效果如下:
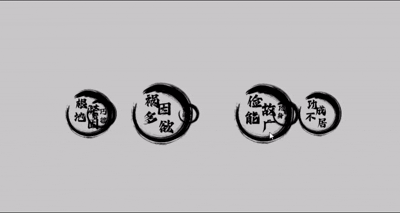
场景挂载:
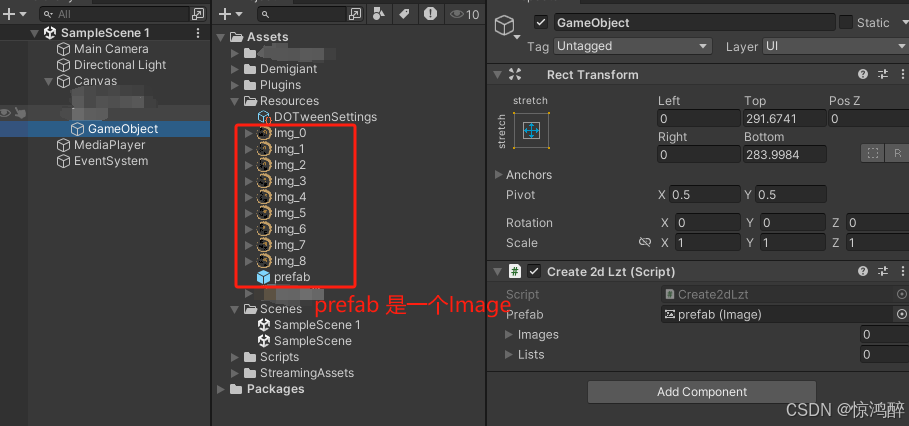
代码部分:
using DG.Tweening;
using System;
using System.Collections;
using System.Collections.Generic;
using System.Drawing.Printing;
using UnityEngine;
using UnityEngine.EventSystems;
using UnityEngine.UI;
public class Create2dLzt : MonoBehaviour, IDragHandler, IEndDragHandler
{
public Image prefab;
float r;
float c;
float ang;
float space = 400;//间距
float n = 7;
public List<Image> Images = new List<Image>();
public List<Transform> lists = new List<Transform>();
float moveAng = 0;
/// <summary>
/// 缩放最大小值
/// </summary>
float max = 3;
float min = 1f;
// Start is called before the first frame update
void Start()
{
c = (space + prefab.rectTransform.rect.width) * n;
r = c / (2 * Mathf.PI);
ang = (2 * Mathf.PI) / n;
Move();
}
private void Move()
{
for (int i = 0; i < n; i++)
{
if (Images.Count <= i)
{
Images.Add(Instantiate(prefab, transform));
Images[i].sprite = Resources.Load<Sprite>("Img_" + i);
lists.Add(Images[i].transform);
Images[i].name = "Img_" + i;
}
float x = Mathf.Sin(ang * i + moveAng) * r;
float z = Mathf.Cos(ang * i + moveAng) * r;
float p = (r + z) / (2 * r);
float scale = (max - min) * p + min;
Images[i].transform.localScale = Vector3.one * scale;
Images[i].rectTransform.anchoredPosition = new Vector2(x, 0);
}
lists.Sort((a, b) =>
{
if (a.localScale.x < b.localScale.x)
{
return -1;
}
else if (a.localScale.x == b.localScale.x)
{
return 0;
}
else
{
return 1;
}
});
for (int i = 0; i < lists.Count; i++)
{
lists[i].SetSiblingIndex(i);
}
}
public void OnDrag(PointerEventData eventData)
{
float dis = eventData.delta.x;
float ang = dis / r;
moveAng += ang;
Move();
}
public void OnEndDrag(PointerEventData eventData)
{
float dis = eventData.delta.x;
float time = Mathf.Abs(dis) / 100;
DOTween.To((a) =>
{
float ang = a / r;
moveAng += ang;
Move();
}, dis, 0, time).OnComplete(() =>
{
Align();
});
}
private void Align()
{
float ang = Mathf.Asin(lists[lists.Count - 1].localPosition.x / r);
float dis = ang * r;
float time = Mathf.Abs(dis) / 100;
DOTween.To((a) =>
{
moveAng = a;
Move();
}, moveAng, moveAng - ang, time);
}
}