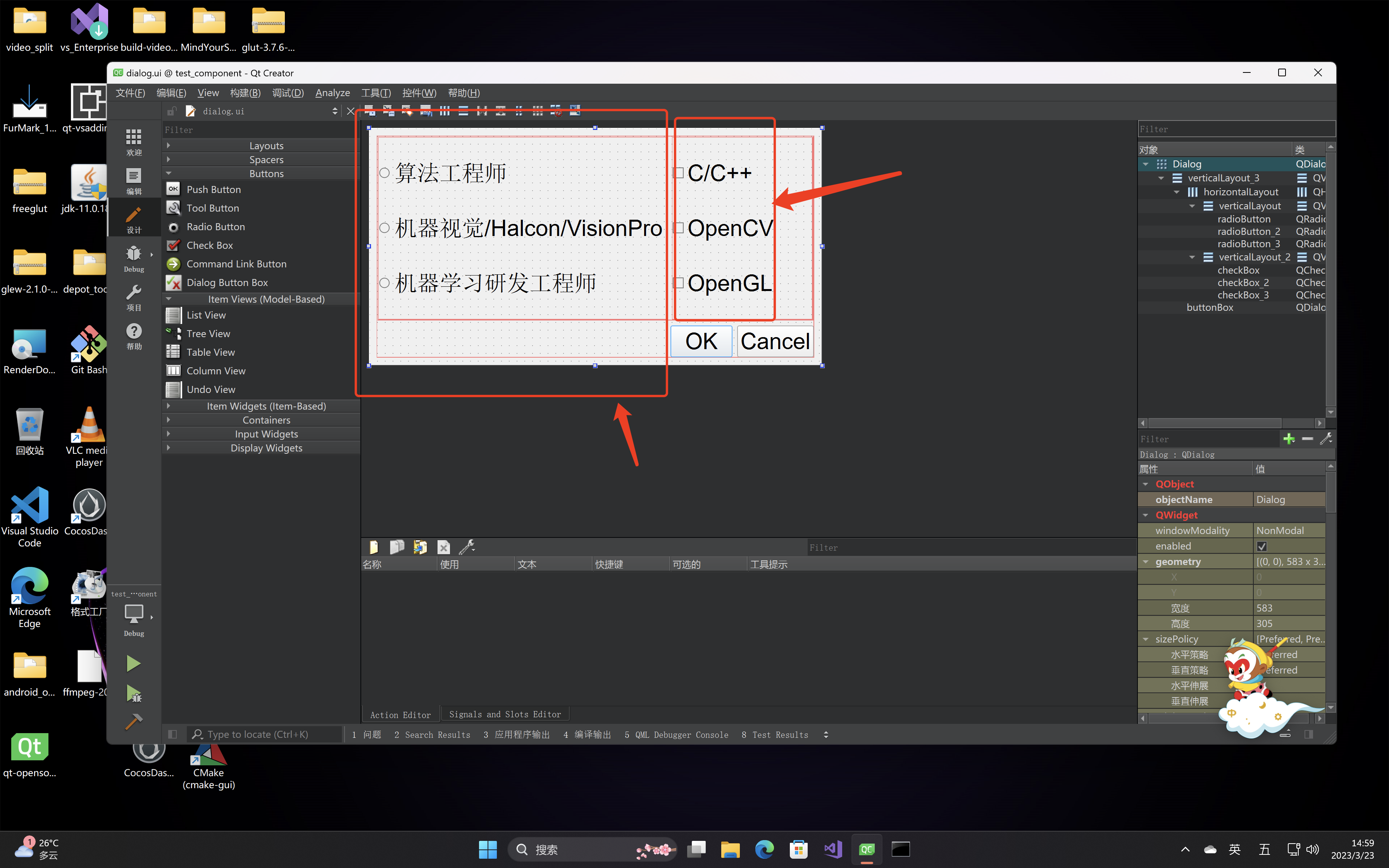
使用代码添加到按钮组:
//按钮组
group = new QButtonGroup(this);
group->addButton(ui->radioButton,0);
group->addButton(ui->radioButton_2,1);
group->addButton(ui->radioButton_3,2);
ui->radioButton->setChecked(true);//默认项
对按钮组中的控件进行信号连接
//信号连接
connect(ui->radioButton,SIGNAL(clicked(bool)),this,SLOT(on_radiobtn_checked(bool)));
connect(ui->radioButton_2,SIGNAL(clicked(bool)),this,SLOT(on_radiobtn_checked(bool)));
connect(ui->radioButton_3,SIGNAL(clicked(bool)),this,SLOT(on_radiobtn_checked(bool)));
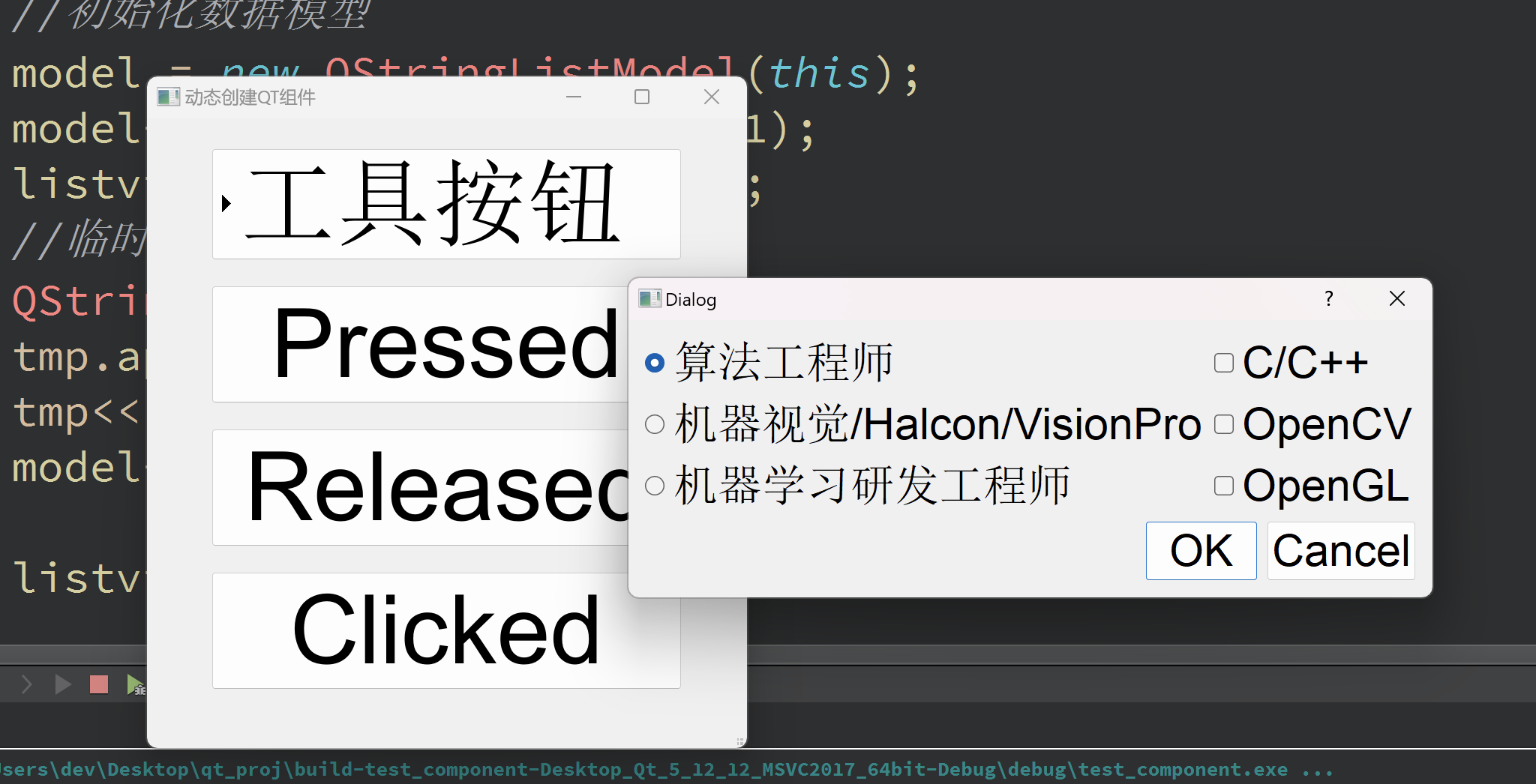
QCheckBox使用:
动态创建:
strList1<<"One"<<"Two"<<"Three";
//指向QCheckBox的指针
QCheckBox *exclusive[3];
//创建按钮组
group = new QButtonGroup(this);
//动态创建QCheckBox并添加到按钮组
for (int i=0;i<strList1.size() ;i++ ) {
exclusive[i] = new QCheckBox(strList1[i],this);
//设置QCheckBox几何位置与控件大小
//没有添加布局层的可用setGeometry
//添加了布局层的只能用addWidget
exclusive[i]->setGeometry(50,50+i*50,200,30);
//信号连接
connect(exclusive[i],SIGNAL(stateChanged(int)),this,SLOT(on_stateChanged(int)));
group->addButton(exclusive[i],i);
}
group->setExclusive(true);//true:单选 false:复选
QListView使用:
//初始化数据模型
model = new QStringListModel(this);
model->setStringList(strList1);
listview=new QListView(this);
//临时更新数据集
QStringList tmp = model->stringList();
tmp.append("Four");
tmp<<"Fire"<<"Six";
model->setStringList(tmp);
listview->setModel(model);
listview->setGeometry(50,200,300,300);
listview->model()->removeRow(model->rowCount()-1);
listview->model()->removeRows(3,2);
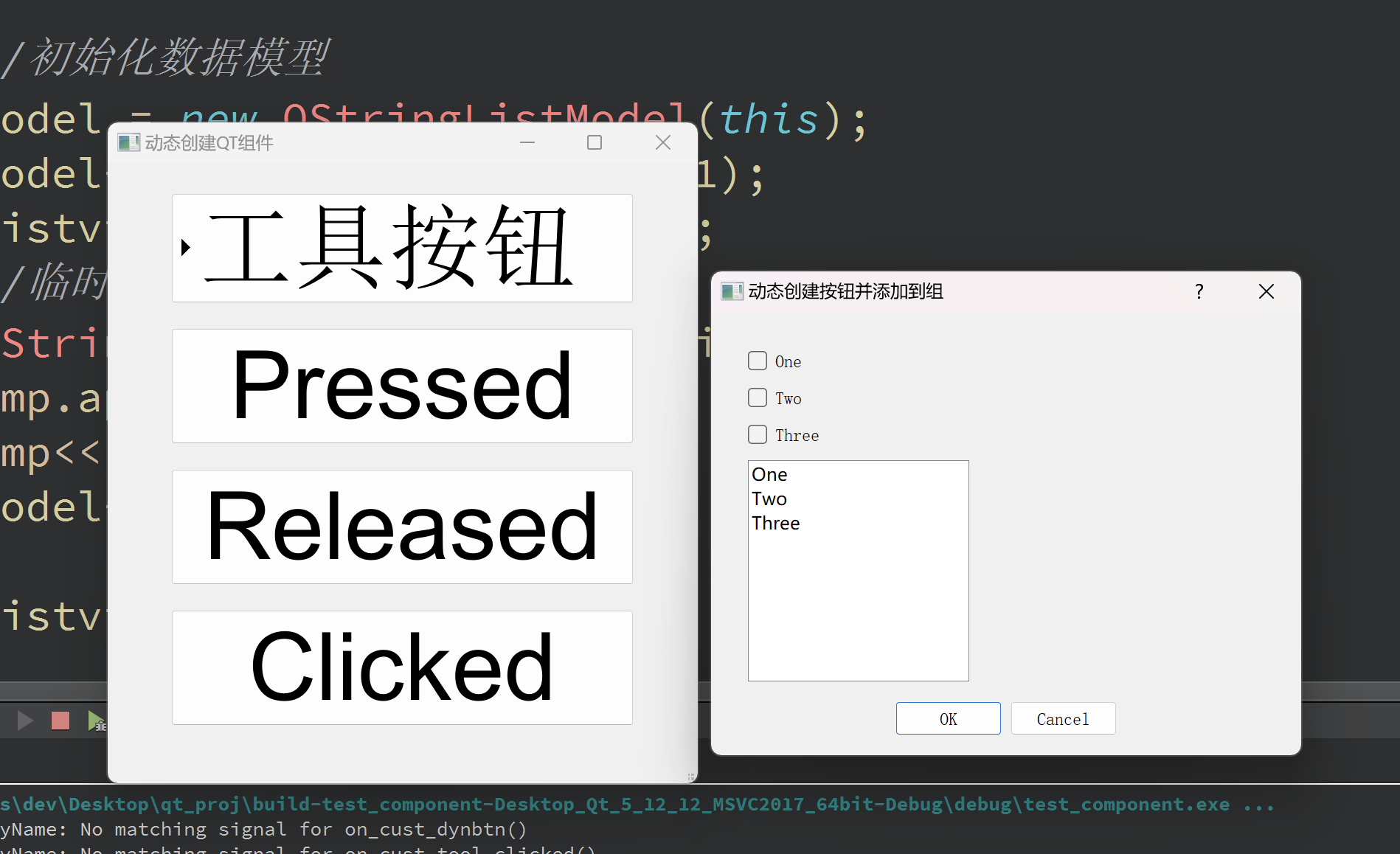