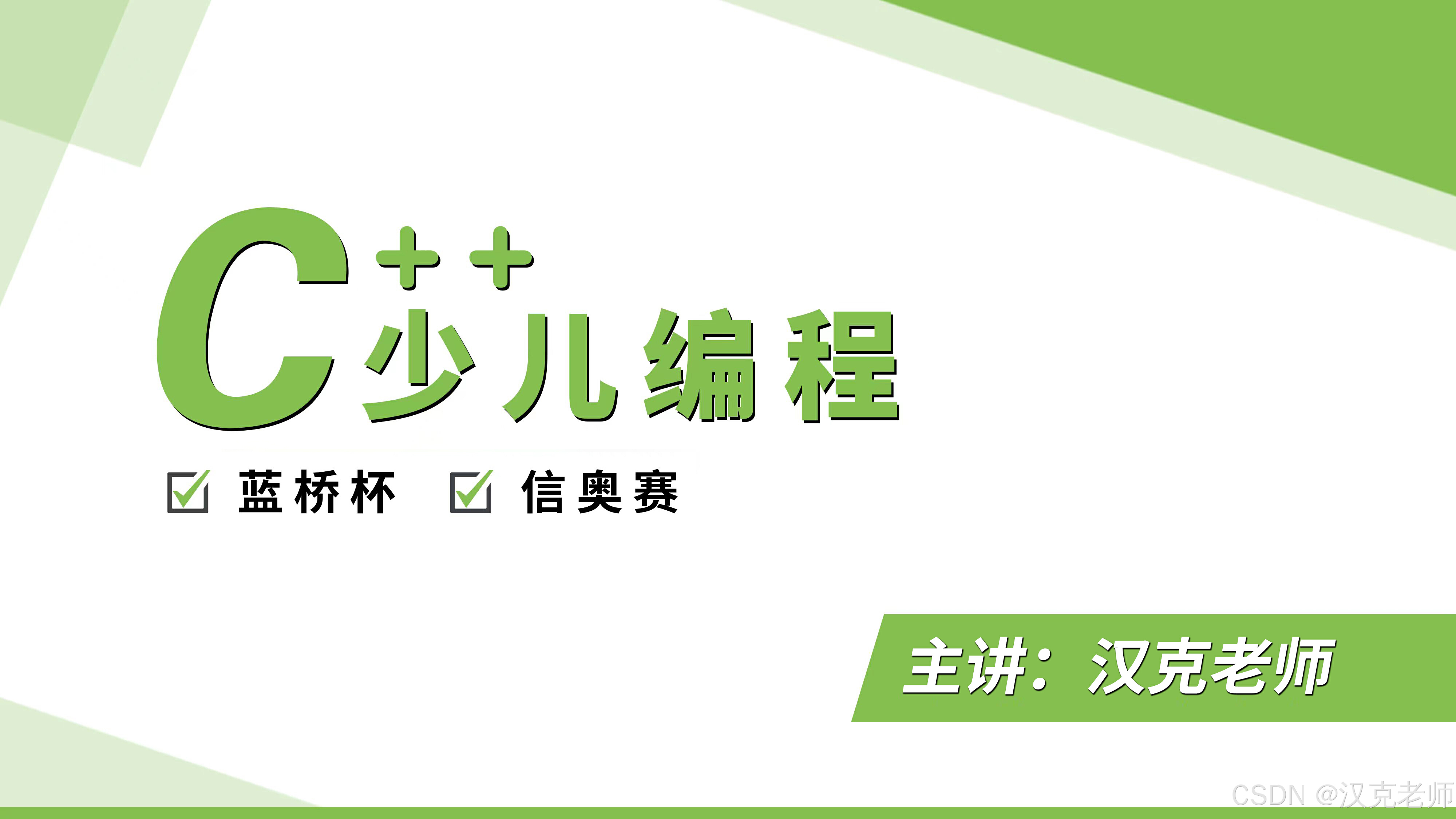
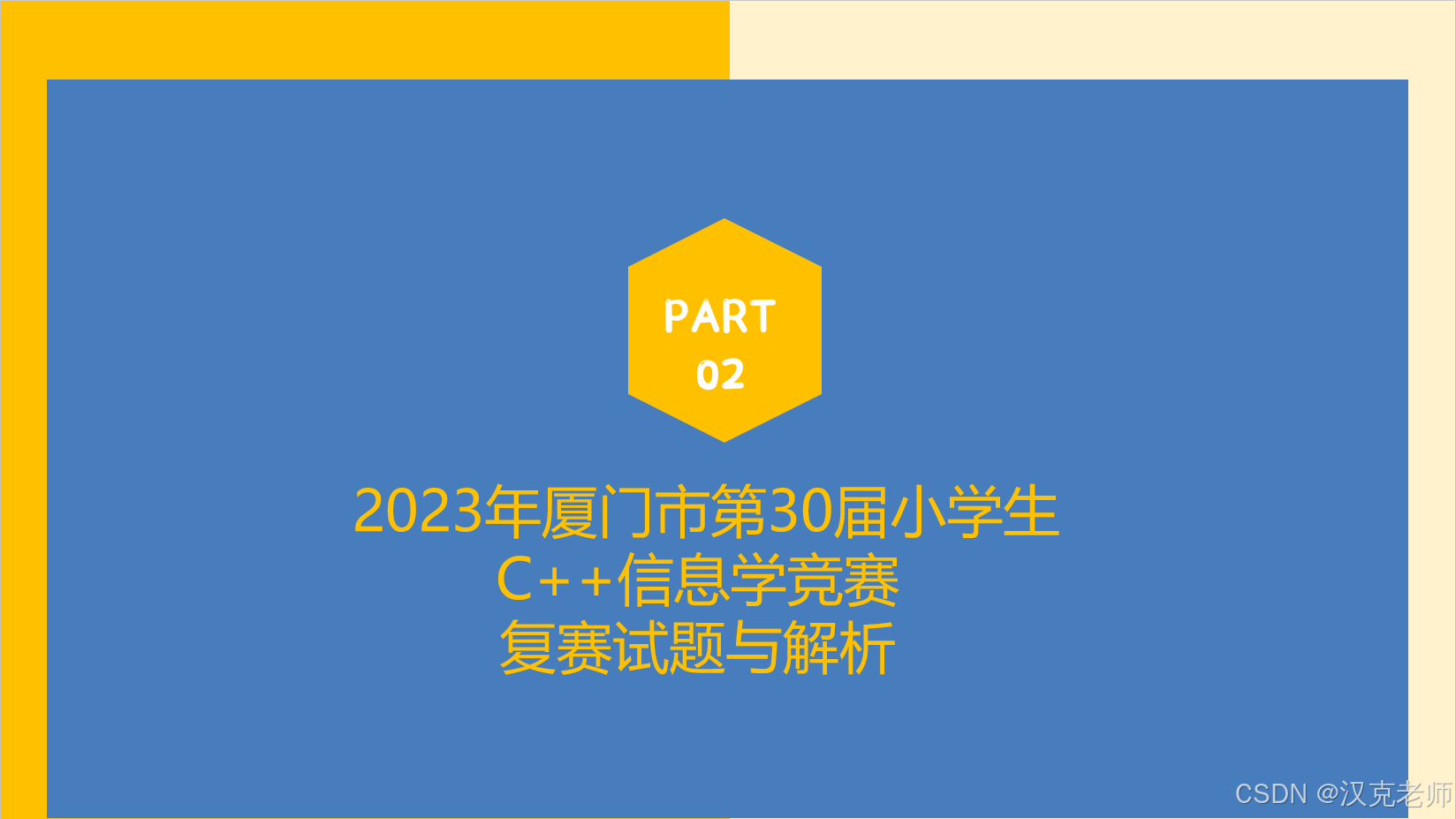
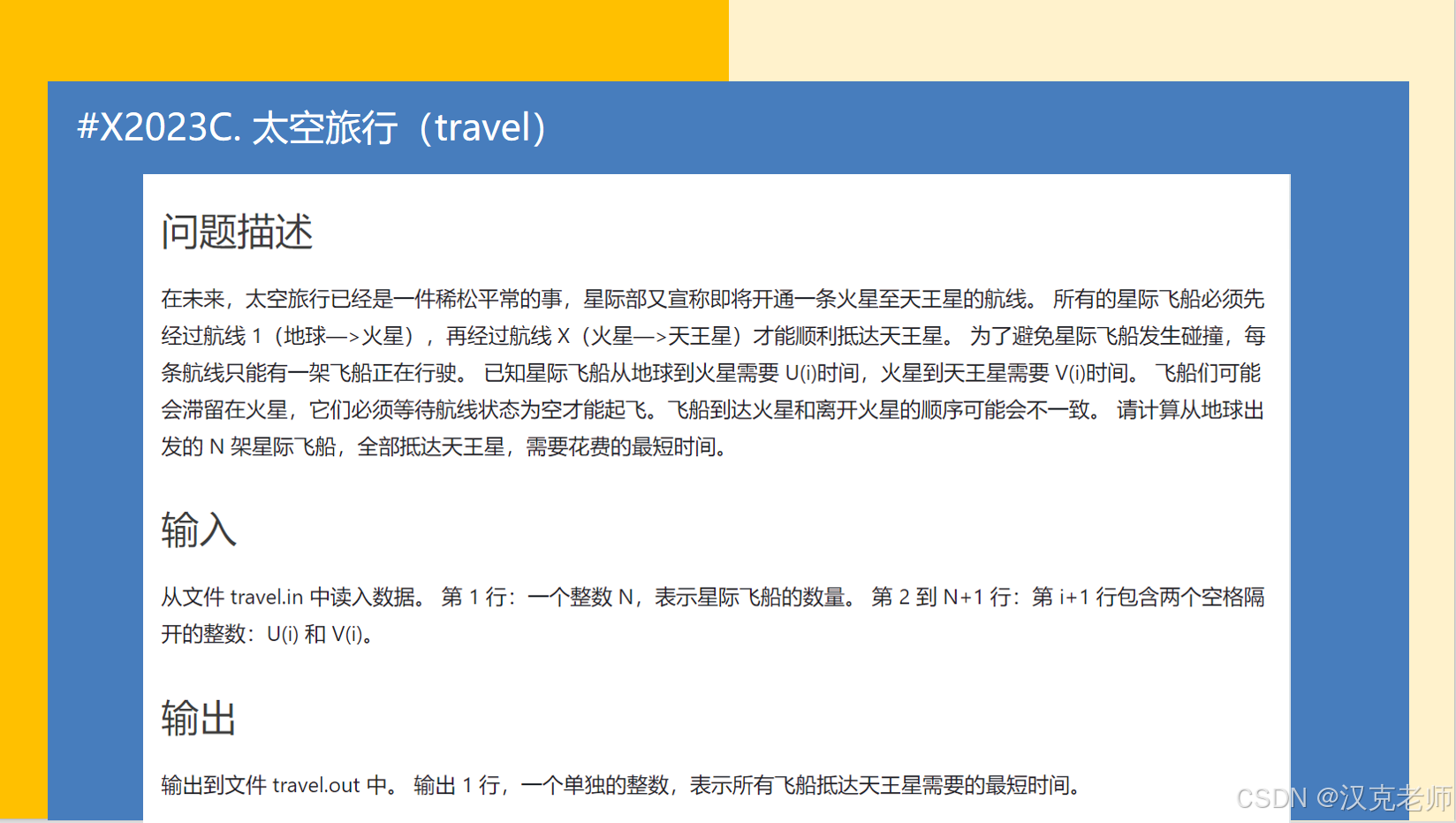
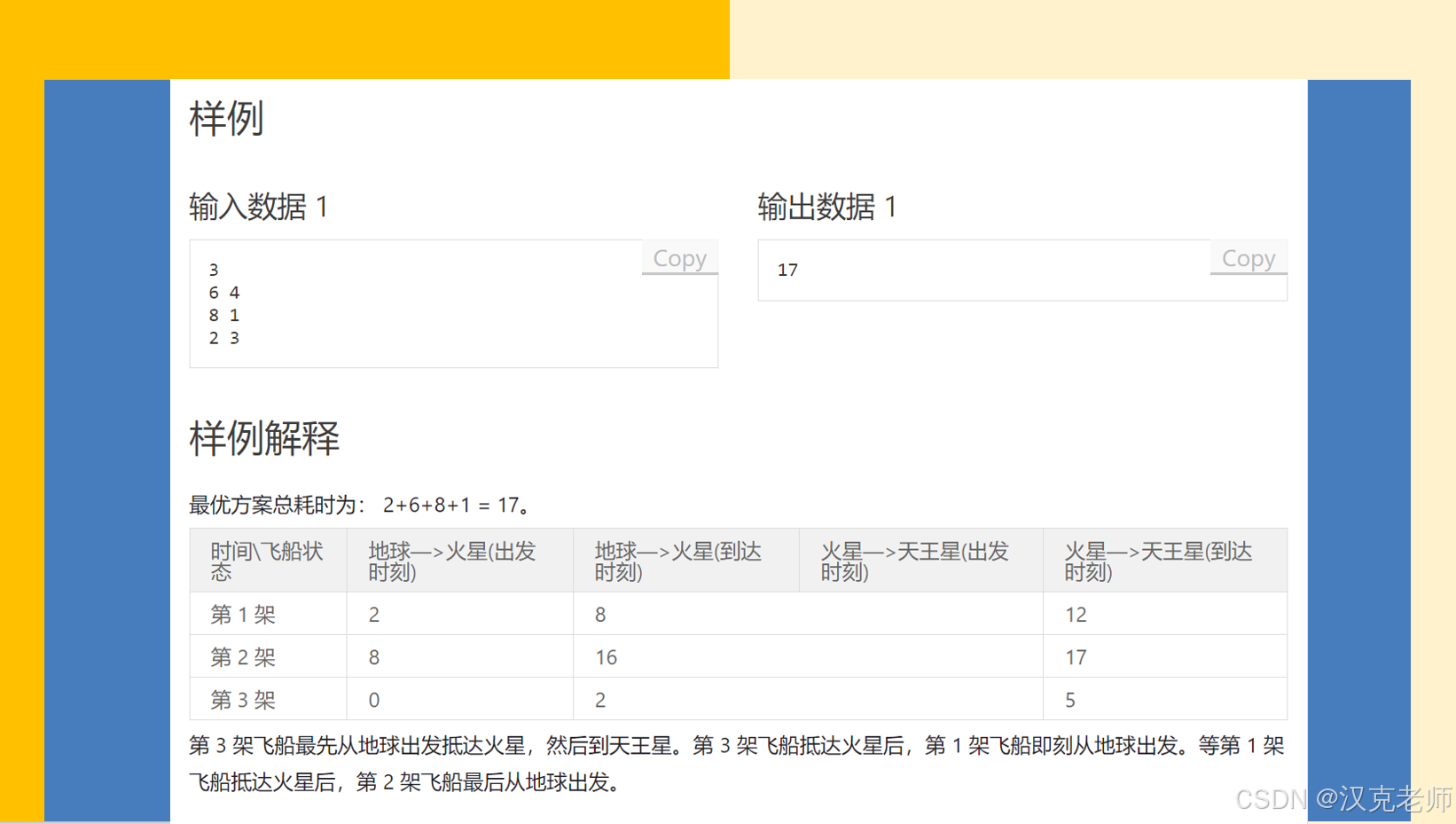
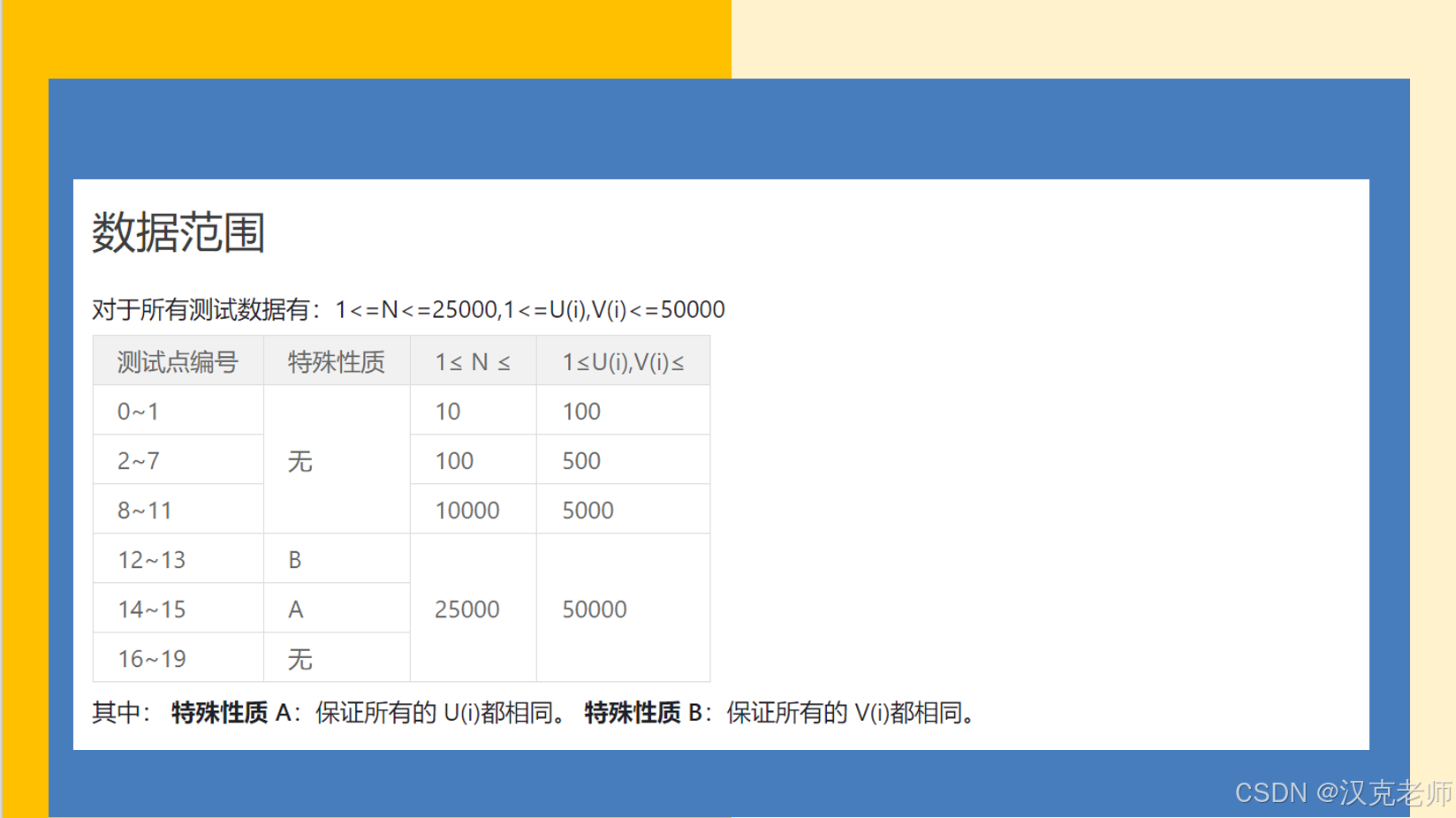
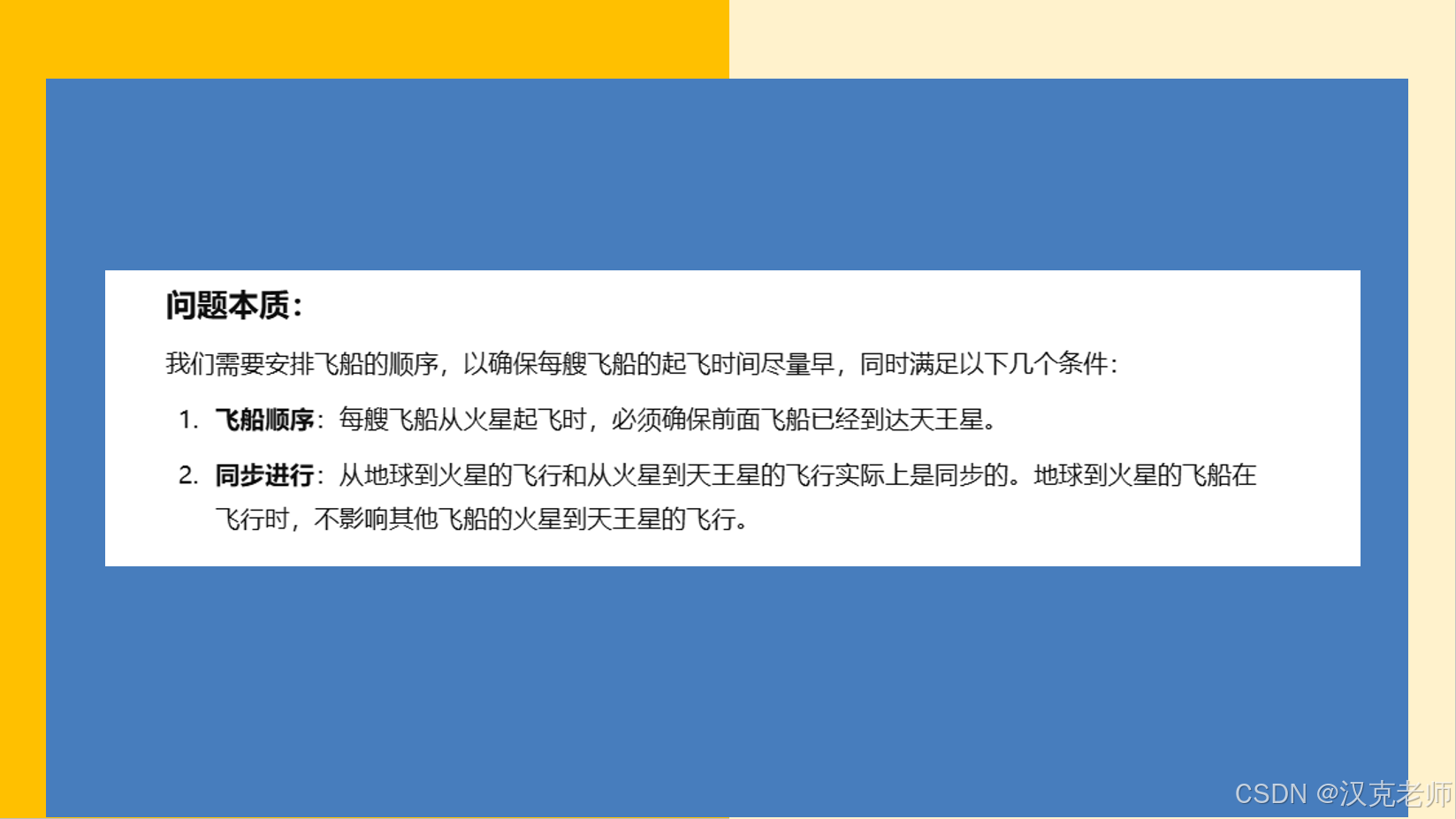
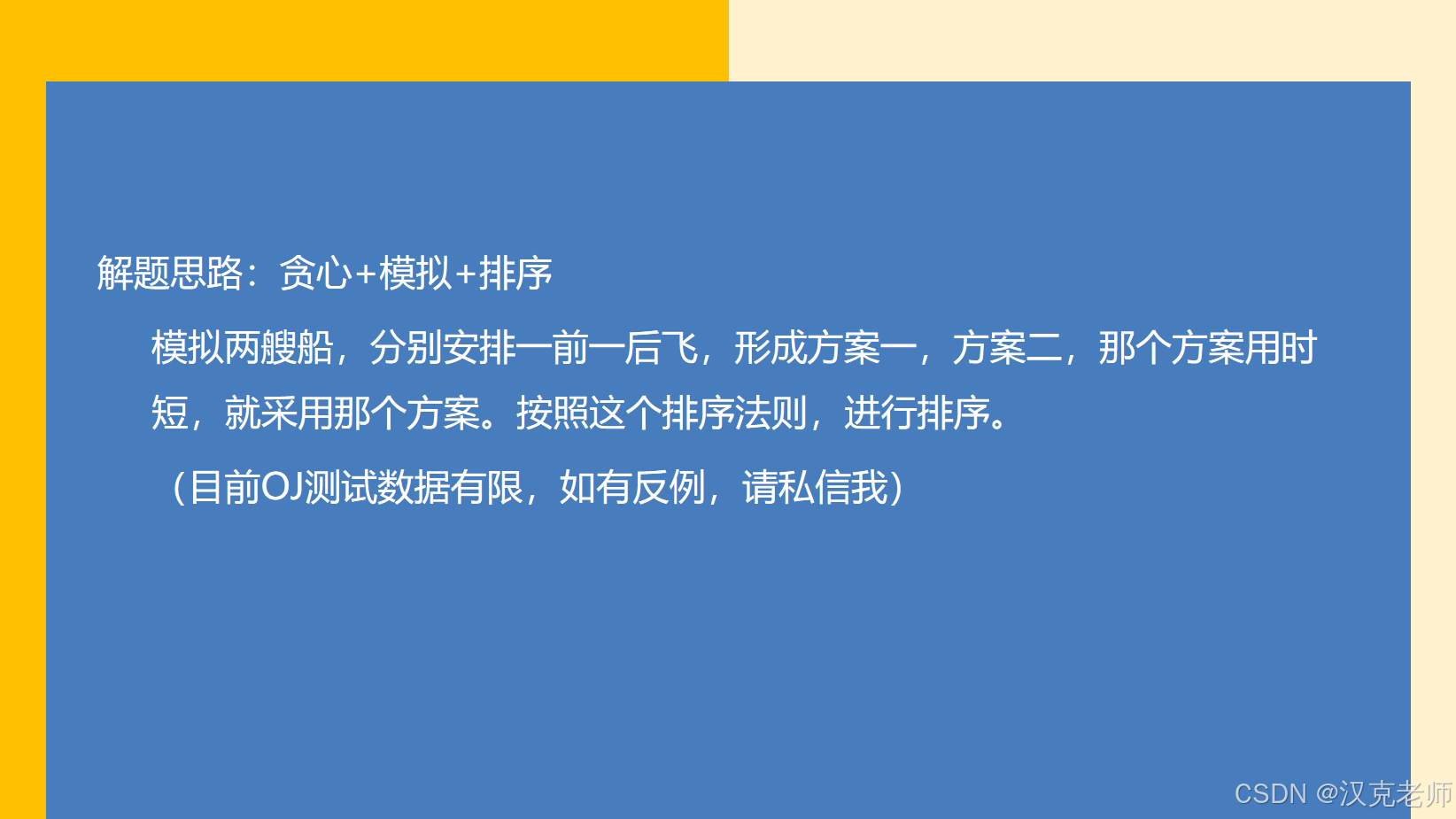
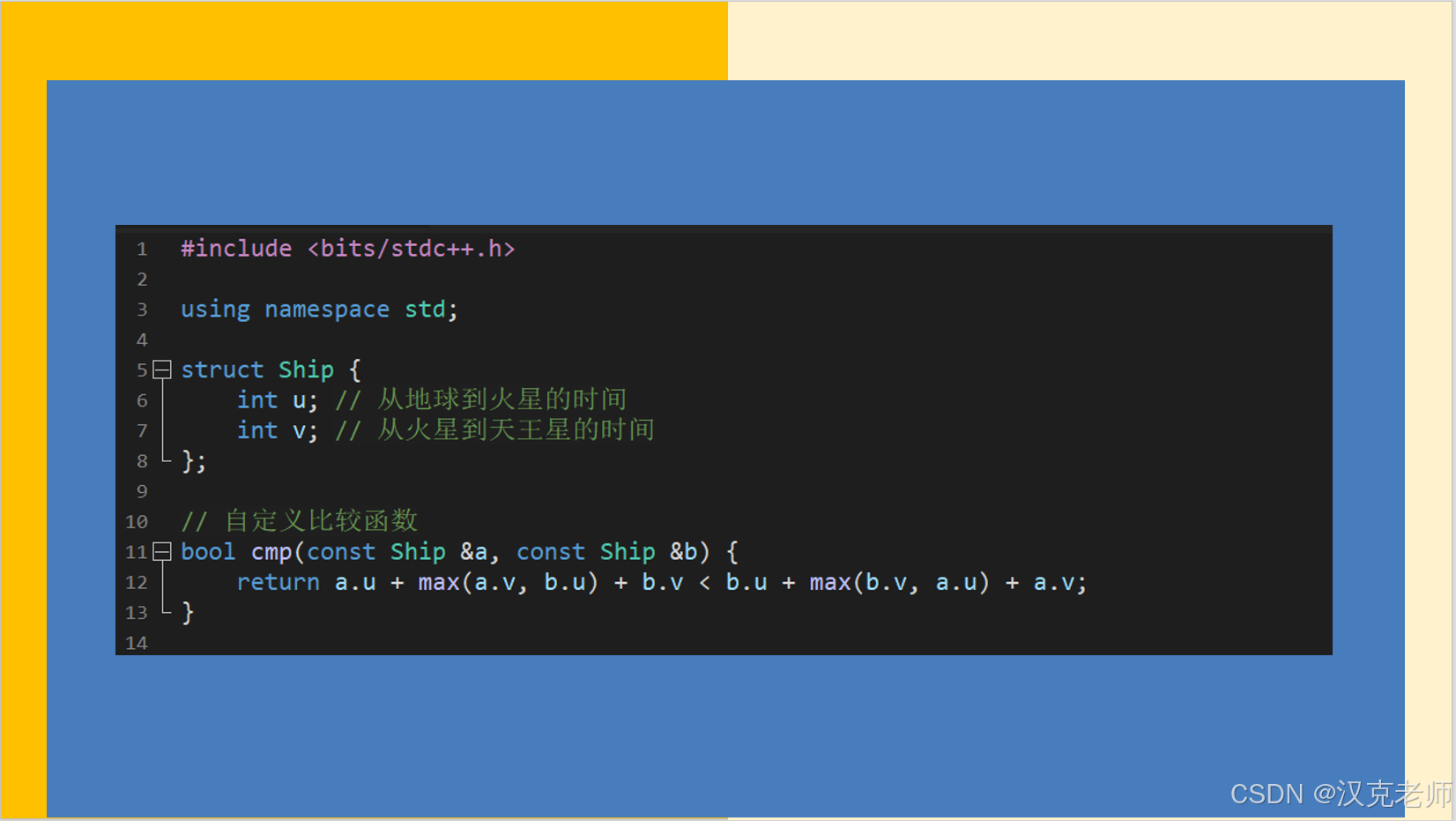
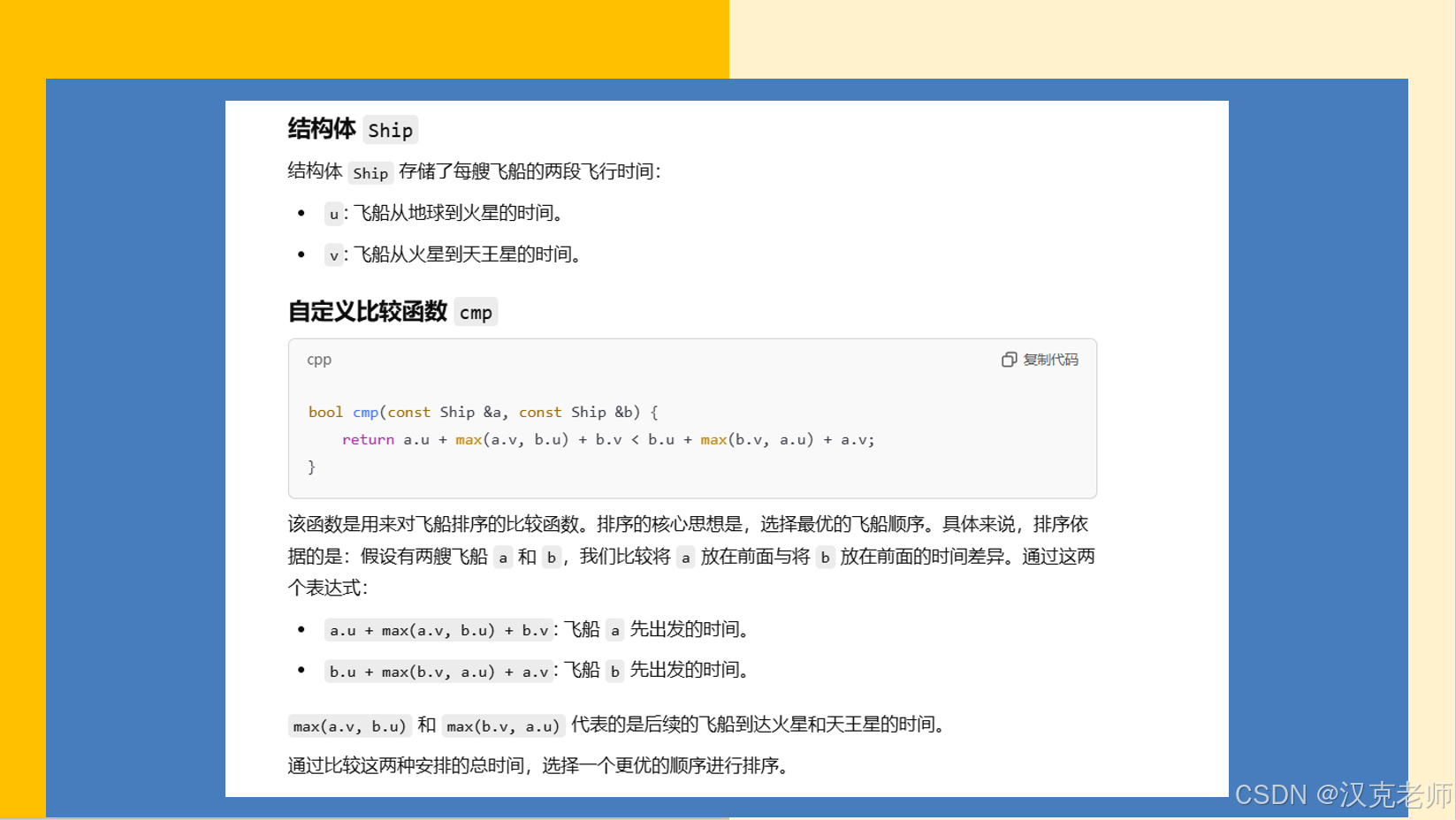
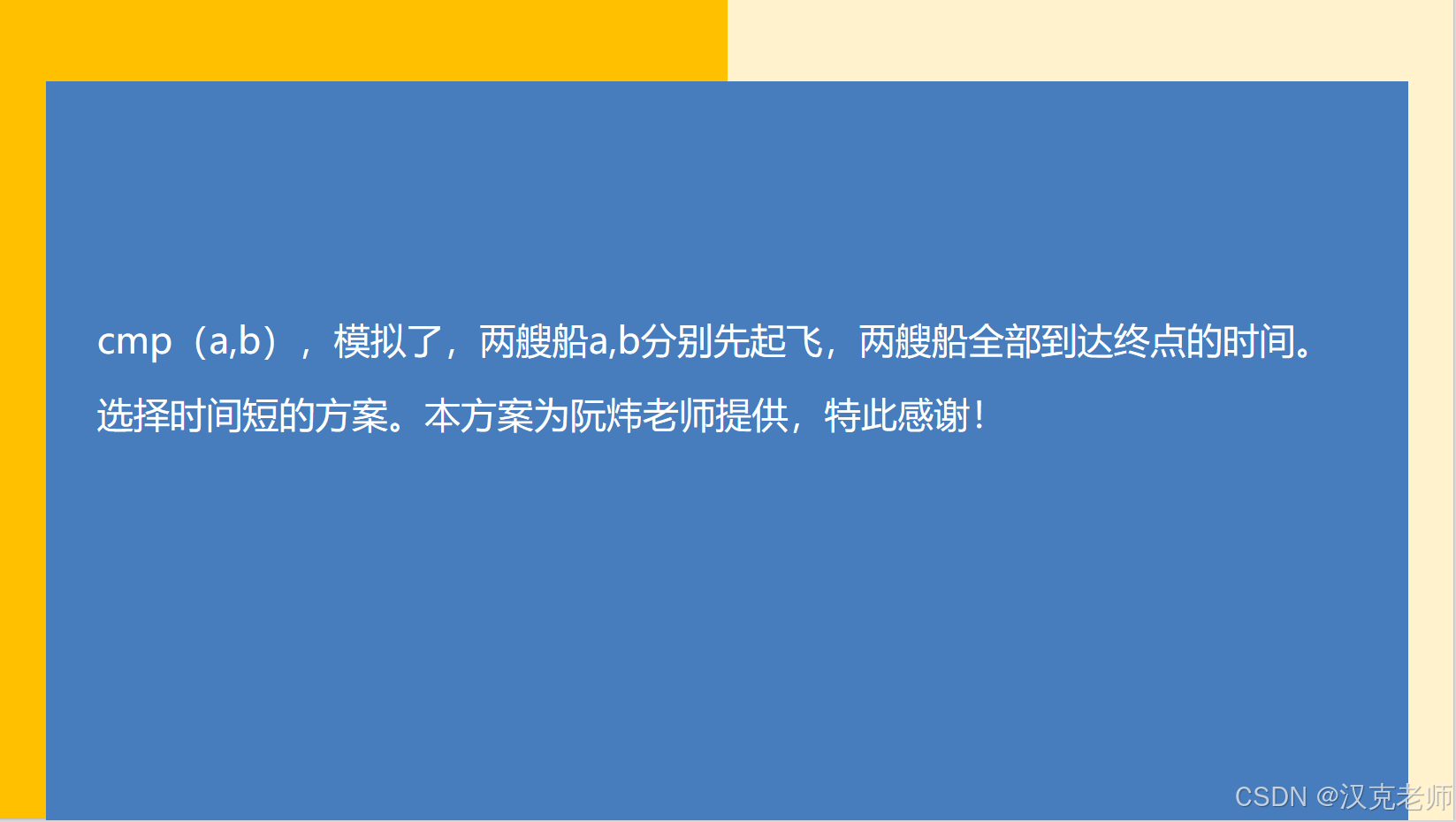
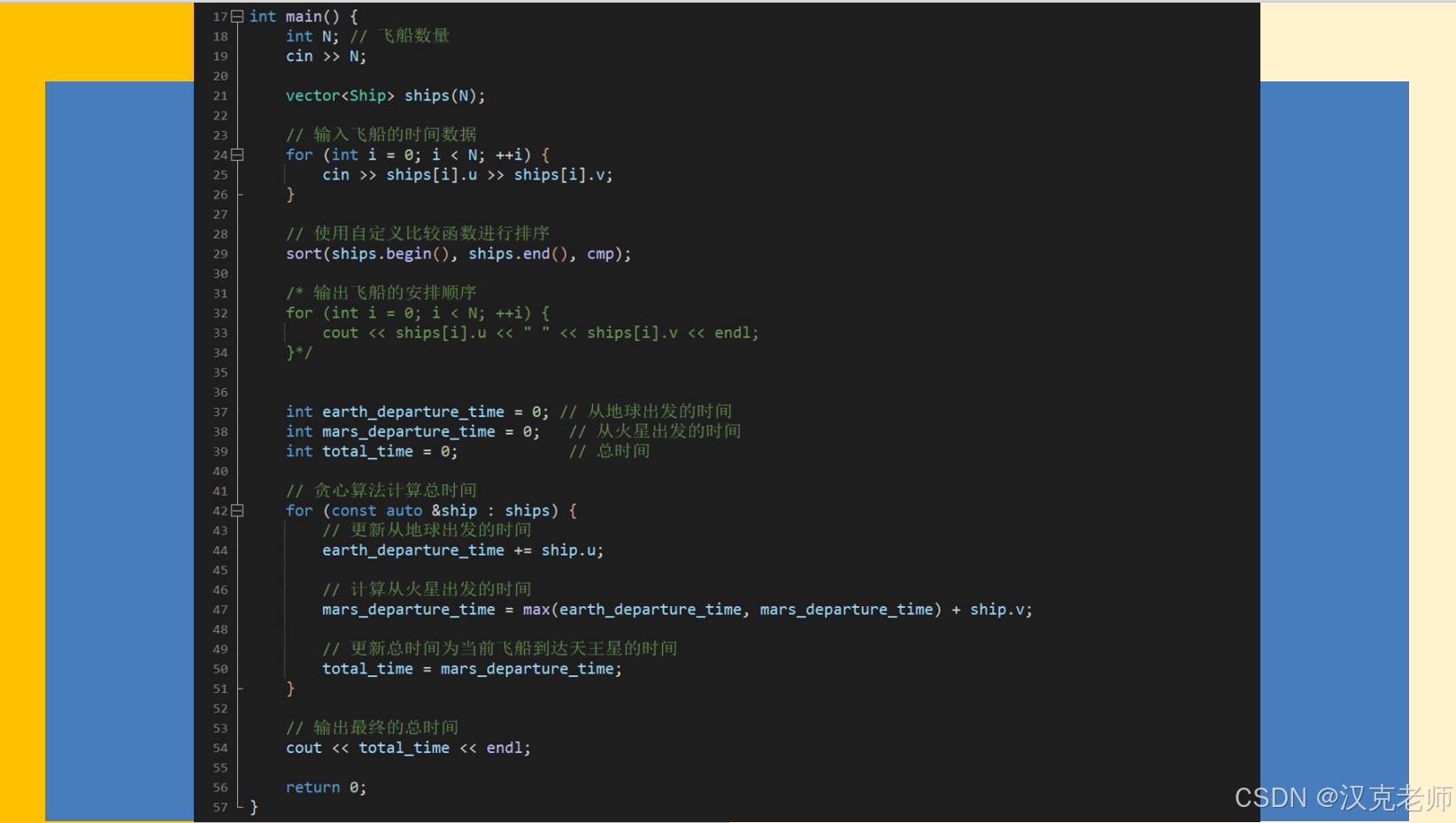
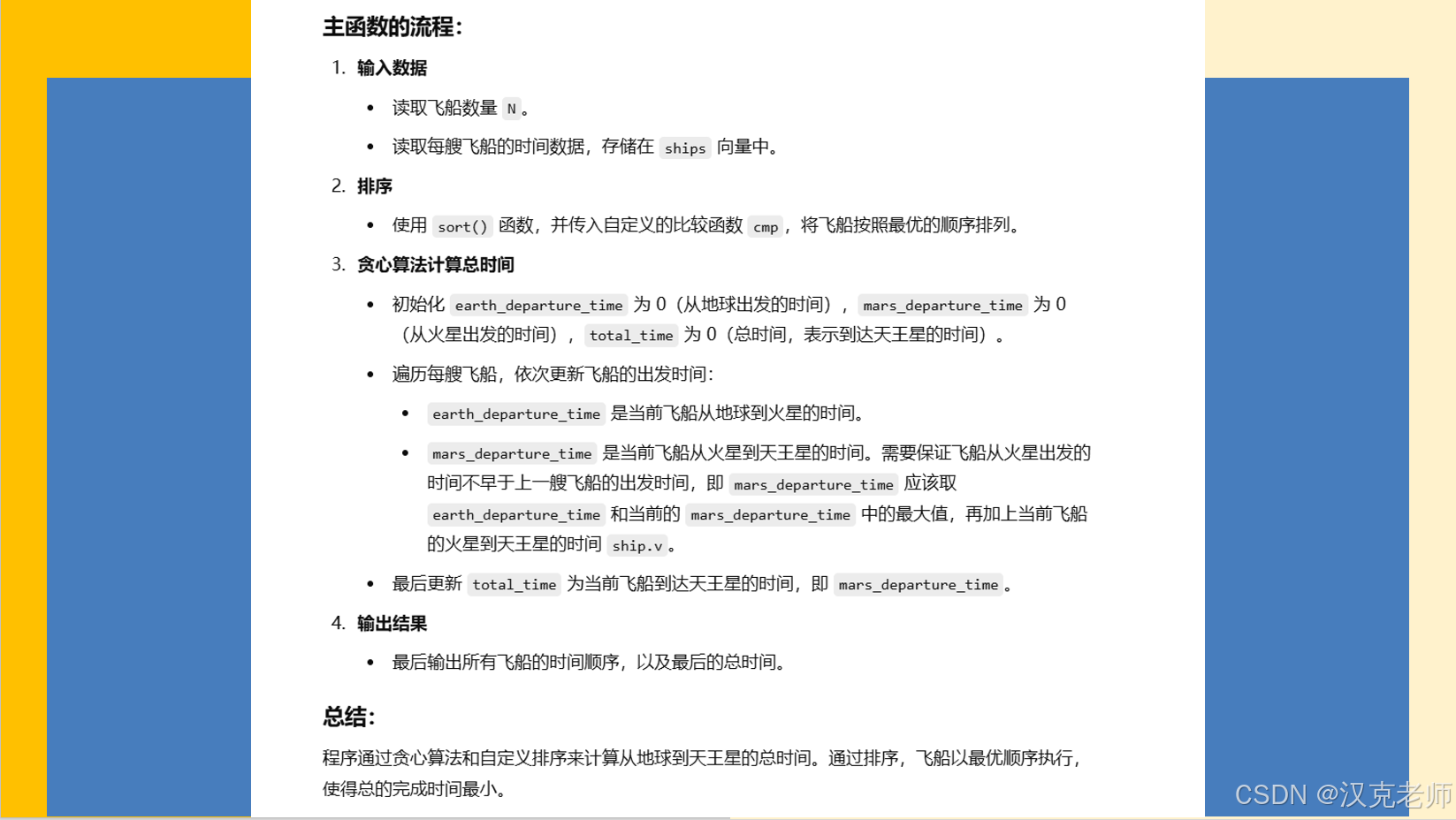
#include <bits/stdc++.h>
using namespace std;
struct Ship {
int u; // 从地球到火星的时间
int v; // 从火星到天王星的时间
};
// 自定义比较函数
bool cmp(const Ship &a, const Ship &b) {
return a.u + max(a.v, b.u) + b.v < b.u + max(b.v, a.u) + a.v;
}
int main() {
// 使用 freopen 进行文件输入输出
//freopen("travel.in", "r", stdin); // 输入文件
//freopen("travel.out", "w", stdout); // 输出文件
int N; // 飞船数量
cin >> N;
vector<Ship> ships(N);
// 输入飞船的时间数据
for (int i = 0; i < N; ++i) {
cin >> ships[i].u >> ships[i].v;
}
// 使用自定义比较函数进行排序
sort(ships.begin(), ships.end(), cmp);
// 输出飞船的排序结果
/*for (int i = 0; i < N; ++i) {
cout << ships[i].u << " " << ships[i].v << endl;
}*/
int earth_departure_time = 0; // 从地球出发的时间
int mars_departure_time = 0; // 从火星出发的时间
int total_time = 0; // 总时间
// 贪心算法计算总时间
for (const auto &ship : ships) {
// 更新从地球出发的时间
earth_departure_time += ship.u;
// 计算从火星出发的时间
mars_departure_time = max(earth_departure_time, mars_departure_time) + ship.v;
// 更新总时间为当前飞船到达天王星的时间
total_time = mars_departure_time;
}
// 输出最终的总时间
cout << total_time << endl;
return 0;
}